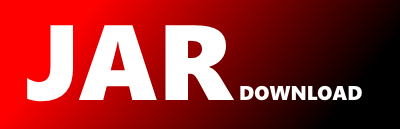
com.sirolf2009.objectchain.example.node.ChatNode Maven / Gradle / Ivy
The newest version!
package com.sirolf2009.objectchain.example.node;
import com.esotericsoftware.kryo.Kryo;
import com.sirolf2009.objectchain.common.crypto.Keys;
import com.sirolf2009.objectchain.common.interfaces.IState;
import com.sirolf2009.objectchain.common.model.Configuration;
import com.sirolf2009.objectchain.common.model.Mutation;
import com.sirolf2009.objectchain.example.common.model.ChatConfiguration;
import com.sirolf2009.objectchain.example.common.model.ChatState;
import com.sirolf2009.objectchain.example.common.model.ClaimUsername;
import com.sirolf2009.objectchain.example.common.model.Message;
import com.sirolf2009.objectchain.node.Node;
import java.net.InetSocketAddress;
import java.security.KeyPair;
import java.util.Collections;
import java.util.List;
import java.util.Scanner;
import java.util.function.Supplier;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.InputOutput;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.ObjectExtensions;
import org.eclipse.xtext.xbase.lib.Procedures.Procedure1;
import org.slf4j.Logger;
@SuppressWarnings("all")
public class ChatNode extends Node {
public ChatNode(final List trackers, final int nodePort, final KeyPair keys) {
super(new ChatConfiguration(), ((Supplier) () -> {
return ChatNode.getChatKryo();
}), trackers, nodePort, keys);
}
public ChatNode(final Logger logger, final List trackers, final int nodePort, final KeyPair keys) {
super(logger, new ChatConfiguration(), ((Supplier) () -> {
return ChatNode.getChatKryo();
}), trackers, nodePort, keys);
}
public ChatNode(final Logger logger, final Configuration configuration, final List trackers, final int nodePort, final KeyPair keys) {
super(logger, configuration, ((Supplier) () -> {
return ChatNode.getChatKryo();
}), trackers, nodePort, keys);
}
/**
* When we launch the application, we first synchronise to download any missing data. After sync we start running our chat
*/
@Override
public void onSynchronised() {
final Runnable _function = () -> {
this.getLog().info("Initialized, running chat...");
final Scanner scanner = new Scanner(System.in);
while (true) {
{
final String line = scanner.nextLine();
boolean _startsWith = line.startsWith("/");
if (_startsWith) {
boolean _startsWith_1 = line.startsWith("/claim");
if (_startsWith_1) {
ClaimUsername _claimUsername = new ClaimUsername();
final Procedure1 _function_1 = (ClaimUsername it) -> {
it.setUsername(line.replaceFirst("/claim", "").trim());
};
final ClaimUsername claim = ObjectExtensions.operator_doubleArrow(_claimUsername, _function_1);
this.submitMutation(claim);
} else {
boolean _startsWith_2 = line.startsWith("/help");
if (_startsWith_2) {
InputOutput.println("Commands:");
InputOutput.println("/claim \t\tClaim a username");
}
}
} else {
Message _message = new Message();
final Procedure1 _function_2 = (Message it) -> {
it.setMessage(line);
};
final Message message = ObjectExtensions.operator_doubleArrow(_message, _function_2);
this.submitMutation(message);
}
}
}
};
new Thread(_function).start();
}
/**
* This is called whenever a new mutation is broadcasted. We check if we consider it valid. If it is, it gets saved and propagated further to the network
*/
@Override
public boolean isValid(final Mutation mutation) {
Object _object = mutation.getObject();
if ((_object instanceof ClaimUsername)) {
Object _object_1 = mutation.getObject();
final ClaimUsername claim = ((ClaimUsername) _object_1);
IState _lastState = this.getBlockchain().getMainBranch().getLastState();
final ChatState state = ((ChatState) _lastState);
boolean _contains = state.getUsernames().values().contains(claim.getUsername());
return (!_contains);
}
return true;
}
/**
* This is called whenever a block is added to the blockchain. We retrieve its state to see wich messages were included in the block.
* See {@link ChatState} for more
*/
@Override
public void onBranchExpanded() {
IState _lastState = this.getBlockchain().getMainBranch().getLastState();
final ChatState lastState = ((ChatState) _lastState);
InputOutput.println(IterableExtensions.join(lastState.getNewChat(), "\n"));
}
/**
* Our instance of kryo. Note that this is a supplier, because we need a kryo instance for every thread that uses kryo.
* We only register our own objects.
*/
public static Kryo getChatKryo() {
Kryo _kryo = new Kryo();
final Procedure1 _function = (Kryo it) -> {
it.register(Message.class);
it.register(ClaimUsername.class);
};
return ObjectExtensions.operator_doubleArrow(_kryo, _function);
}
public static void main(final String[] args) {
int _xifexpression = (int) 0;
int _length = args.length;
boolean _greaterThan = (_length > 0);
if (_greaterThan) {
_xifexpression = Integer.parseInt(args[0]);
} else {
_xifexpression = 4567;
}
final int port = _xifexpression;
InetSocketAddress _inetSocketAddress = new InetSocketAddress("localhost", 2012);
KeyPair _generateAssymetricPair = Keys.generateAssymetricPair();
new ChatNode(Collections.unmodifiableList(CollectionLiterals.newArrayList(_inetSocketAddress)), port, _generateAssymetricPair).start();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy