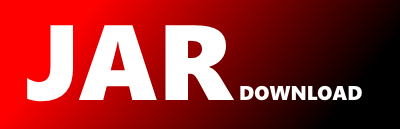
com.sinch.sdk.domains.voice.CallsService Maven / Gradle / Ivy
Show all versions of sinch-sdk-java Show documentation
package com.sinch.sdk.domains.voice;
import com.sinch.sdk.domains.voice.models.CallLegType;
import com.sinch.sdk.domains.voice.models.response.CallInformation;
import com.sinch.sdk.domains.voice.models.svaml.SVAMLControl;
/**
* Using the Calls service, you can manage on-going calls or retrieve information about a call.
*
* @see https://developers.sinch.com/docs/voice/api-reference/voice/tag/Calls
* @since 1.0
*/
public interface CallsService {
/**
* You can retrieve information about an ongoing or completed call using a call ID. You can find
* the call ID of an ongoing call by viewing the response object from a callout request. You can
* find the call ID of a completed call by looking at your call logs in your Sinch Dashboard.
*
* Note: You can only use this method for calls that terminate to PSTN or SIP networks from an
* In-app call.
*
* @param callId The unique identifier of the call. This value is generated by the system
* @return Information about the call
*/
CallInformation get(String callId);
/**
* This method is used to manage ongoing, connected calls. This method uses SVAML in the request
* body to perform various tasks related to the call. For more information about SVAML, see the
* Callback API documentation.
*
*
Note: You can only use this method for calls that terminate to PSTN or SIP networks from an
* In-app call.
*
* @param callId The unique identifier of the call. This value is generated by the system
* @param parameters Tasks to be used related to this call
*/
void update(String callId, SVAMLControl parameters);
/**
* This method is used to manage ongoing, connected calls. This method is only used when using the
* PlayFiles and Say instructions in the request body. This method uses SVAML in the request body
* to perform various tasks related to the call. For more information about SVAML, see the
* Callback API documentation.
*
*
Note: You can only use this method for calls that terminate to PSTN or SIP networks from an
* In-app call.
*
* @param callId The unique identifier of the call. This value is generated by the system
* @param parameters Tasks to be used related to this call
*/
void manageWithCallLeg(String callId, CallLegType callLeg, SVAMLControl parameters);
}