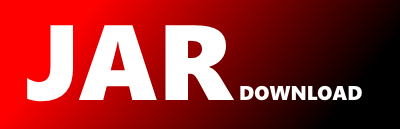
com.sinch.sdk.domains.voice.adapters.api.v1.ApplicationsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Voice API | Sinch
* The Voice API exposes calling- and conference-related functionality in the Sinch Voice Platform.
*
* The version of the OpenAPI document: 1.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.voice.adapters.api.v1;
import com.fasterxml.jackson.core.type.TypeReference;
import com.sinch.sdk.core.exceptions.ApiException;
import com.sinch.sdk.core.exceptions.ApiExceptionBuilder;
import com.sinch.sdk.core.http.AuthManager;
import com.sinch.sdk.core.http.HttpClient;
import com.sinch.sdk.core.http.HttpMapper;
import com.sinch.sdk.core.http.HttpMethod;
import com.sinch.sdk.core.http.HttpRequest;
import com.sinch.sdk.core.http.HttpResponse;
import com.sinch.sdk.core.http.HttpStatus;
import com.sinch.sdk.core.http.URLParameter;
import com.sinch.sdk.core.http.URLPathUtils;
import com.sinch.sdk.core.models.ServerConfiguration;
import com.sinch.sdk.domains.voice.models.dto.v1.CallbacksDto;
import com.sinch.sdk.domains.voice.models.dto.v1.GetNumbersResponseObjDto;
import com.sinch.sdk.domains.voice.models.dto.v1.GetQueryNumberDto;
import com.sinch.sdk.domains.voice.models.dto.v1.UnassignNumbersDto;
import com.sinch.sdk.domains.voice.models.dto.v1.UpdateNumbersDto;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
public class ApplicationsApi {
private static final Logger LOGGER = Logger.getLogger(ApplicationsApi.class.getName());
private HttpClient httpClient;
private ServerConfiguration serverConfiguration;
private Map authManagersByOasSecuritySchemes;
private HttpMapper mapper;
public ApplicationsApi(
HttpClient httpClient,
ServerConfiguration serverConfiguration,
Map authManagersByOasSecuritySchemes,
HttpMapper mapper) {
this.httpClient = httpClient;
this.serverConfiguration = serverConfiguration;
this.authManagersByOasSecuritySchemes = authManagersByOasSecuritySchemes;
this.mapper = mapper;
}
/**
* Query number Returns information about the requested number.
*
* @param number The phone number you want to query. (required)
* @return GetQueryNumberDto
* @throws ApiException if fails to make API call
*/
public GetQueryNumberDto callingQueryNumber(String number) throws ApiException {
LOGGER.finest("[callingQueryNumber]" + " " + "number: " + number);
HttpRequest httpRequest = callingQueryNumberRequestBuilder(number);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType =
new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest callingQueryNumberRequestBuilder(String number) throws ApiException {
// verify the required parameter 'number' is set
if (number == null) {
throw new ApiException(
400, "Missing the required parameter 'number' when calling callingQueryNumber");
}
String localVarPath =
"/v1/calling/query/number/{number}"
.replaceAll(
"\\{" + "number" + "\\}", URLPathUtils.encodePathSegment(number.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("Basic", "Signed");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Get Callback URLs Returns any callback URLs configured for the specified application.
*
* @param applicationkey The unique identifying key of the application. (required)
* @return CallbacksDto
* @throws ApiException if fails to make API call
*/
public CallbacksDto configurationGetCallbackURLs(String applicationkey) throws ApiException {
LOGGER.finest("[configurationGetCallbackURLs]" + " " + "applicationkey: " + applicationkey);
HttpRequest httpRequest = configurationGetCallbackURLsRequestBuilder(applicationkey);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest configurationGetCallbackURLsRequestBuilder(String applicationkey)
throws ApiException {
// verify the required parameter 'applicationkey' is set
if (applicationkey == null) {
throw new ApiException(
400,
"Missing the required parameter 'applicationkey' when calling"
+ " configurationGetCallbackURLs");
}
String localVarPath =
"/v1/configuration/callbacks/applications/{applicationkey}"
.replaceAll(
"\\{" + "applicationkey" + "\\}",
URLPathUtils.encodePathSegment(applicationkey.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("Basic", "Signed");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Get Numbers Get information about your numbers. It returns a list of numbers that you own, as
* well as their capability (voice or SMS). For the ones that are assigned to an app, it returns
* the application key of the app.
*
* @return GetNumbersResponseObjDto
* @throws ApiException if fails to make API call
*/
public GetNumbersResponseObjDto configurationGetNumbers() throws ApiException {
LOGGER.finest("[configurationGetNumbers]");
HttpRequest httpRequest = configurationGetNumbersRequestBuilder();
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType =
new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest configurationGetNumbersRequestBuilder() throws ApiException {
String localVarPath = "/v1/configuration/numbers";
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("Basic", "Signed");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Un-assign number Un-assign a number from an application.
*
* @param unassignNumbersDto (optional)
* @throws ApiException if fails to make API call
*/
public void configurationUnassignNumber(UnassignNumbersDto unassignNumbersDto)
throws ApiException {
LOGGER.finest(
"[configurationUnassignNumber]" + " " + "unassignNumbersDto: " + unassignNumbersDto);
HttpRequest httpRequest = configurationUnassignNumberRequestBuilder(unassignNumbersDto);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
return;
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest configurationUnassignNumberRequestBuilder(
UnassignNumbersDto unassignNumbersDto) throws ApiException {
String localVarPath = "/v1/configuration/numbers";
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList();
final Collection localVarContentTypes = Arrays.asList("application/json");
final Collection localVarAuthNames = Arrays.asList("Basic", "Signed");
final String serializedBody = mapper.serialize(localVarContentTypes, unassignNumbersDto);
return new HttpRequest(
localVarPath,
HttpMethod.DELETE,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Update Callbacks Update the configured callback URLs for the specified application.
*
* @param applicationkey The unique identifying key of the application. (required)
* @param callbacksDto (optional)
* @throws ApiException if fails to make API call
*/
public void configurationUpdateCallbackURLs(String applicationkey, CallbacksDto callbacksDto)
throws ApiException {
LOGGER.finest(
"[configurationUpdateCallbackURLs]"
+ " "
+ "applicationkey: "
+ applicationkey
+ ", "
+ "callbacksDto: "
+ callbacksDto);
HttpRequest httpRequest =
configurationUpdateCallbackURLsRequestBuilder(applicationkey, callbacksDto);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
return;
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest configurationUpdateCallbackURLsRequestBuilder(
String applicationkey, CallbacksDto callbacksDto) throws ApiException {
// verify the required parameter 'applicationkey' is set
if (applicationkey == null) {
throw new ApiException(
400,
"Missing the required parameter 'applicationkey' when calling"
+ " configurationUpdateCallbackURLs");
}
String localVarPath =
"/v1/configuration/callbacks/applications/{applicationkey}"
.replaceAll(
"\\{" + "applicationkey" + "\\}",
URLPathUtils.encodePathSegment(applicationkey.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList();
final Collection localVarContentTypes = Arrays.asList("application/json");
final Collection localVarAuthNames = Arrays.asList("Basic", "Signed");
final String serializedBody = mapper.serialize(localVarContentTypes, callbacksDto);
return new HttpRequest(
localVarPath,
HttpMethod.POST,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Update Numbers Assign a number or a list of numbers to an application.
*
* @param updateNumbersDto (optional)
* @throws ApiException if fails to make API call
*/
public void configurationUpdateNumbers(UpdateNumbersDto updateNumbersDto) throws ApiException {
LOGGER.finest("[configurationUpdateNumbers]" + " " + "updateNumbersDto: " + updateNumbersDto);
HttpRequest httpRequest = configurationUpdateNumbersRequestBuilder(updateNumbersDto);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
return;
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest configurationUpdateNumbersRequestBuilder(UpdateNumbersDto updateNumbersDto)
throws ApiException {
String localVarPath = "/v1/configuration/numbers";
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList();
final Collection localVarContentTypes = Arrays.asList("application/json");
final Collection localVarAuthNames = Arrays.asList("Basic", "Signed");
final String serializedBody = mapper.serialize(localVarContentTypes, updateNumbersDto);
return new HttpRequest(
localVarPath,
HttpMethod.POST,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy