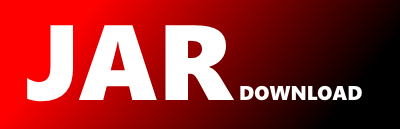
com.sinch.sdk.domains.voice.models.requests.CalloutRequestParametersCustom Maven / Gradle / Ivy
Show all versions of sinch-sdk-java Show documentation
package com.sinch.sdk.domains.voice.models.requests;
import com.sinch.sdk.core.models.OptionalValue;
import com.sinch.sdk.domains.voice.models.Destination;
import com.sinch.sdk.domains.voice.models.requests.CalloutRequestParametersConference.Builder;
import com.sinch.sdk.models.DualToneMultiFrequency;
import com.sinch.sdk.models.E164PhoneNumber;
/**
* The custom callout, the server initiates a call from the servers that can be controlled by
* specifying how the call should progress at each call event.
*/
public class CalloutRequestParametersCustom extends CalloutRequestParameters {
private final OptionalValue maxDuration;
private final OptionalValue ice;
private final OptionalValue ace;
private final OptionalValue pie;
private CalloutRequestParametersCustom(
OptionalValue destination,
OptionalValue cli,
OptionalValue dtfm,
OptionalValue custom,
OptionalValue maxDuration,
OptionalValue ice,
OptionalValue ace,
OptionalValue pie) {
super(destination, cli, dtfm, custom);
this.maxDuration = maxDuration;
this.ice = ice;
this.ace = ace;
this.pie = pie;
}
/**
* See builder
*
* @see Builder#setMaxDuration(Integer)
*/
public OptionalValue getMaxDuration() {
return maxDuration;
}
/**
* See builder
*
* @see Builder#setIce(Control)
*/
public OptionalValue getIce() {
return ice;
}
/**
* See builder
*
* @see Builder#setAce(Control)
*/
public OptionalValue getAce() {
return ace;
}
/**
* See builder
*
* @see Builder#setPie(Control)
*/
public OptionalValue getPie() {
return pie;
}
@Override
public String toString() {
return "CalloutRequestParametersCustom{"
+ "maxDuration="
+ maxDuration
+ ", ice='"
+ ice
+ '\''
+ ", ace='"
+ ace
+ '\''
+ ", pie='"
+ pie
+ '\''
+ "} "
+ super.toString();
}
public static Builder builder() {
return new Builder();
}
public static class Builder extends CalloutRequestParameters.Builder {
OptionalValue maxDuration = OptionalValue.empty();
OptionalValue ice = OptionalValue.empty();
OptionalValue ace = OptionalValue.empty();
OptionalValue pie = OptionalValue.empty();
public Builder() {
super();
}
/**
* The maximum amount of time in seconds that the call will last.
*
* @param maxDuration Max duration value
* @return current builder
*/
public Builder setMaxDuration(Integer maxDuration) {
this.maxDuration = OptionalValue.of(maxDuration);
return self();
}
/**
* You can use inline SVAML to replace a
* callback URL when using custom callouts.
*
* Ensure that the JSON object is escaped correctly
*
*
If inline ICE SVAML is passed, exclude cli and destination properties from the
* customCallout request body. Example:
* "{\"action\": {\"name\": \"RunMenu\",\"locale\": \"en-US\",\"menus\": [{\"id\": \"main\",\"mainPrompt\": \"#tts[ Welcome to the main menu. Press 1 for a callback or 2 for a cancel<\/speak>]\",\"timeoutMills\": 5000,\"options\": [ {\"dtmf\": \"1\",\"action\": \"return(callback)\"}, {\"dtmf\": \"2\",\"action\": \"return(cancel)\"}]}]}}""
*
*
* @param ice The Incoming Call Event value
* @return current builder
*/
public Builder setIce(Control ice) {
this.ice = OptionalValue.of(ice);
return self();
}
/**
* You can use inline SVAML to replace a
* callback URL when using custom callouts.
*
*
Ensure that the JSON object is escaped correctly Example:
* "{\"action\":{\"name\":\"connectPstn\",\"number\":\"46000000001\",\"maxDuration\":90}}"
*
*
* @param ace The Answered Call Event value
* @return current builder
*/
public Builder setAce(Control ace) {
this.ace = OptionalValue.of(ace);
return self();
}
/**
* Note: PIE callbacks are not available for DATA Calls; only PSTN and SIP calls.
*
*
You can use inline SVAML to replace a
* callback URL when using custom callouts.
*
*
Ensure that the JSON object is escaped correctly. A PIE event will contain a value chosen
* from an IVR choice. Usually a PIE event wil contain a URL to a callback sever that will
* receive the choice and be able to parse it. This could result in further SVAML or some other
* application logic function.
*
* @param pie Prompt Input Event value
* @return current builder
*/
public Builder setPie(Control pie) {
this.pie = OptionalValue.of(pie);
return self();
}
public CalloutRequestParametersCustom build() {
return new CalloutRequestParametersCustom(
destination, cli, dtfm, custom, maxDuration, ice, ace, pie);
}
@Override
protected Builder self() {
return this;
}
}
}