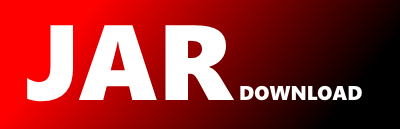
skadistats.clarity.source.PacketPosition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clarity Show documentation
Show all versions of clarity Show documentation
Clarity is an open source replay parser for Dota 2 and CSGO 1 and 2 written in Java.
package skadistats.clarity.source;
public class PacketPosition implements Comparable {
private final int tick;
private final ResetRelevantKind kind;
private final int offset;
public static PacketPosition createPacketPosition(int tick, ResetRelevantKind kind, int offset) {
if (kind != null) {
return new PacketPosition(tick, kind, offset);
}
else {
return null;
}
}
private PacketPosition(int tick, ResetRelevantKind kind, int offset) {
this.tick = tick;
this.kind = kind;
this.offset = offset;
}
public int getTick() {
return tick;
}
public ResetRelevantKind getKind() {
return kind;
}
public int getOffset() {
return offset;
}
@Override
public int compareTo(PacketPosition o) {
var r = Integer.compare(tick, o.tick);
return r != 0 ? r : kind.compareTo(o.kind);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
var that = (PacketPosition) o;
return tick == that.tick && kind == that.kind;
}
@Override
public int hashCode() {
var result = tick;
result = 31 * result + kind.hashCode();
return result;
}
@Override
public String toString() {
final var sb = new StringBuilder("PacketPosition{");
sb.append("tick=").append(tick);
sb.append(", kind=").append(kind);
sb.append(", offset=").append(offset);
sb.append('}');
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy