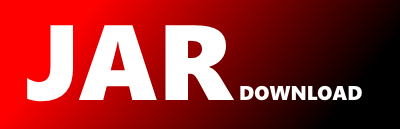
com.sinch.sdk.domains.voice.models.svaml.ActionConnectMxp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
package com.sinch.sdk.domains.voice.models.svaml;
import com.sinch.sdk.core.models.OptionalValue;
import com.sinch.sdk.core.utils.Pair;
import com.sinch.sdk.domains.voice.models.Destination;
import java.util.Collection;
public class ActionConnectMxp extends Action {
private final OptionalValue destination;
private final OptionalValue>> callheaders;
private ActionConnectMxp(
OptionalValue destination,
OptionalValue>> callheaders) {
this.destination = destination;
this.callheaders = callheaders;
}
public OptionalValue getDestination() {
return destination;
}
public OptionalValue>> getCallheaders() {
return callheaders;
}
@Override
public String toString() {
return "ActionConnectMxp{"
+ "destination="
+ destination
+ ", callheaders="
+ callheaders
+ "} "
+ super.toString();
}
public static Builder> builder() {
return new Builder<>();
}
public static class Builder> {
OptionalValue destination = OptionalValue.empty();
OptionalValue>> callheaders = OptionalValue.empty();
public Builder setDestination(Destination destination) {
this.destination = OptionalValue.of(destination);
return this;
}
public Builder setCallheaders(Collection> callheaders) {
this.callheaders = OptionalValue.of(callheaders);
return this;
}
public ActionConnectMxp build() {
return new ActionConnectMxp(destination, callheaders);
}
@SuppressWarnings("unchecked")
protected B self() {
return (B) this;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy