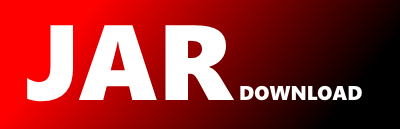
com.sinch.sdk.domains.voice.models.requests.ConferenceManageParticipantRequestParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
package com.sinch.sdk.domains.voice.models.requests;
import com.sinch.sdk.core.models.OptionalValue;
import com.sinch.sdk.domains.voice.models.MusicOnHoldType;
/** Use to configure conference participant settings */
public class ConferenceManageParticipantRequestParameters {
private final OptionalValue command;
private final OptionalValue musicOnHold;
private ConferenceManageParticipantRequestParameters(
OptionalValue command,
OptionalValue musicOnHold) {
this.command = command;
this.musicOnHold = musicOnHold;
}
/**
* @see Builder#setCommand(ConferenceManageParticipantCommandType)
*/
public OptionalValue getCommand() {
return command;
}
/**
* @see Builder#setMusicOnHold(MusicOnHoldType) (MusicOnHoldType)
*/
public OptionalValue getMusicOnHold() {
return musicOnHold;
}
@Override
public String toString() {
return "ConferenceManageParticipantRequestParameters{"
+ "command="
+ command
+ ", musicOnHold="
+ musicOnHold
+ '}';
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
OptionalValue command = OptionalValue.empty();
OptionalValue musicOnHold = OptionalValue.empty();
/**
* @param command Action to apply on conference participant.
* @return current builder
*/
public Builder setCommand(ConferenceManageParticipantCommandType command) {
this.command = OptionalValue.of(command);
return this;
}
/**
* @param musicOnHold If this optional parameter is included, plays music to the first
* participant in a conference while they're alone and waiting for other participants to
* join. If musicOnHold isn't specified, the user will only hear silence while alone in the
* conference. This property is only available to use with the onhold command.
* @return current builder
*/
public Builder setMusicOnHold(MusicOnHoldType musicOnHold) {
this.musicOnHold = OptionalValue.of(musicOnHold);
return this;
}
public ConferenceManageParticipantRequestParameters build() {
return new ConferenceManageParticipantRequestParameters(command, musicOnHold);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy