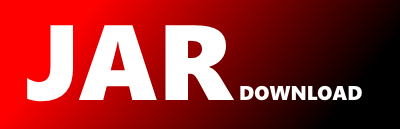
com.sinszm.common.util.SignUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of szm-boot-common Show documentation
Show all versions of szm-boot-common Show documentation
基于SpringBoot扩展生态应用公共组件
Copyright © 2020 智慧程序猿 All rights reserved.
https://www.sinsz.com
package com.sinszm.common.util;
import com.sinszm.common.exception.ApiException;
import org.apache.commons.codec.digest.DigestUtils;
import org.springframework.util.StringUtils;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.security.MessageDigest;
import java.util.List;
import java.util.SortedMap;
import static com.sinszm.common.exception.SystemApiError.*;
import static java.util.stream.Collectors.toList;
/**
* 签名工具
*
* @author chenjianbo
*/
public final class SignUtils {
/**
* 签名方式
*/
public enum Method {
MD5,
HMAC_SHA256,
SHA1
}
/**
* 创建签名
* @param map 参数集合
* @param secretKey 签名密钥
* @param method 签名方式
* @return 签名字符串
*/
public static String createSign(SortedMap map, String secretKey, Method method) {
if (map == null || map.isEmpty()) {
throw new ApiException(SIGN_ERROR_01);
}
if (StringUtils.isEmpty(secretKey)) {
throw new ApiException(SIGN_ERROR_02);
}
if (method == null) {
throw new ApiException(SIGN_ERROR_03);
}
List list = map.entrySet()
.stream()
.filter(e -> !StringUtils.isEmpty(e.getValue()))
.map(e -> e.getKey() + "=" + String.valueOf(e.getValue()))
.collect(toList());
list.add("key=" + secretKey);
String sourceStr = String.join("&", list);
switch (method) {
case SHA1:
return generate(sourceStr, DigestUtils.getSha1Digest().getAlgorithm());
case HMAC_SHA256:
return hmacSha256(sourceStr, secretKey);
case MD5:
default:
return generate(sourceStr, DigestUtils.getMd5Digest().getAlgorithm());
}
}
/**
* SHA1或MD5加密
* @param message 加密内容
* @param name 加密方式
* @return 加密串
*/
private static String generate(final String message, String name) {
final byte[] btInput = message.getBytes();
final char[] hexDigits = {'0', '1', '2', '3', '4', '5', '6', '7', '8',
'9', 'A', 'B', 'C', 'D', 'E', 'F'};
try {
final MessageDigest mdInst = DigestUtils.getDigest(name);
mdInst.update(btInput);
// 获得密文
final byte[] md = mdInst.digest();
// 把密文转换成十六进制的字符串形式
final int j = md.length;
final char[] str = new char[j * 2];
int k = 0;
for (final byte byte0 : md) {
str[k++] = hexDigits[byte0 >>> 4 & 0xf];
str[k++] = hexDigits[byte0 & 0xf];
}
return new String(str).toUpperCase();
} catch (final Exception e) {
e.printStackTrace(System.err);
throw new ApiException(SIGN_ERROR_04);
}
}
/**
* HMAC-SHA256加密
* @param message 加密内容
* @param secret 加密密钥
* @return 加密串
*/
private static String hmacSha256(String message, String secret) {
try {
Mac sha256 = Mac.getInstance("HmacSHA256");
SecretKeySpec secretKey = new SecretKeySpec(secret.getBytes(), "HmacSHA256");
sha256.init(secretKey);
byte[] bytes = sha256.doFinal(message.getBytes());
StringBuilder hs = new StringBuilder();
for (int n = 0; bytes!=null && n < bytes.length; n++) {
String stmp = Integer.toHexString(bytes[n] & 0XFF);
if (stmp.length() == 1) {
hs.append('0');
}
hs.append(stmp);
}
return hs.toString().toUpperCase();
} catch (Exception e) {
e.printStackTrace(System.err);
throw new ApiException(SIGN_ERROR_04);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy