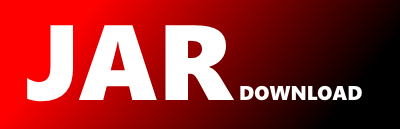
com.sinch.sdk.domains.voice.models.requests.CalloutRequestParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
package com.sinch.sdk.domains.voice.models.requests;
import com.sinch.sdk.core.models.OptionalValue;
import com.sinch.sdk.domains.voice.models.Destination;
import com.sinch.sdk.models.DualToneMultiFrequency;
import com.sinch.sdk.models.E164PhoneNumber;
public class CalloutRequestParameters {
private final OptionalValue destination;
private final OptionalValue cli;
private final OptionalValue dtfm;
private final OptionalValue custom;
protected CalloutRequestParameters(
OptionalValue destination,
OptionalValue cli,
OptionalValue dtfm,
OptionalValue custom) {
this.destination = destination;
this.cli = cli;
this.dtfm = dtfm;
this.custom = custom;
}
/**
* Destination getter
*
* @see Builder#setDestination(Destination)
*/
public OptionalValue getDestination() {
return destination;
}
/**
* Cli getter
*
* @see Builder#setCli(E164PhoneNumber)
*/
public OptionalValue getCli() {
return cli;
}
/**
* Dual Tone Multi Frequency getter
*
* @see Builder#setDtfm(DualToneMultiFrequency)
*/
public OptionalValue getDtfm() {
return dtfm;
}
/**
* Custom value getter
*
* @see Builder#setCustom(String)
*/
public OptionalValue getCustom() {
return custom;
}
@Override
public String toString() {
return "CalloutRequestParameters{"
+ "destination="
+ destination
+ ", cli="
+ cli
+ ", dtfm="
+ dtfm
+ ", custom="
+ custom
+ '}';
}
public static Builder> builder() {
return new Builder<>();
}
public static class Builder> {
OptionalValue destination = OptionalValue.empty();
OptionalValue cli = OptionalValue.empty();
OptionalValue dtfm = OptionalValue.empty();
OptionalValue custom = OptionalValue.empty();
public Builder() {}
/**
* @param destination The type of device and number or endpoint to call
* @return current builder
*/
public B setDestination(Destination destination) {
this.destination = OptionalValue.of(destination);
return self();
}
/**
* @param cli The number that will be displayed as the incoming caller. To set your own CLI, you
* may use your verified number or your Dashboard number
* @return current builder
*/
public B setCli(E164PhoneNumber cli) {
this.cli = OptionalValue.of(cli);
return self();
}
/**
* @param dtfm When the destination picks up, this DTMF tones will be played to the callee.
* @return current builder
*/
public B setDtfm(DualToneMultiFrequency dtfm) {
this.dtfm = OptionalValue.of(dtfm);
return self();
}
/**
* @param custom Custom data
* @return current builder
*/
public B setCustom(String custom) {
this.custom = OptionalValue.of(custom);
return self();
}
@SuppressWarnings("unchecked")
protected B self() {
return (B) this;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy