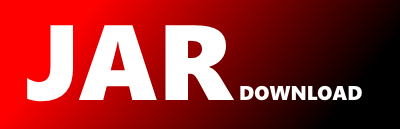
com.sinsz.wxpn.open.support.FormaterUtils Maven / Gradle / Ivy
package com.sinsz.wxpn.open.support;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.sinsz.wxpn.exception.WxpnException;
import org.apache.commons.lang3.StringUtils;
import org.nutz.json.Json;
import org.nutz.json.JsonFormat;
import javax.servlet.http.HttpServletRequest;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.security.MessageDigest;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.stream.Collectors;
/**
* 格式化接口相关参数与返回值工具
* @author chenjianbo
* @date 2018-11-15
*/
public final class FormaterUtils {
private FormaterUtils() {
}
/**
* 下划线转驼峰
* @param arg0
* @return
*/
private synchronized static String underline2Hump(String arg0) {
if (arg0 == null || "".equals(arg0.trim())) {
return "";
}
int len = arg0.length();
StringBuilder builder = new StringBuilder(len);
for (int i = 0; i < len; i++) {
char c = arg0.charAt(i);
if (c == '_') {
if (++i < len) {
builder.append(Character.toUpperCase(arg0.charAt(i)));
}
} else {
builder.append(c);
}
}
return builder.toString();
}
/**
* 格式化map,将key转换为驼峰模式
*
* 待优化
*
* @param map 待转换对象
* @return 新的map
*/
@SuppressWarnings(value = {"unchecked"})
private static Map format(Map map) {
return map.entrySet()
.stream()
.filter(entry -> entry.getValue() != null)
.map(entry -> {
if (entry.getValue() instanceof Map) {
return new DefaultEntry(FormaterUtils.underline2Hump(entry.getKey()), format((Map) entry.getValue()));
} else if (entry.getValue() instanceof List) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy