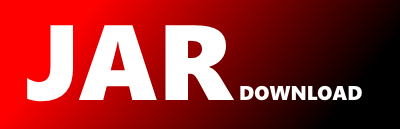
com.sinch.sdk.domains.voice.models.dto.v1.SvamlActionDto Maven / Gradle / Ivy
Show all versions of sinch-sdk-java Show documentation
/*
* Voice API | Sinch
* The Voice API exposes calling- and conference-related functionality in the Sinch Voice Platform.
*
* The version of the OpenAPI document: 1.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.voice.models.dto.v1;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.SerializerProvider;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import com.fasterxml.jackson.databind.ser.std.StdSerializer;
import com.sinch.sdk.core.models.AbstractOpenApiSchema;
import com.sinch.sdk.core.utils.databind.JSONNavigator;
import java.io.IOException;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
@JsonDeserialize(using = SvamlActionDto.SvamlActionDtoDeserializer.class)
@JsonSerialize(using = SvamlActionDto.SvamlActionDtoSerializer.class)
public final class SvamlActionDto extends AbstractOpenApiSchema {
private static final Logger log = Logger.getLogger(SvamlActionDto.class.getName());
public static final class SvamlActionDtoSerializer extends StdSerializer {
private static final long serialVersionUID = 1L;
public SvamlActionDtoSerializer(Class t) {
super(t);
}
public SvamlActionDtoSerializer() {
this(null);
}
@Override
public void serialize(SvamlActionDto value, JsonGenerator jgen, SerializerProvider provider)
throws IOException, JsonProcessingException {
jgen.writeObject(value.getActualInstance());
}
}
public static final class SvamlActionDtoDeserializer extends StdDeserializer {
private static final long serialVersionUID = 1L;
public SvamlActionDtoDeserializer() {
this(SvamlActionDto.class);
}
public SvamlActionDtoDeserializer(Class> vc) {
super(vc);
}
@Override
public SvamlActionDto deserialize(JsonParser jp, DeserializationContext ctxt)
throws IOException, JsonProcessingException {
JsonNode tree = jp.readValueAsTree();
Object deserialized = null;
Class> cls = JSONNavigator.getClassForElement(tree, SvamlActionDto.class);
if (cls != null) {
// When the OAS schema includes a discriminator, use the discriminator value to
// discriminate the anyOf schemas.
// Get the discriminator mapping value to get the class.
deserialized = tree.traverse(jp.getCodec()).readValueAs(cls);
SvamlActionDto ret = new SvamlActionDto();
ret.setActualInstance(deserialized);
return ret;
}
// deserialize SvamlActionConnectConfDto
try {
deserialized = tree.traverse(jp.getCodec()).readValueAs(SvamlActionConnectConfDto.class);
SvamlActionDto ret = new SvamlActionDto();
ret.setActualInstance(deserialized);
return ret;
} catch (Exception e) {
// deserialization failed, continue, log to help debugging
log.log(Level.FINER, "Input data does not match 'SvamlActionDto'", e);
}
// deserialize SvamlActionConnectMxpDto
try {
deserialized = tree.traverse(jp.getCodec()).readValueAs(SvamlActionConnectMxpDto.class);
SvamlActionDto ret = new SvamlActionDto();
ret.setActualInstance(deserialized);
return ret;
} catch (Exception e) {
// deserialization failed, continue, log to help debugging
log.log(Level.FINER, "Input data does not match 'SvamlActionDto'", e);
}
// deserialize SvamlActionConnectPstnDto
try {
deserialized = tree.traverse(jp.getCodec()).readValueAs(SvamlActionConnectPstnDto.class);
SvamlActionDto ret = new SvamlActionDto();
ret.setActualInstance(deserialized);
return ret;
} catch (Exception e) {
// deserialization failed, continue, log to help debugging
log.log(Level.FINER, "Input data does not match 'SvamlActionDto'", e);
}
// deserialize SvamlActionConnectSipDto
try {
deserialized = tree.traverse(jp.getCodec()).readValueAs(SvamlActionConnectSipDto.class);
SvamlActionDto ret = new SvamlActionDto();
ret.setActualInstance(deserialized);
return ret;
} catch (Exception e) {
// deserialization failed, continue, log to help debugging
log.log(Level.FINER, "Input data does not match 'SvamlActionDto'", e);
}
// deserialize SvamlActionContinueDto
try {
deserialized = tree.traverse(jp.getCodec()).readValueAs(SvamlActionContinueDto.class);
SvamlActionDto ret = new SvamlActionDto();
ret.setActualInstance(deserialized);
return ret;
} catch (Exception e) {
// deserialization failed, continue, log to help debugging
log.log(Level.FINER, "Input data does not match 'SvamlActionDto'", e);
}
// deserialize SvamlActionHangupDto
try {
deserialized = tree.traverse(jp.getCodec()).readValueAs(SvamlActionHangupDto.class);
SvamlActionDto ret = new SvamlActionDto();
ret.setActualInstance(deserialized);
return ret;
} catch (Exception e) {
// deserialization failed, continue, log to help debugging
log.log(Level.FINER, "Input data does not match 'SvamlActionDto'", e);
}
// deserialize SvamlActionParkDto
try {
deserialized = tree.traverse(jp.getCodec()).readValueAs(SvamlActionParkDto.class);
SvamlActionDto ret = new SvamlActionDto();
ret.setActualInstance(deserialized);
return ret;
} catch (Exception e) {
// deserialization failed, continue, log to help debugging
log.log(Level.FINER, "Input data does not match 'SvamlActionDto'", e);
}
// deserialize SvamlActionRunMenuDto
try {
deserialized = tree.traverse(jp.getCodec()).readValueAs(SvamlActionRunMenuDto.class);
SvamlActionDto ret = new SvamlActionDto();
ret.setActualInstance(deserialized);
return ret;
} catch (Exception e) {
// deserialization failed, continue, log to help debugging
log.log(Level.FINER, "Input data does not match 'SvamlActionDto'", e);
}
throw new IOException(
String.format("Failed deserialization for SvamlActionDto: no match found"));
}
/** Handle deserialization of the 'null' value. */
@Override
public SvamlActionDto getNullValue(DeserializationContext ctxt) throws JsonMappingException {
throw new JsonMappingException(ctxt.getParser(), "SvamlActionDto cannot be null");
}
}
// store a list of schema names defined in anyOf
public static final Map> schemas = new HashMap>();
public SvamlActionDto() {
super("anyOf", Boolean.FALSE);
}
public SvamlActionDto(SvamlActionConnectConfDto o) {
super("anyOf", Boolean.FALSE);
setActualInstance(o);
}
public SvamlActionDto(SvamlActionConnectMxpDto o) {
super("anyOf", Boolean.FALSE);
setActualInstance(o);
}
public SvamlActionDto(SvamlActionConnectPstnDto o) {
super("anyOf", Boolean.FALSE);
setActualInstance(o);
}
public SvamlActionDto(SvamlActionConnectSipDto o) {
super("anyOf", Boolean.FALSE);
setActualInstance(o);
}
public SvamlActionDto(SvamlActionContinueDto o) {
super("anyOf", Boolean.FALSE);
setActualInstance(o);
}
public SvamlActionDto(SvamlActionHangupDto o) {
super("anyOf", Boolean.FALSE);
setActualInstance(o);
}
public SvamlActionDto(SvamlActionParkDto o) {
super("anyOf", Boolean.FALSE);
setActualInstance(o);
}
public SvamlActionDto(SvamlActionRunMenuDto o) {
super("anyOf", Boolean.FALSE);
setActualInstance(o);
}
static {
schemas.put("SvamlActionConnectConfDto", SvamlActionConnectConfDto.class);
schemas.put("SvamlActionConnectMxpDto", SvamlActionConnectMxpDto.class);
schemas.put("SvamlActionConnectPstnDto", SvamlActionConnectPstnDto.class);
schemas.put("SvamlActionConnectSipDto", SvamlActionConnectSipDto.class);
schemas.put("SvamlActionContinueDto", SvamlActionContinueDto.class);
schemas.put("SvamlActionHangupDto", SvamlActionHangupDto.class);
schemas.put("SvamlActionParkDto", SvamlActionParkDto.class);
schemas.put("SvamlActionRunMenuDto", SvamlActionRunMenuDto.class);
JSONNavigator.registerDescendants(SvamlActionDto.class, Collections.unmodifiableMap(schemas));
// Initialize and register the discriminator mappings.
Map> mappings = new HashMap>();
mappings.put("connectConf", SvamlActionConnectConfDto.class);
mappings.put("connectMxp", SvamlActionConnectMxpDto.class);
mappings.put("connectPstn", SvamlActionConnectPstnDto.class);
mappings.put("connectSip", SvamlActionConnectSipDto.class);
mappings.put("continue", SvamlActionContinueDto.class);
mappings.put("hangup", SvamlActionHangupDto.class);
mappings.put("park", SvamlActionParkDto.class);
mappings.put("runMenu", SvamlActionRunMenuDto.class);
mappings.put("svaml.action.connectConf", SvamlActionConnectConfDto.class);
mappings.put("svaml.action.connectMxp", SvamlActionConnectMxpDto.class);
mappings.put("svaml.action.connectPstn", SvamlActionConnectPstnDto.class);
mappings.put("svaml.action.connectSip", SvamlActionConnectSipDto.class);
mappings.put("svaml.action.continue", SvamlActionContinueDto.class);
mappings.put("svaml.action.hangup", SvamlActionHangupDto.class);
mappings.put("svaml.action.park", SvamlActionParkDto.class);
mappings.put("svaml.action.runMenu", SvamlActionRunMenuDto.class);
mappings.put("svaml.action", SvamlActionDto.class);
JSONNavigator.registerDiscriminator(SvamlActionDto.class, "name", mappings);
}
@Override
public Map> getSchemas() {
return SvamlActionDto.schemas;
}
/**
* Set the instance that matches the anyOf child schema, check the instance parameter is valid
* against the anyOf child schemas: SvamlActionConnectConfDto, SvamlActionConnectMxpDto,
* SvamlActionConnectPstnDto, SvamlActionConnectSipDto, SvamlActionContinueDto,
* SvamlActionHangupDto, SvamlActionParkDto, SvamlActionRunMenuDto
*
* It could be an instance of the 'anyOf' schemas. The anyOf child schemas may themselves be a
* composed schema (allOf, anyOf, anyOf).
*/
@Override
public void setActualInstance(Object instance) {
if (JSONNavigator.isInstanceOf(
SvamlActionConnectConfDto.class, instance, new HashSet>())) {
super.setActualInstance(instance);
return;
}
if (JSONNavigator.isInstanceOf(
SvamlActionConnectMxpDto.class, instance, new HashSet>())) {
super.setActualInstance(instance);
return;
}
if (JSONNavigator.isInstanceOf(
SvamlActionConnectPstnDto.class, instance, new HashSet>())) {
super.setActualInstance(instance);
return;
}
if (JSONNavigator.isInstanceOf(
SvamlActionConnectSipDto.class, instance, new HashSet>())) {
super.setActualInstance(instance);
return;
}
if (JSONNavigator.isInstanceOf(
SvamlActionContinueDto.class, instance, new HashSet>())) {
super.setActualInstance(instance);
return;
}
if (JSONNavigator.isInstanceOf(SvamlActionHangupDto.class, instance, new HashSet>())) {
super.setActualInstance(instance);
return;
}
if (JSONNavigator.isInstanceOf(SvamlActionParkDto.class, instance, new HashSet>())) {
super.setActualInstance(instance);
return;
}
if (JSONNavigator.isInstanceOf(
SvamlActionRunMenuDto.class, instance, new HashSet>())) {
super.setActualInstance(instance);
return;
}
throw new RuntimeException(
"Invalid instance type. Must be SvamlActionConnectConfDto, SvamlActionConnectMxpDto,"
+ " SvamlActionConnectPstnDto, SvamlActionConnectSipDto, SvamlActionContinueDto,"
+ " SvamlActionHangupDto, SvamlActionParkDto, SvamlActionRunMenuDto");
}
/**
* Get the actual instance, which can be the following: SvamlActionConnectConfDto,
* SvamlActionConnectMxpDto, SvamlActionConnectPstnDto, SvamlActionConnectSipDto,
* SvamlActionContinueDto, SvamlActionHangupDto, SvamlActionParkDto, SvamlActionRunMenuDto
*
* @return The actual instance (SvamlActionConnectConfDto, SvamlActionConnectMxpDto,
* SvamlActionConnectPstnDto, SvamlActionConnectSipDto, SvamlActionContinueDto,
* SvamlActionHangupDto, SvamlActionParkDto, SvamlActionRunMenuDto)
*/
@Override
public Object getActualInstance() {
return super.getActualInstance();
}
/**
* Get the actual instance of `SvamlActionConnectConfDto`. If the actual instance is not
* `SvamlActionConnectConfDto`, the ClassCastException will be thrown.
*
* @return The actual instance of `SvamlActionConnectConfDto`
* @throws ClassCastException if the instance is not `SvamlActionConnectConfDto`
*/
public SvamlActionConnectConfDto getSvamlActionConnectConfDto() throws ClassCastException {
return (SvamlActionConnectConfDto) super.getActualInstance();
}
/**
* Get the actual instance of `SvamlActionConnectMxpDto`. If the actual instance is not
* `SvamlActionConnectMxpDto`, the ClassCastException will be thrown.
*
* @return The actual instance of `SvamlActionConnectMxpDto`
* @throws ClassCastException if the instance is not `SvamlActionConnectMxpDto`
*/
public SvamlActionConnectMxpDto getSvamlActionConnectMxpDto() throws ClassCastException {
return (SvamlActionConnectMxpDto) super.getActualInstance();
}
/**
* Get the actual instance of `SvamlActionConnectPstnDto`. If the actual instance is not
* `SvamlActionConnectPstnDto`, the ClassCastException will be thrown.
*
* @return The actual instance of `SvamlActionConnectPstnDto`
* @throws ClassCastException if the instance is not `SvamlActionConnectPstnDto`
*/
public SvamlActionConnectPstnDto getSvamlActionConnectPstnDto() throws ClassCastException {
return (SvamlActionConnectPstnDto) super.getActualInstance();
}
/**
* Get the actual instance of `SvamlActionConnectSipDto`. If the actual instance is not
* `SvamlActionConnectSipDto`, the ClassCastException will be thrown.
*
* @return The actual instance of `SvamlActionConnectSipDto`
* @throws ClassCastException if the instance is not `SvamlActionConnectSipDto`
*/
public SvamlActionConnectSipDto getSvamlActionConnectSipDto() throws ClassCastException {
return (SvamlActionConnectSipDto) super.getActualInstance();
}
/**
* Get the actual instance of `SvamlActionContinueDto`. If the actual instance is not
* `SvamlActionContinueDto`, the ClassCastException will be thrown.
*
* @return The actual instance of `SvamlActionContinueDto`
* @throws ClassCastException if the instance is not `SvamlActionContinueDto`
*/
public SvamlActionContinueDto getSvamlActionContinueDto() throws ClassCastException {
return (SvamlActionContinueDto) super.getActualInstance();
}
/**
* Get the actual instance of `SvamlActionHangupDto`. If the actual instance is not
* `SvamlActionHangupDto`, the ClassCastException will be thrown.
*
* @return The actual instance of `SvamlActionHangupDto`
* @throws ClassCastException if the instance is not `SvamlActionHangupDto`
*/
public SvamlActionHangupDto getSvamlActionHangupDto() throws ClassCastException {
return (SvamlActionHangupDto) super.getActualInstance();
}
/**
* Get the actual instance of `SvamlActionParkDto`. If the actual instance is not
* `SvamlActionParkDto`, the ClassCastException will be thrown.
*
* @return The actual instance of `SvamlActionParkDto`
* @throws ClassCastException if the instance is not `SvamlActionParkDto`
*/
public SvamlActionParkDto getSvamlActionParkDto() throws ClassCastException {
return (SvamlActionParkDto) super.getActualInstance();
}
/**
* Get the actual instance of `SvamlActionRunMenuDto`. If the actual instance is not
* `SvamlActionRunMenuDto`, the ClassCastException will be thrown.
*
* @return The actual instance of `SvamlActionRunMenuDto`
* @throws ClassCastException if the instance is not `SvamlActionRunMenuDto`
*/
public SvamlActionRunMenuDto getSvamlActionRunMenuDto() throws ClassCastException {
return (SvamlActionRunMenuDto) super.getActualInstance();
}
}