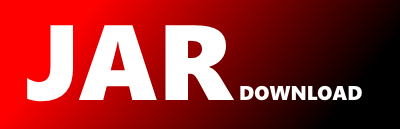
com.sirolf2009.objectchain.example.common.model.ChatState Maven / Gradle / Ivy
The newest version!
package com.sirolf2009.objectchain.example.common.model;
import com.esotericsoftware.kryo.Kryo;
import com.sirolf2009.objectchain.common.crypto.Hashing;
import com.sirolf2009.objectchain.common.interfaces.IState;
import com.sirolf2009.objectchain.common.model.Block;
import com.sirolf2009.objectchain.common.model.Mutation;
import com.sirolf2009.objectchain.example.common.model.ClaimUsername;
import com.sirolf2009.objectchain.example.common.model.Message;
import java.security.PublicKey;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Stack;
import java.util.function.Consumer;
import org.eclipse.xtend.lib.annotations.Data;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Conversions;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.Pure;
import org.eclipse.xtext.xbase.lib.util.ToStringBuilder;
@Data
@SuppressWarnings("all")
public class ChatState implements IState {
private final List newChat;
private final Stack chat;
private final Map usernames;
@Override
public IState apply(final Kryo kryo, final Block block) {
final HashMap usernames = new HashMap();
usernames.putAll(this.usernames);
final Function1 _function = (Mutation it) -> {
Object _object = it.getObject();
return Boolean.valueOf((_object instanceof ClaimUsername));
};
final Consumer _function_1 = (Mutation it) -> {
Object _object = it.getObject();
usernames.put(it.getPublicKey(), ((ClaimUsername) _object).getUsername());
};
IterableExtensions.filter(block.getMutations(), _function).forEach(_function_1);
final Stack chat = new Stack();
final ArrayList newChat = new ArrayList();
chat.addAll(this.chat);
final Function1 _function_2 = (Mutation it) -> {
Object _object = it.getObject();
return Boolean.valueOf((_object instanceof Message));
};
final Consumer _function_3 = (Mutation it) -> {
StringConcatenation _builder = new StringConcatenation();
String _orDefault = usernames.getOrDefault(it.getPublicKey(), Hashing.toHexString(((List)Conversions.doWrapArray(it.getPublicKey().getEncoded()))));
_builder.append(_orDefault);
_builder.append(": ");
Object _object = it.getObject();
String _message = ((Message) _object).getMessage();
_builder.append(_message);
chat.add(_builder.toString());
StringConcatenation _builder_1 = new StringConcatenation();
String _orDefault_1 = usernames.getOrDefault(it.getPublicKey(), Hashing.toHexString(((List)Conversions.doWrapArray(it.getPublicKey().getEncoded()))));
_builder_1.append(_orDefault_1);
_builder_1.append(": ");
Object _object_1 = it.getObject();
String _message_1 = ((Message) _object_1).getMessage();
_builder_1.append(_message_1);
newChat.add(_builder_1.toString());
};
IterableExtensions.filter(block.getMutations(), _function_2).forEach(_function_3);
return new ChatState(newChat, chat, usernames);
}
public ChatState(final List newChat, final Stack chat, final Map usernames) {
super();
this.newChat = newChat;
this.chat = chat;
this.usernames = usernames;
}
@Override
@Pure
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((this.newChat== null) ? 0 : this.newChat.hashCode());
result = prime * result + ((this.chat== null) ? 0 : this.chat.hashCode());
result = prime * result + ((this.usernames== null) ? 0 : this.usernames.hashCode());
return result;
}
@Override
@Pure
public boolean equals(final Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
ChatState other = (ChatState) obj;
if (this.newChat == null) {
if (other.newChat != null)
return false;
} else if (!this.newChat.equals(other.newChat))
return false;
if (this.chat == null) {
if (other.chat != null)
return false;
} else if (!this.chat.equals(other.chat))
return false;
if (this.usernames == null) {
if (other.usernames != null)
return false;
} else if (!this.usernames.equals(other.usernames))
return false;
return true;
}
@Override
@Pure
public String toString() {
ToStringBuilder b = new ToStringBuilder(this);
b.add("newChat", this.newChat);
b.add("chat", this.chat);
b.add("usernames", this.usernames);
return b.toString();
}
@Pure
public List getNewChat() {
return this.newChat;
}
@Pure
public Stack getChat() {
return this.chat;
}
@Pure
public Map getUsernames() {
return this.usernames;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy