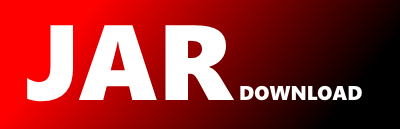
com.sinszm.common.Response Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of szm-boot-common Show documentation
Show all versions of szm-boot-common Show documentation
基于SpringBoot扩展生态应用公共组件
Copyright © 2020 智慧程序猿 All rights reserved.
https://www.sinsz.com
package com.sinszm.common;
import com.sinszm.common.exception.ApiError;
import com.sinszm.common.exception.SystemApiError;
import org.nutz.json.Json;
/**
* 统一返回值对象
*
* @author chenjianbo
*/
public class Response {
private static final String MESSAGE = "SUCCESS";
/**
* 状态
*/
private Boolean state;
/**
* 请求消息内容
*/
private String message;
/**
* 返回值对象
*/
private T body;
/**
* 异常栈
*/
private Throwable cause;
private Response() {
}
public Boolean getState() {
return state;
}
private void setState(Boolean state) {
this.state = state;
}
public String getMessage() {
return message;
}
private void setMessage(String message) {
this.message = message;
}
public T getBody() {
return body;
}
private void setBody(T body) {
this.body = body;
}
public Throwable getCause() {
return cause;
}
private void setCause(Throwable cause) {
this.cause = cause;
}
/**
* 成功返回
* @param body 返回值
* @param 类型
* @return 响应
*/
public synchronized static Response success(T body) {
Response response = new Response<>();
response.setState(true);
response.setMessage(MESSAGE);
if (body != null) {
response.setBody(body);
}
response.setCause(null);
return response;
}
/**
* 失败返回
* @param apiError 错误代码
* @param cause 异常栈
* @return 响应
*/
public synchronized static Response
© 2015 - 2025 Weber Informatics LLC | Privacy Policy