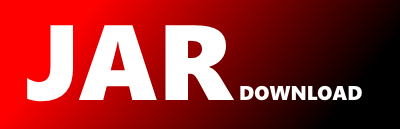
com.sitewhere.microservice.grpc.JwtServerInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sitewhere-microservice Show documentation
Show all versions of sitewhere-microservice Show documentation
SiteWhere Microservice Components Library
The newest version!
/**
* Copyright © 2014-2021 The SiteWhere Authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.sitewhere.microservice.grpc;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import com.sitewhere.spi.microservice.IFunctionIdentifier;
import com.sitewhere.spi.microservice.IMicroservice;
import com.sitewhere.spi.microservice.IMicroserviceConfiguration;
import io.grpc.BindableService;
import io.grpc.Context;
import io.grpc.Contexts;
import io.grpc.Metadata;
import io.grpc.ServerCall;
import io.grpc.ServerCall.Listener;
import io.grpc.ServerCallHandler;
import io.grpc.ServerInterceptor;
import io.grpc.Status;
/**
* Interceptor that enforces JWT authentication constraints before invoking
* service methods.
*/
public class JwtServerInterceptor implements ServerInterceptor {
/** Static logger instance */
private static Log LOGGER = LogFactory.getLog(JwtServerInterceptor.class);
/** Parent microservice */
private IMicroservice extends IFunctionIdentifier, ? extends IMicroserviceConfiguration> microservice;
/** Service implementation */
private Class extends BindableService> implementation;
public JwtServerInterceptor(
IMicroservice extends IFunctionIdentifier, ? extends IMicroserviceConfiguration> microservice,
Class extends BindableService> implementation) {
this.microservice = microservice;
this.implementation = implementation;
}
/*
* (non-Javadoc)
*
* @see io.grpc.ServerInterceptor#interceptCall(io.grpc.ServerCall,
* io.grpc.Metadata, io.grpc.ServerCallHandler)
*/
@Override
public Listener interceptCall(ServerCall call, Metadata headers,
ServerCallHandler next) {
if (headers.containsKey(GrpcKeys.JWT_KEY)) {
String jwt = headers.get(GrpcKeys.JWT_KEY);
LOGGER.trace("Server received jwt key: " + jwt);
Context ctx = Context.current().withValue(GrpcKeys.JWT_CONTEXT_KEY, jwt);
return Contexts.interceptCall(ctx, call, headers, next);
} else {
call.close(Status.UNAUTHENTICATED.withDescription("JWT not passed in metadata."), headers);
return new ServerCall.Listener() {
};
}
}
protected IMicroservice extends IFunctionIdentifier, ? extends IMicroserviceConfiguration> getMicroservice() {
return microservice;
}
protected Class extends BindableService> getImplementation() {
return implementation;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy