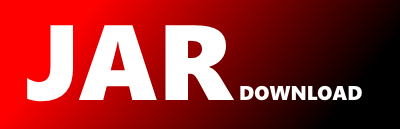
com.sitewhere.microservice.context.SiteWhereContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sitewhere-microservice Show documentation
Show all versions of sitewhere-microservice Show documentation
SiteWhere Microservice Components Library
The newest version!
/**
* Copyright © 2014-2021 The SiteWhere Authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.sitewhere.microservice.context;
import java.util.ArrayList;
import java.util.List;
import com.sitewhere.spi.SiteWhereException;
import com.sitewhere.spi.device.IDeviceAssignment;
import com.sitewhere.spi.device.event.IDeviceAlert;
import com.sitewhere.spi.device.event.IDeviceCommandInvocation;
import com.sitewhere.spi.device.event.IDeviceCommandResponse;
import com.sitewhere.spi.device.event.IDeviceEvent;
import com.sitewhere.spi.device.event.IDeviceLocation;
import com.sitewhere.spi.device.event.IDeviceMeasurement;
import com.sitewhere.spi.device.event.request.IDeviceAlertCreateRequest;
import com.sitewhere.spi.device.event.request.IDeviceLocationCreateRequest;
import com.sitewhere.spi.device.event.request.IDeviceMeasurementCreateRequest;
import com.sitewhere.spi.microservice.context.ISiteWhereContext;
/**
* Default context implementation.
*/
public class SiteWhereContext implements ISiteWhereContext {
/** Current assignment for device */
private IDeviceAssignment deviceAssignment;
/** Measurements that have not been persisted */
private List unsavedDeviceMeasurements = new ArrayList();
/** Locations that have not been persisted */
private List unsavedDeviceLocations = new ArrayList();
/** Alerts that have not been persisted */
private List unsavedDeviceAlerts = new ArrayList();
/** Measurements that have been persisted */
private List deviceMeasurements = new ArrayList();
/** Locations that have been persisted */
private List deviceLocations = new ArrayList();
/** Alerts that have been persisted */
private List deviceAlerts = new ArrayList();
/** Command invocations that have been persisted */
private List deviceCommandInvocations = new ArrayList();
/** Command responses that have been persisted */
private List deviceCommandResponses = new ArrayList();
/** Information for replying to originator */
private String replyTo;
/*
* (non-Javadoc)
*
* @see com.sitewhere.spi.ISiteWhereContext#getDeviceAssignment()
*/
public IDeviceAssignment getDeviceAssignment() {
return deviceAssignment;
}
public void setDeviceAssignment(IDeviceAssignment deviceAssignment) {
this.deviceAssignment = deviceAssignment;
}
/*
* (non-Javadoc)
*
* @see com.sitewhere.spi.ISiteWhereContext#getUnsavedDeviceMeasurements()
*/
public List getUnsavedDeviceMeasurements() {
return unsavedDeviceMeasurements;
}
public void setUnsavedDeviceMeasurements(List unsavedDeviceMeasurements) {
this.unsavedDeviceMeasurements = unsavedDeviceMeasurements;
}
/*
* (non-Javadoc)
*
* @see com.sitewhere.spi.ISiteWhereContext#getUnsavedDeviceLocations()
*/
public List getUnsavedDeviceLocations() {
return unsavedDeviceLocations;
}
public void setUnsavedDeviceLocations(List unsavedDeviceLocations) {
this.unsavedDeviceLocations = unsavedDeviceLocations;
}
/*
* (non-Javadoc)
*
* @see com.sitewhere.spi.ISiteWhereContext#getUnsavedDeviceAlerts()
*/
public List getUnsavedDeviceAlerts() {
return unsavedDeviceAlerts;
}
public void setUnsavedDeviceAlerts(List unsavedDeviceAlerts) {
this.unsavedDeviceAlerts = unsavedDeviceAlerts;
}
/*
* (non-Javadoc)
*
* @see com.sitewhere.spi.ISiteWhereContext#getDeviceMeasurements()
*/
public List getDeviceMeasurements() {
return deviceMeasurements;
}
public void setDeviceMeasurements(List deviceMeasurements) {
this.deviceMeasurements = deviceMeasurements;
}
/*
* (non-Javadoc)
*
* @see com.sitewhere.spi.ISiteWhereContext#getDeviceLocations()
*/
public List getDeviceLocations() {
return deviceLocations;
}
public void setDeviceLocations(List deviceLocations) {
this.deviceLocations = deviceLocations;
}
/*
* (non-Javadoc)
*
* @see com.sitewhere.spi.ISiteWhereContext#getDeviceAlerts()
*/
public List getDeviceAlerts() {
return deviceAlerts;
}
public void setDeviceAlerts(List deviceAlerts) {
this.deviceAlerts = deviceAlerts;
}
/*
* (non-Javadoc)
*
* @see com.sitewhere.spi.ISiteWhereContext#getDeviceCommandInvocations()
*/
public List getDeviceCommandInvocations() {
return deviceCommandInvocations;
}
public void setDeviceCommandInvocations(List deviceCommandInvocations) {
this.deviceCommandInvocations = deviceCommandInvocations;
}
/*
* (non-Javadoc)
*
* @see com.sitewhere.spi.ISiteWhereContext#getDeviceCommandResponses()
*/
public List getDeviceCommandResponses() {
return deviceCommandResponses;
}
public void setDeviceCommandResponses(List deviceCommandResponses) {
this.deviceCommandResponses = deviceCommandResponses;
}
/*
* (non-Javadoc)
*
* @see com.sitewhere.spi.ISiteWhereContext#getReplyTo()
*/
public String getReplyTo() {
return replyTo;
}
public void setReplyTo(String replyTo) {
this.replyTo = replyTo;
}
/**
* Helper function for add an arbitrary device event.
*
* @param event
* @throws SiteWhereException
*/
public void addDeviceEvent(IDeviceEvent event) throws SiteWhereException {
if (event instanceof IDeviceMeasurement) {
getDeviceMeasurements().add((IDeviceMeasurement) event);
} else if (event instanceof IDeviceLocation) {
getDeviceLocations().add((IDeviceLocation) event);
} else if (event instanceof IDeviceAlert) {
getDeviceAlerts().add((IDeviceAlert) event);
} else if (event instanceof IDeviceCommandInvocation) {
getDeviceCommandInvocations().add((IDeviceCommandInvocation) event);
} else if (event instanceof IDeviceCommandResponse) {
getDeviceCommandResponses().add((IDeviceCommandResponse) event);
} else {
throw new SiteWhereException("Context does not support event type: " + event.getClass().getName());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy