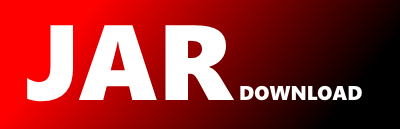
com.sinch.sdk.domains.voice.models.dto.v1.NotifyRequestDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Voice API | Sinch
* The Voice API exposes calling- and conference-related functionality in the Sinch Voice Platform.
*
* The version of the OpenAPI document: 1.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.voice.models.dto.v1;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonValue;
import com.sinch.sdk.core.utils.databind.JSONNavigator;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/** The request body of a Notify Event. */
@JsonPropertyOrder({
NotifyRequestDto.JSON_PROPERTY_EVENT,
NotifyRequestDto.JSON_PROPERTY_TYPE,
NotifyRequestDto.JSON_PROPERTY_CUSTOM
})
@JsonFilter("uninitializedFilter")
@JsonInclude(value = JsonInclude.Include.CUSTOM)
/*@JsonIgnoreProperties(
value = "event", // ignore manually set event, it will be automatically generated by Jackson during serialization
allowSetters = true // allows the event to be set during deserialization
)*/
@JsonTypeInfo(
use = JsonTypeInfo.Id.NONE,
include = JsonTypeInfo.As.EXISTING_PROPERTY,
property = "event",
visible = true)
public class NotifyRequestDto extends WebhooksEventRequestDto {
private static final long serialVersionUID = 1L;
/** Must have the value `notify`. */
public enum EventEnum {
NOTIFY("notify"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
EventEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static EventEnum fromValue(String value) {
for (EventEnum b : EventEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_EVENT = "event";
private String event;
private boolean eventDefined = false;
public static final String JSON_PROPERTY_TYPE = "type";
private String type;
private boolean typeDefined = false;
public static final String JSON_PROPERTY_CUSTOM = "custom";
private String custom;
private boolean customDefined = false;
public NotifyRequestDto() {}
public NotifyRequestDto event(String event) {
this.event = event;
this.eventDefined = true;
return this;
}
/**
* Must have the value `notify`.
*
* @return event
*/
@JsonProperty(JSON_PROPERTY_EVENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEvent() {
return event;
}
@JsonIgnore
public boolean getEventDefined() {
return eventDefined;
}
@JsonProperty(JSON_PROPERTY_EVENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEvent(String event) {
this.event = event;
this.eventDefined = true;
}
public NotifyRequestDto type(String type) {
this.type = type;
this.typeDefined = true;
return this;
}
/**
* The type of information communicated in the notification.
*
* @return type
*/
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getType() {
return type;
}
@JsonIgnore
public boolean getTypeDefined() {
return typeDefined;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setType(String type) {
this.type = type;
this.typeDefined = true;
}
public NotifyRequestDto custom(String custom) {
this.custom = custom;
this.customDefined = true;
return this;
}
/**
* An optional parameter containing notification-specific information.
*
* @return custom
*/
@JsonProperty(JSON_PROPERTY_CUSTOM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCustom() {
return custom;
}
@JsonIgnore
public boolean getCustomDefined() {
return customDefined;
}
@JsonProperty(JSON_PROPERTY_CUSTOM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCustom(String custom) {
this.custom = custom;
this.customDefined = true;
}
@Override
public NotifyRequestDto callid(String callid) {
this.setCallid(callid);
return this;
}
@Override
public NotifyRequestDto version(Integer version) {
this.setVersion(version);
return this;
}
/** Return true if this notifyRequest object is equal to o. */
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
NotifyRequestDto notifyRequest = (NotifyRequestDto) o;
return Objects.equals(this.event, notifyRequest.event)
&& Objects.equals(this.type, notifyRequest.type)
&& Objects.equals(this.custom, notifyRequest.custom)
&& super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(event, type, custom, super.hashCode());
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class NotifyRequestDto {\n");
sb.append(" ").append(toIndentedString(super.toString())).append("\n");
sb.append(" event: ").append(toIndentedString(event)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" custom: ").append(toIndentedString(custom)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
static {
// Initialize and register the discriminator mappings.
Map> mappings = new HashMap>();
mappings.put("notifyRequest", NotifyRequestDto.class);
JSONNavigator.registerDiscriminator(NotifyRequestDto.class, "event", mappings);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy