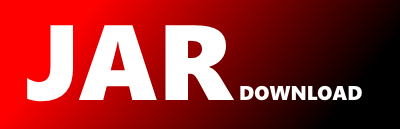
com.skillw.pouvoir.internal.feature.hologram.Hologram.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Pouvoir Show documentation
Show all versions of Pouvoir Show documentation
Bukkit Script Engine Plugin.
package com.skillw.pouvoir.internal.feature.hologram
import com.skillw.pouvoir.api.feature.hologram.IHologram
import org.bukkit.Location
import org.bukkit.entity.Player
import java.util.concurrent.ConcurrentHashMap
class Hologram private constructor(var location: Location, val content: List) : IHologram {
override val key = (location.toString().hashCode() + content.hashCode()).toString()
val viewers = ConcurrentHashSet()
private val lines = ConcurrentHashMap()
init {
content.forEachIndexed { index, line ->
lines[index] =
HologramLine(location.clone().add(0.0, (((content.size - 1) - index) * 0.3), 0.0), line, this.viewers)
}
}
constructor(location: Location, content: List, vararg viewers: Player) : this(location, content) {
this.viewers.addAll(viewers)
}
constructor(location: Location, content: List, viewers: Set) : this(location, content) {
this.viewers.addAll(viewers)
}
override fun teleport(location: Location) {
this.location = location
lines.forEach { (index, singleLine) ->
singleLine.teleport(location.clone().add(0.0, (((lines.size - 1) - index) * 0.3), 0.0))
}
}
override fun update(content: List) {
delete()
lines.clear()
content.forEachIndexed { index, line ->
lines[index] = HologramLine(
location.clone().add(0.0, (((content.size - 1) - index) * 0.3), 0.0),
line,
viewers
)
}
}
override fun delete() {
lines.forEach { it.value.delete() }
}
override fun destroy() {
lines.forEach { it.value.destroy() }
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy