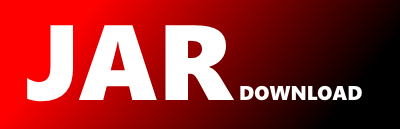
com.sinch.sdk.domains.voice.models.dto.v1.MenuDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Voice API | Sinch
* The Voice API exposes calling- and conference-related functionality in the Sinch Voice Platform.
*
* The version of the OpenAPI document: 1.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.voice.models.dto.v1;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** An IVR menu that contains an audio prompt as well as configured options. */
@JsonPropertyOrder({
MenuDto.JSON_PROPERTY_ID,
MenuDto.JSON_PROPERTY_MAIN_PROMPT,
MenuDto.JSON_PROPERTY_REPEAT_PROMPT,
MenuDto.JSON_PROPERTY_REPEATS,
MenuDto.JSON_PROPERTY_MAX_DIGITS,
MenuDto.JSON_PROPERTY_TIMEOUT_MILLS,
MenuDto.JSON_PROPERTY_MAX_TIMEOUT_MILLS,
MenuDto.JSON_PROPERTY_OPTIONS
})
@JsonFilter("uninitializedFilter")
@JsonInclude(value = JsonInclude.Include.CUSTOM)
public class MenuDto {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ID = "id";
private String id;
private boolean idDefined = false;
public static final String JSON_PROPERTY_MAIN_PROMPT = "mainPrompt";
private String mainPrompt;
private boolean mainPromptDefined = false;
public static final String JSON_PROPERTY_REPEAT_PROMPT = "repeatPrompt";
private String repeatPrompt;
private boolean repeatPromptDefined = false;
public static final String JSON_PROPERTY_REPEATS = "repeats";
private Integer repeats;
private boolean repeatsDefined = false;
public static final String JSON_PROPERTY_MAX_DIGITS = "maxDigits";
private Integer maxDigits;
private boolean maxDigitsDefined = false;
public static final String JSON_PROPERTY_TIMEOUT_MILLS = "timeoutMills";
private Integer timeoutMills;
private boolean timeoutMillsDefined = false;
public static final String JSON_PROPERTY_MAX_TIMEOUT_MILLS = "maxTimeoutMills";
private Integer maxTimeoutMills;
private boolean maxTimeoutMillsDefined = false;
public static final String JSON_PROPERTY_OPTIONS = "options";
private List options;
private boolean optionsDefined = false;
public MenuDto() {}
public MenuDto id(String id) {
this.id = id;
this.idDefined = true;
return this;
}
/**
* The identifier of a menu. One menu must have the ID value of `main`.
*
* @return id
*/
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getId() {
return id;
}
@JsonIgnore
public boolean getIdDefined() {
return idDefined;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setId(String id) {
this.id = id;
this.idDefined = true;
}
public MenuDto mainPrompt(String mainPrompt) {
this.mainPrompt = mainPrompt;
this.mainPromptDefined = true;
return this;
}
/**
* The main voice prompt that the user hears when the menu starts the first time. You can use
* text-to-speech using the `#tts[]` element, SSML commands using the
* `#ssml[]` element, pre-recorded messages, or URL references to external media
* resources. You can use multiple prompts by separating each prompt with a semi-colon
* (`;`). If multiple prompts are used, they will be played in the order they are
* specified, without any pauses between playback. For external media resources, you can use an
* `#href[...]` or directly specify the full URL. Check the [Supported audio
* formats](/docs/voice/api-reference/supported-audio-formats) section for more information.
*
* @return mainPrompt
*/
@JsonProperty(JSON_PROPERTY_MAIN_PROMPT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMainPrompt() {
return mainPrompt;
}
@JsonIgnore
public boolean getMainPromptDefined() {
return mainPromptDefined;
}
@JsonProperty(JSON_PROPERTY_MAIN_PROMPT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMainPrompt(String mainPrompt) {
this.mainPrompt = mainPrompt;
this.mainPromptDefined = true;
}
public MenuDto repeatPrompt(String repeatPrompt) {
this.repeatPrompt = repeatPrompt;
this.repeatPromptDefined = true;
return this;
}
/**
* The prompt that will be played if valid or expected DTMF digits are not entered. You can use
* text-to-speech using the `#tts[]` element, SSML commands using the
* `#ssml[]` element, pre-recorded messages, or URL references to external media
* resources. You can use multiple prompts by separating each prompt with a semi-colon
* (`;`). If multiple prompts are used, they will be played in the order they are
* specified, without any pauses between playback. For external media resources, you can use an
* `#href[...]` or directly specify the full URL. Check the [Supported audio
* formats](/docs/voice/api-reference/supported-audio-formats) section for more information.
*
* @return repeatPrompt
*/
@JsonProperty(JSON_PROPERTY_REPEAT_PROMPT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRepeatPrompt() {
return repeatPrompt;
}
@JsonIgnore
public boolean getRepeatPromptDefined() {
return repeatPromptDefined;
}
@JsonProperty(JSON_PROPERTY_REPEAT_PROMPT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRepeatPrompt(String repeatPrompt) {
this.repeatPrompt = repeatPrompt;
this.repeatPromptDefined = true;
}
public MenuDto repeats(Integer repeats) {
this.repeats = repeats;
this.repeatsDefined = true;
return this;
}
/**
* The number of times that the `repeatPrompt` is played.
*
* @return repeats
*/
@JsonProperty(JSON_PROPERTY_REPEATS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getRepeats() {
return repeats;
}
@JsonIgnore
public boolean getRepeatsDefined() {
return repeatsDefined;
}
@JsonProperty(JSON_PROPERTY_REPEATS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRepeats(Integer repeats) {
this.repeats = repeats;
this.repeatsDefined = true;
}
public MenuDto maxDigits(Integer maxDigits) {
this.maxDigits = maxDigits;
this.maxDigitsDefined = true;
return this;
}
/**
* The maximum number of digits expected for a user to enter. Once these digits are collected, a
* [Prompt Input Event (PIE)](../../voice/tag/Callbacks/#tag/Callbacks/operation/pie) is triggered
* containing these digits.
*
* @return maxDigits
*/
@JsonProperty(JSON_PROPERTY_MAX_DIGITS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getMaxDigits() {
return maxDigits;
}
@JsonIgnore
public boolean getMaxDigitsDefined() {
return maxDigitsDefined;
}
@JsonProperty(JSON_PROPERTY_MAX_DIGITS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMaxDigits(Integer maxDigits) {
this.maxDigits = maxDigits;
this.maxDigitsDefined = true;
}
public MenuDto timeoutMills(Integer timeoutMills) {
this.timeoutMills = timeoutMills;
this.timeoutMillsDefined = true;
return this;
}
/**
* Determines silence for the purposes of collecting a DTMF or voice response in milliseconds. If
* the timeout is reached, the response is considered completed and will be submitted.
*
* @return timeoutMills
*/
@JsonProperty(JSON_PROPERTY_TIMEOUT_MILLS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getTimeoutMills() {
return timeoutMills;
}
@JsonIgnore
public boolean getTimeoutMillsDefined() {
return timeoutMillsDefined;
}
@JsonProperty(JSON_PROPERTY_TIMEOUT_MILLS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTimeoutMills(Integer timeoutMills) {
this.timeoutMills = timeoutMills;
this.timeoutMillsDefined = true;
}
public MenuDto maxTimeoutMills(Integer maxTimeoutMills) {
this.maxTimeoutMills = maxTimeoutMills;
this.maxTimeoutMillsDefined = true;
return this;
}
/**
* Sets a limit for the maximum amount of time allowed to collect voice input.
*
* @return maxTimeoutMills
*/
@JsonProperty(JSON_PROPERTY_MAX_TIMEOUT_MILLS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getMaxTimeoutMills() {
return maxTimeoutMills;
}
@JsonIgnore
public boolean getMaxTimeoutMillsDefined() {
return maxTimeoutMillsDefined;
}
@JsonProperty(JSON_PROPERTY_MAX_TIMEOUT_MILLS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMaxTimeoutMills(Integer maxTimeoutMills) {
this.maxTimeoutMills = maxTimeoutMills;
this.maxTimeoutMillsDefined = true;
}
public MenuDto options(List options) {
this.options = options;
this.optionsDefined = true;
return this;
}
public MenuDto addOptionsItem(OptionDto optionsItem) {
if (this.options == null) {
this.options = new ArrayList<>();
}
this.optionsDefined = true;
this.options.add(optionsItem);
return this;
}
/**
* The set of options available in the menu.
*
* @return options
*/
@JsonProperty(JSON_PROPERTY_OPTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getOptions() {
return options;
}
@JsonIgnore
public boolean getOptionsDefined() {
return optionsDefined;
}
@JsonProperty(JSON_PROPERTY_OPTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOptions(List options) {
this.options = options;
this.optionsDefined = true;
}
/** Return true if this menu object is equal to o. */
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MenuDto menu = (MenuDto) o;
return Objects.equals(this.id, menu.id)
&& Objects.equals(this.mainPrompt, menu.mainPrompt)
&& Objects.equals(this.repeatPrompt, menu.repeatPrompt)
&& Objects.equals(this.repeats, menu.repeats)
&& Objects.equals(this.maxDigits, menu.maxDigits)
&& Objects.equals(this.timeoutMills, menu.timeoutMills)
&& Objects.equals(this.maxTimeoutMills, menu.maxTimeoutMills)
&& Objects.equals(this.options, menu.options);
}
@Override
public int hashCode() {
return Objects.hash(
id, mainPrompt, repeatPrompt, repeats, maxDigits, timeoutMills, maxTimeoutMills, options);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MenuDto {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" mainPrompt: ").append(toIndentedString(mainPrompt)).append("\n");
sb.append(" repeatPrompt: ").append(toIndentedString(repeatPrompt)).append("\n");
sb.append(" repeats: ").append(toIndentedString(repeats)).append("\n");
sb.append(" maxDigits: ").append(toIndentedString(maxDigits)).append("\n");
sb.append(" timeoutMills: ").append(toIndentedString(timeoutMills)).append("\n");
sb.append(" maxTimeoutMills: ").append(toIndentedString(maxTimeoutMills)).append("\n");
sb.append(" options: ").append(toIndentedString(options)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy