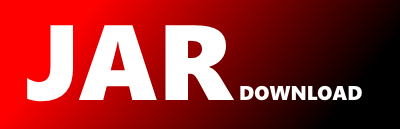
com.sitewhere.spring.handler.SearchProvidersParser Maven / Gradle / Ivy
/*
* Copyright (c) SiteWhere, LLC. All rights reserved. http://www.sitewhere.com
*
* The software in this package is published under the terms of the CPAL v1.0
* license, a copy of which has been included with this distribution in the
* LICENSE.txt file.
*/
package com.sitewhere.spring.handler;
import java.util.List;
import org.springframework.beans.factory.support.AbstractBeanDefinition;
import org.springframework.beans.factory.support.BeanDefinitionBuilder;
import org.springframework.beans.factory.support.ManagedList;
import org.springframework.beans.factory.xml.AbstractBeanDefinitionParser;
import org.springframework.beans.factory.xml.NamespaceHandler;
import org.springframework.beans.factory.xml.ParserContext;
import org.springframework.util.xml.DomUtils;
import org.w3c.dom.Attr;
import org.w3c.dom.Element;
import com.sitewhere.server.SiteWhereServerBeans;
import com.sitewhere.server.search.SearchProviderManager;
import com.sitewhere.solr.SiteWhereSolrConfiguration;
import com.sitewhere.solr.search.SolrSearchProvider;
import com.sitewhere.spring.handler.ISearchProvidersParser.Elements;
/**
* Parses configuration information for the 'search-providers' section.
*
* @author Derek
*/
public class SearchProvidersParser extends AbstractBeanDefinitionParser {
/*
* (non-Javadoc)
*
* @see org.springframework.beans.factory.xml.AbstractBeanDefinitionParser#
* parseInternal (org.w3c.dom.Element,
* org.springframework.beans.factory.xml.ParserContext)
*/
@Override
protected AbstractBeanDefinition parseInternal(Element element, ParserContext context) {
BeanDefinitionBuilder manager = BeanDefinitionBuilder.rootBeanDefinition(SearchProviderManager.class);
ManagedList> providers = parseSearchProviders(element, context);
manager.addPropertyValue("searchProviders", providers);
context.getRegistry().registerBeanDefinition(SiteWhereServerBeans.BEAN_SEARCH_PROVIDER_MANAGER,
manager.getBeanDefinition());
return null;
}
/**
* Parse the list of serach providers from nested elements.
*
* @param element
* @param context
* @return
*/
protected ManagedList> parseSearchProviders(Element element, ParserContext context) {
ManagedList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy