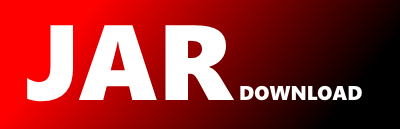
com.skillw.pouvoir.util.MapUtil.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Pouvoir Show documentation
Show all versions of Pouvoir Show documentation
Bukkit Script Engine Plugin.
package com.skillw.pouvoir.util
import com.skillw.pouvoir.api.plugin.map.BaseMap
import org.bukkit.entity.Entity
import org.bukkit.entity.Player
import java.util.*
/**
* ClassName : com.skillw.pouvoir.feature.MapUtils Created by Glom_ on
* 2021-03-28 21:59:37 Copyright 2021 user.
*/
@JvmName("addSingleKListV")
fun BaseMap>.put(key: K, value: V): BaseMap> {
if (!containsKey(key)) {
this[key] = LinkedList(listOf(value))
} else {
this[key]!!.add(value)
}
return this
}
@JvmName("addSingleKSetV")
fun BaseMap>.put(key: K, value: V): BaseMap> {
if (!containsKey(key)) {
this[key] = HashSet(listOf(value))
} else {
this[key]!!.add(value)
}
return this
}
@JvmName("addSingleKSetV2")
fun BaseMap>.put(key: K, value: V): BaseMap> {
if (!containsKey(key)) {
this[key] = LinkedHashSet(listOf(value))
} else {
this[key]!!.add(value)
}
return this
}
fun BaseMap>.put(
key1: K,
key2: Z,
value: V,
): BaseMap> {
if (!containsKey(key1)) {
val map1: BaseMap = BaseMap()
map1[key2] = value
this[key1] = map1
} else {
this[key1]!![key2] = value
}
return this
}
@JvmName("putKZListV")
fun BaseMap>>.put(
key1: K,
key2: Z,
value: V,
): BaseMap>> {
if (!containsKey(key1)) put(key1, BaseMap())
get(key1)!!.put(key2, value)
return this
}
fun BaseMap>.getAllValues(): List {
val list = ArrayList()
forEach {
list.addAll(it.value.values)
}
return list
}
fun MutableMap.putEntity(entity: Entity?) {
entity?.let {
put("entity", entity)
if (it is Player) {
put("player", it)
}
}
}
fun MutableMap.putDeep(key: String, value: Any): Any? {
var map: MutableMap? = this
var list: MutableList? = null
val keys = key.split(".")
val lastIndex = keys.lastIndex
for (i in keys.indices) {
val keyStr = keys[i]
if (i == lastIndex) {
map?.put(keyStr, value) ?: list?.set(keyStr.toInt(), value)
break
}
when (val obj = map?.get(keyStr) ?: keyStr.toIntOrNull()?.let { list?.getOrNull(it) }) {
is Map<*, *> -> {
map = obj as MutableMap
list = null
}
is List<*> -> {
list = obj as MutableList?
map = null
}
null -> {
map?.let {
HashMap().also { newMap ->
it[keyStr] = newMap
map = newMap
}
}
list?.let {
val index = keyStr.toInt()
ArrayList().also { newList ->
it[index] = newList
list = newList
}
}
}
else -> {
return null
}
}
}
return null
}
fun MutableMap.getDeep(key: String): Any? {
var map: MutableMap? = this
var list: MutableList? = null
val keys = key.split(".")
val lastIndex = keys.lastIndex
for (i in keys.indices) {
val keyStr = keys[i]
val obj = map?.get(keyStr) ?: keyStr.toIntOrNull()?.let { list?.getOrNull(it) }
if (i == lastIndex) return obj
when (obj) {
is Map<*, *> -> {
map = obj as MutableMap
list = null
}
is List<*> -> {
list = obj as MutableList?
map = null
}
else -> {
return null
}
}
}
return null
}
internal fun T.clone(): Any {
return when (this) {
is Map<*, *> -> {
val map = HashMap()
forEach { (key, value) ->
key ?: return@forEach
value ?: return@forEach
map[key.toString()] = value.clone()
}
map
}
is List<*> -> {
val list = ArrayList()
mapNotNull { it }.forEach {
list.add(it.clone())
}
list
}
else -> this
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy