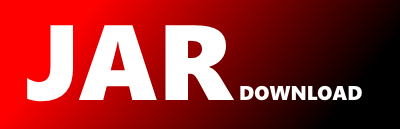
com.sinch.sdk.domains.verification.models.v1.webhooks.VerificationRequestEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Verification | Sinch
*
* OpenAPI document version: 2.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.verification.models.v1.webhooks;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.sinch.sdk.core.utils.EnumDynamic;
import com.sinch.sdk.core.utils.EnumSupportDynamic;
import com.sinch.sdk.domains.verification.models.v1.Identity;
import com.sinch.sdk.domains.verification.models.v1.Price;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Stream;
/** VerificationRequestEvent */
@JsonDeserialize(builder = VerificationRequestEventImpl.Builder.class)
public interface VerificationRequestEvent
extends com.sinch.sdk.domains.verification.models.v1.webhooks.VerificationEvent {
/**
* The ID of the verification request.
*
* @return id
*/
String getId();
/** The type of the event. */
public class EventEnum extends EnumDynamic {
public static final EventEnum VERIFICATION_REQUEST_EVENT =
new EventEnum("VerificationRequestEvent");
private static final EnumSupportDynamic ENUM_SUPPORT =
new EnumSupportDynamic<>(
EventEnum.class, EventEnum::new, Arrays.asList(VERIFICATION_REQUEST_EVENT));
private EventEnum(String value) {
super(value);
}
public static Stream values() {
return ENUM_SUPPORT.values();
}
public static EventEnum from(String value) {
return ENUM_SUPPORT.from(value);
}
public static String valueOf(EventEnum e) {
return ENUM_SUPPORT.valueOf(e);
}
}
/**
* The type of the event.
*
* @return event
*/
EventEnum getEvent();
/**
* Get identity
*
* @return identity
*/
Identity getIdentity();
/**
* Used to pass your own reference in the request for tracking purposes.
*
* @return reference
*/
String getReference();
/**
* Can be used to pass custom data in the request.
*
* @return custom
*/
String getCustom();
/** The verification method. */
public class MethodEnum extends EnumDynamic {
public static final MethodEnum SMS = new MethodEnum("sms");
public static final MethodEnum FLASH_CALL = new MethodEnum("flashcall");
public static final MethodEnum PHONE_CALL = new MethodEnum("callout");
private static final EnumSupportDynamic ENUM_SUPPORT =
new EnumSupportDynamic<>(
MethodEnum.class, MethodEnum::new, Arrays.asList(SMS, FLASH_CALL, PHONE_CALL));
private MethodEnum(String value) {
super(value);
}
public static Stream values() {
return ENUM_SUPPORT.values();
}
public static MethodEnum from(String value) {
return ENUM_SUPPORT.from(value);
}
public static String valueOf(MethodEnum e) {
return ENUM_SUPPORT.valueOf(e);
}
}
/**
* The verification method.
*
* @return method
*/
MethodEnum getMethod();
/**
* Get price
*
* @return price
*/
Price getPrice();
/**
* Allows you to set or override if provided in the API request, the SMS verification content
* language. Only used with the SMS verification method. The content language specified in the API
* request or in the callback can be overridden by carrier provider specific templates, due to
* compliance and legal requirements, such as US
* shortcode requirements (pdf).
*
* @return acceptLanguage
* @deprecated
*/
@Deprecated
List getAcceptLanguage();
/**
* Getting builder
*
* @return New Builder instance
*/
static Builder builder() {
return new VerificationRequestEventImpl.Builder();
}
/** Dedicated Builder */
interface Builder
extends com.sinch.sdk.domains.verification.models.v1.webhooks.VerificationEvent.Builder {
/**
* see getter
*
* @param id see getter
* @return Current builder
* @see #getId
*/
Builder setId(String id);
/**
* see getter
*
* @param event see getter
* @return Current builder
* @see #getEvent
*/
Builder setEvent(EventEnum event);
/**
* see getter
*
* @param identity see getter
* @return Current builder
* @see #getIdentity
*/
Builder setIdentity(Identity identity);
/**
* see getter
*
* @param reference see getter
* @return Current builder
* @see #getReference
*/
Builder setReference(String reference);
/**
* see getter
*
* @param custom see getter
* @return Current builder
* @see #getCustom
*/
Builder setCustom(String custom);
/**
* see getter
*
* @param method see getter
* @return Current builder
* @see #getMethod
*/
Builder setMethod(MethodEnum method);
/**
* see getter
*
* @param price see getter
* @return Current builder
* @see #getPrice
*/
Builder setPrice(Price price);
/**
* see getter
*
* @param acceptLanguage see getter
* @return Current builder
* @see #getAcceptLanguage
*/
@Deprecated
Builder setAcceptLanguage(List acceptLanguage);
/**
* Create instance
*
* @return The instance build with current builder values
*/
VerificationRequestEvent build();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy