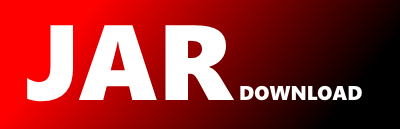
com.skillw.pouvoir.util.read.StrTrie Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Pouvoir Show documentation
Show all versions of Pouvoir Show documentation
Bukkit Script Engine Plugin.
package com.skillw.pouvoir.util.read;
import org.jetbrains.annotations.NotNull;
import java.util.Arrays;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
public class StrTrie implements Parser {
final Char c;
private final HashMap> children = new HashMap<>();
T target = null;
public StrTrie() {
this.c = new Char(' ');
}
public StrTrie(Char c) {
this.c = c;
}
@Override
public int hashCode() {
return this.c.c;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof StrTrie)) {
return false;
}
StrTrie> node = (StrTrie>) o;
return this.c == (node.c);
}
public void put(String name, T target) {
StrTrie node = this;
int index = 0;
while (index < name.length()) {
char ch = name.charAt(index++);
Char c = new Char(ch);
node = node.children.computeIfAbsent(c, (cc) -> new StrTrie<>(c));
}
node.target = target;
}
@NotNull
@Override
public Result parse(String text) {
StrTrie node = this;
int index = 0;
int start = -1;
while (index < text.length()) {
Char c = new Char(text.charAt(index++));
StrTrie next = node.children.get(c);
if (next != null) {
if (start == -1) {
start = index - 1;
}
node = next;
if (node.target != null && (index == text.length() || !node.children.containsKey(new Char(text.charAt(index))))) {
return new Result<>(this, text).setResult(node.target).setRange(start, index);
}
} else {
start = -1;
}
}
return new Result<>();
}
public Map serialize() {
HashMap map = new LinkedHashMap<>();
map.put("char", this.c.c);
map.put("children", Arrays.asList(this.children.values().stream().map(StrTrie::serialize).toArray()));
map.put("target", (this.target == null) ? "null" : this.target.toString());
return map;
}
@Override
public String toString() {
return this.serialize().toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy