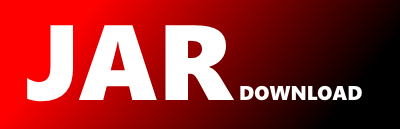
com.sinch.sdk.domains.voice.adapters.api.v1.CalloutsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Voice API | Sinch
* The Voice API exposes calling- and conference-related functionality in the Sinch Voice Platform.
*
* The version of the OpenAPI document: 1.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.voice.adapters.api.v1;
import com.fasterxml.jackson.core.type.TypeReference;
import com.sinch.sdk.core.exceptions.ApiException;
import com.sinch.sdk.core.exceptions.ApiExceptionBuilder;
import com.sinch.sdk.core.http.AuthManager;
import com.sinch.sdk.core.http.HttpClient;
import com.sinch.sdk.core.http.HttpMapper;
import com.sinch.sdk.core.http.HttpMethod;
import com.sinch.sdk.core.http.HttpRequest;
import com.sinch.sdk.core.http.HttpResponse;
import com.sinch.sdk.core.http.HttpStatus;
import com.sinch.sdk.core.http.URLParameter;
import com.sinch.sdk.core.models.ServerConfiguration;
import com.sinch.sdk.domains.voice.models.dto.v1.CalloutRequestDto;
import com.sinch.sdk.domains.voice.models.dto.v1.GetCalloutResponseObjDto;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
public class CalloutsApi {
private static final Logger LOGGER = Logger.getLogger(CalloutsApi.class.getName());
private HttpClient httpClient;
private ServerConfiguration serverConfiguration;
private Map authManagersByOasSecuritySchemes;
private HttpMapper mapper;
public CalloutsApi(
HttpClient httpClient,
ServerConfiguration serverConfiguration,
Map authManagersByOasSecuritySchemes,
HttpMapper mapper) {
this.httpClient = httpClient;
this.serverConfiguration = serverConfiguration;
this.authManagersByOasSecuritySchemes = authManagersByOasSecuritySchemes;
this.mapper = mapper;
}
/**
* Callout Request Makes a call out to a phone number. The types of callouts currently supported
* are conference callouts, text-to-speech callouts, and custom callouts. The custom callout is
* the most flexible, but text-to-speech and conference callouts are more convenient.
*
* @param calloutRequestDto (optional)
* @return GetCalloutResponseObjDto
* @throws ApiException if fails to make API call
*/
public GetCalloutResponseObjDto callouts(CalloutRequestDto calloutRequestDto)
throws ApiException {
LOGGER.finest("[callouts]" + " " + "calloutRequestDto: " + calloutRequestDto);
HttpRequest httpRequest = calloutsRequestBuilder(calloutRequestDto);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType =
new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest calloutsRequestBuilder(CalloutRequestDto calloutRequestDto)
throws ApiException {
String localVarPath = "/calling/v1/callouts";
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList("application/json");
final Collection localVarAuthNames = Arrays.asList("Basic", "Signed");
final String serializedBody = mapper.serialize(localVarContentTypes, calloutRequestDto);
return new HttpRequest(
localVarPath,
HttpMethod.POST,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy