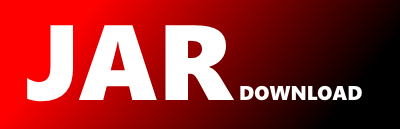
com.sinch.sdk.domains.voice.models.dto.v1.DiceRequestDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Voice API | Sinch
* The Voice API exposes calling- and conference-related functionality in the Sinch Voice Platform.
*
* The version of the OpenAPI document: 1.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.voice.models.dto.v1;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonValue;
import com.sinch.sdk.core.utils.databind.JSONNavigator;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/** The request body of a Disconnected Call Event. */
@JsonPropertyOrder({
DiceRequestDto.JSON_PROPERTY_EVENT,
DiceRequestDto.JSON_PROPERTY_TIMESTAMP,
DiceRequestDto.JSON_PROPERTY_CUSTOM,
DiceRequestDto.JSON_PROPERTY_APPLICATION_KEY,
DiceRequestDto.JSON_PROPERTY_REASON,
DiceRequestDto.JSON_PROPERTY_RESULT,
DiceRequestDto.JSON_PROPERTY_DEBIT,
DiceRequestDto.JSON_PROPERTY_USER_RATE,
DiceRequestDto.JSON_PROPERTY_TO,
DiceRequestDto.JSON_PROPERTY_DURATION,
DiceRequestDto.JSON_PROPERTY_FROM,
DiceRequestDto.JSON_PROPERTY_CALL_HEADERS
})
@JsonFilter("uninitializedFilter")
@JsonInclude(value = JsonInclude.Include.CUSTOM)
/*@JsonIgnoreProperties(
value = "event", // ignore manually set event, it will be automatically generated by Jackson during serialization
allowSetters = true // allows the event to be set during deserialization
)*/
@JsonTypeInfo(
use = JsonTypeInfo.Id.NONE,
include = JsonTypeInfo.As.EXISTING_PROPERTY,
property = "event",
visible = true)
public class DiceRequestDto extends WebhooksEventRequestDto {
private static final long serialVersionUID = 1L;
/** Must have the value `dice`. */
public enum EventEnum {
DICE("dice"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
EventEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static EventEnum fromValue(String value) {
for (EventEnum b : EventEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_EVENT = "event";
private String event;
private boolean eventDefined = false;
public static final String JSON_PROPERTY_TIMESTAMP = "timestamp";
private OffsetDateTime timestamp;
private boolean timestampDefined = false;
public static final String JSON_PROPERTY_CUSTOM = "custom";
private String custom;
private boolean customDefined = false;
public static final String JSON_PROPERTY_APPLICATION_KEY = "applicationKey";
private String applicationKey;
private boolean applicationKeyDefined = false;
/** The reason the call was disconnected. */
public enum ReasonEnum {
N_A("N/A"),
TIMEOUT("TIMEOUT"),
CALLERHANGUP("CALLERHANGUP"),
CALLEEHANGUP("CALLEEHANGUP"),
BLOCKED("BLOCKED"),
MANAGERHANGUP("MANAGERHANGUP"),
NOCREDITPARTNER("NOCREDITPARTNER"),
GENERALERROR("GENERALERROR"),
CANCEL("CANCEL"),
USERNOTFOUND("USERNOTFOUND"),
CALLBACKERROR("CALLBACKERROR"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ReasonEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ReasonEnum fromValue(String value) {
for (ReasonEnum b : ReasonEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_REASON = "reason";
private String reason;
private boolean reasonDefined = false;
public static final String JSON_PROPERTY_RESULT = "result";
private CallResultDto result;
private boolean resultDefined = false;
public static final String JSON_PROPERTY_DEBIT = "debit";
private PriceDto debit;
private boolean debitDefined = false;
public static final String JSON_PROPERTY_USER_RATE = "userRate";
private PriceDto userRate;
private boolean userRateDefined = false;
public static final String JSON_PROPERTY_TO = "to";
private DestinationDto to;
private boolean toDefined = false;
public static final String JSON_PROPERTY_DURATION = "duration";
private Integer duration;
private boolean durationDefined = false;
public static final String JSON_PROPERTY_FROM = "from";
private String from;
private boolean fromDefined = false;
public static final String JSON_PROPERTY_CALL_HEADERS = "callHeaders";
private List callHeaders;
private boolean callHeadersDefined = false;
public DiceRequestDto() {}
public DiceRequestDto event(String event) {
this.event = event;
this.eventDefined = true;
return this;
}
/**
* Must have the value `dice`.
*
* @return event
*/
@JsonProperty(JSON_PROPERTY_EVENT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getEvent() {
return event;
}
@JsonIgnore
public boolean getEventDefined() {
return eventDefined;
}
@JsonProperty(JSON_PROPERTY_EVENT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setEvent(String event) {
this.event = event;
this.eventDefined = true;
}
public DiceRequestDto timestamp(OffsetDateTime timestamp) {
this.timestamp = timestamp;
this.timestampDefined = true;
return this;
}
/**
* The timestamp in UTC format.
*
* @return timestamp
*/
@JsonProperty(JSON_PROPERTY_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getTimestamp() {
return timestamp;
}
@JsonIgnore
public boolean getTimestampDefined() {
return timestampDefined;
}
@JsonProperty(JSON_PROPERTY_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTimestamp(OffsetDateTime timestamp) {
this.timestamp = timestamp;
this.timestampDefined = true;
}
public DiceRequestDto custom(String custom) {
this.custom = custom;
this.customDefined = true;
return this;
}
/**
* A string that can be used to pass custom information related to the call.
*
* @return custom
*/
@JsonProperty(JSON_PROPERTY_CUSTOM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCustom() {
return custom;
}
@JsonIgnore
public boolean getCustomDefined() {
return customDefined;
}
@JsonProperty(JSON_PROPERTY_CUSTOM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCustom(String custom) {
this.custom = custom;
this.customDefined = true;
}
public DiceRequestDto applicationKey(String applicationKey) {
this.applicationKey = applicationKey;
this.applicationKeyDefined = true;
return this;
}
/**
* The unique application key. You can find it in the Sinch
* [dashboard](https://dashboard.sinch.com/voice/apps).
*
* @return applicationKey
*/
@JsonProperty(JSON_PROPERTY_APPLICATION_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getApplicationKey() {
return applicationKey;
}
@JsonIgnore
public boolean getApplicationKeyDefined() {
return applicationKeyDefined;
}
@JsonProperty(JSON_PROPERTY_APPLICATION_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setApplicationKey(String applicationKey) {
this.applicationKey = applicationKey;
this.applicationKeyDefined = true;
}
public DiceRequestDto reason(String reason) {
this.reason = reason;
this.reasonDefined = true;
return this;
}
/**
* The reason the call was disconnected.
*
* @return reason
*/
@JsonProperty(JSON_PROPERTY_REASON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getReason() {
return reason;
}
@JsonIgnore
public boolean getReasonDefined() {
return reasonDefined;
}
@JsonProperty(JSON_PROPERTY_REASON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReason(String reason) {
this.reason = reason;
this.reasonDefined = true;
}
public DiceRequestDto result(CallResultDto result) {
this.result = result;
this.resultDefined = true;
return this;
}
/**
* Get result
*
* @return result
*/
@JsonProperty(JSON_PROPERTY_RESULT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CallResultDto getResult() {
return result;
}
@JsonIgnore
public boolean getResultDefined() {
return resultDefined;
}
@JsonProperty(JSON_PROPERTY_RESULT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setResult(CallResultDto result) {
this.result = result;
this.resultDefined = true;
}
public DiceRequestDto debit(PriceDto debit) {
this.debit = debit;
this.debitDefined = true;
return this;
}
/**
* Get debit
*
* @return debit
*/
@JsonProperty(JSON_PROPERTY_DEBIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PriceDto getDebit() {
return debit;
}
@JsonIgnore
public boolean getDebitDefined() {
return debitDefined;
}
@JsonProperty(JSON_PROPERTY_DEBIT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDebit(PriceDto debit) {
this.debit = debit;
this.debitDefined = true;
}
public DiceRequestDto userRate(PriceDto userRate) {
this.userRate = userRate;
this.userRateDefined = true;
return this;
}
/**
* Get userRate
*
* @return userRate
*/
@JsonProperty(JSON_PROPERTY_USER_RATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PriceDto getUserRate() {
return userRate;
}
@JsonIgnore
public boolean getUserRateDefined() {
return userRateDefined;
}
@JsonProperty(JSON_PROPERTY_USER_RATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUserRate(PriceDto userRate) {
this.userRate = userRate;
this.userRateDefined = true;
}
public DiceRequestDto to(DestinationDto to) {
this.to = to;
this.toDefined = true;
return this;
}
/**
* Get to
*
* @return to
*/
@JsonProperty(JSON_PROPERTY_TO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DestinationDto getTo() {
return to;
}
@JsonIgnore
public boolean getToDefined() {
return toDefined;
}
@JsonProperty(JSON_PROPERTY_TO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTo(DestinationDto to) {
this.to = to;
this.toDefined = true;
}
public DiceRequestDto duration(Integer duration) {
this.duration = duration;
this.durationDefined = true;
return this;
}
/**
* The duration of the call in seconds.
*
* @return duration
*/
@JsonProperty(JSON_PROPERTY_DURATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getDuration() {
return duration;
}
@JsonIgnore
public boolean getDurationDefined() {
return durationDefined;
}
@JsonProperty(JSON_PROPERTY_DURATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDuration(Integer duration) {
this.duration = duration;
this.durationDefined = true;
}
public DiceRequestDto from(String from) {
this.from = from;
this.fromDefined = true;
return this;
}
/**
* Information about the initiator of the call.
*
* @return from
*/
@JsonProperty(JSON_PROPERTY_FROM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getFrom() {
return from;
}
@JsonIgnore
public boolean getFromDefined() {
return fromDefined;
}
@JsonProperty(JSON_PROPERTY_FROM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFrom(String from) {
this.from = from;
this.fromDefined = true;
}
public DiceRequestDto callHeaders(List callHeaders) {
this.callHeaders = callHeaders;
this.callHeadersDefined = true;
return this;
}
public DiceRequestDto addCallHeadersItem(CallHeaderDto callHeadersItem) {
if (this.callHeaders == null) {
this.callHeaders = new ArrayList<>();
}
this.callHeadersDefined = true;
this.callHeaders.add(callHeadersItem);
return this;
}
/**
* If the call was initiated by a Sinch SDK client, call headers are the headers specified by the
* *caller* client. Read more about call headers [here](../../../call-headers/).
*
* @return callHeaders
*/
@JsonProperty(JSON_PROPERTY_CALL_HEADERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCallHeaders() {
return callHeaders;
}
@JsonIgnore
public boolean getCallHeadersDefined() {
return callHeadersDefined;
}
@JsonProperty(JSON_PROPERTY_CALL_HEADERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCallHeaders(List callHeaders) {
this.callHeaders = callHeaders;
this.callHeadersDefined = true;
}
@Override
public DiceRequestDto callid(String callid) {
this.setCallid(callid);
return this;
}
@Override
public DiceRequestDto version(Integer version) {
this.setVersion(version);
return this;
}
/** Return true if this diceRequest object is equal to o. */
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DiceRequestDto diceRequest = (DiceRequestDto) o;
return Objects.equals(this.event, diceRequest.event)
&& Objects.equals(this.timestamp, diceRequest.timestamp)
&& Objects.equals(this.custom, diceRequest.custom)
&& Objects.equals(this.applicationKey, diceRequest.applicationKey)
&& Objects.equals(this.reason, diceRequest.reason)
&& Objects.equals(this.result, diceRequest.result)
&& Objects.equals(this.debit, diceRequest.debit)
&& Objects.equals(this.userRate, diceRequest.userRate)
&& Objects.equals(this.to, diceRequest.to)
&& Objects.equals(this.duration, diceRequest.duration)
&& Objects.equals(this.from, diceRequest.from)
&& Objects.equals(this.callHeaders, diceRequest.callHeaders)
&& super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(
event,
timestamp,
custom,
applicationKey,
reason,
result,
debit,
userRate,
to,
duration,
from,
callHeaders,
super.hashCode());
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DiceRequestDto {\n");
sb.append(" ").append(toIndentedString(super.toString())).append("\n");
sb.append(" event: ").append(toIndentedString(event)).append("\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append(" custom: ").append(toIndentedString(custom)).append("\n");
sb.append(" applicationKey: ").append(toIndentedString(applicationKey)).append("\n");
sb.append(" reason: ").append(toIndentedString(reason)).append("\n");
sb.append(" result: ").append(toIndentedString(result)).append("\n");
sb.append(" debit: ").append(toIndentedString(debit)).append("\n");
sb.append(" userRate: ").append(toIndentedString(userRate)).append("\n");
sb.append(" to: ").append(toIndentedString(to)).append("\n");
sb.append(" duration: ").append(toIndentedString(duration)).append("\n");
sb.append(" from: ").append(toIndentedString(from)).append("\n");
sb.append(" callHeaders: ").append(toIndentedString(callHeaders)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
static {
// Initialize and register the discriminator mappings.
Map> mappings = new HashMap>();
mappings.put("diceRequest", DiceRequestDto.class);
JSONNavigator.registerDiscriminator(DiceRequestDto.class, "event", mappings);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy