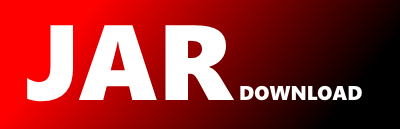
com.sksamuel.avro4s.SchemaFor.scala Maven / Gradle / Ivy
package com.sksamuel.avro4s
import com.sksamuel.avro4s.avroutils.SchemaHelper
import com.sksamuel.avro4s.schemas.{ByteIterableSchemas, CollectionSchemas, EitherSchemas, EnumSchemas, MagnoliaDerivedSchemas, OptionSchemas, PrimitiveSchemas, StringSchemas, TemporalSchemas, TupleSchemas}
import org.apache.avro.util.Utf8
import org.apache.avro.{LogicalType, LogicalTypes, Schema, SchemaBuilder}
import java.nio.ByteBuffer
import java.sql.Timestamp
import java.time.{Instant, LocalDate, LocalDateTime, OffsetDateTime}
import java.util.{Date, UUID}
/**
* A [[SchemaFor]] generates an Avro Schema for a Scala or Java type.
*
* For example, a SchemaFor[String] could return a schema of type Schema.Type.STRING, and
* a SchemaFor[Int] could return an schema of type Schema.Type.INT
*/
trait SchemaFor[T] {
self =>
/**
* Returns the avro [[Schema]] generated by this typeclass, applying the given [[FieldMapper]]
* to each field of record types.
*/
def schema: Schema
/**
* Applies a [[FieldMapper]] to this typeclass, so that the returned schema has any
* fields transformed by the mapper.
*/
final def withFieldMapper(mapper: FieldMapper): SchemaFor[T] = new SchemaFor[T] {
lazy val mapped = SchemaHelper.mapNames(self.schema, mapper)
override def schema = mapped
}
/**
* Changes the type of this SchemaFor to the desired type `U` without any other modifications.
*
* @tparam U new type for SchemaFor.
*/
final def forType[U]: SchemaFor[U] = map[U](identity)
/**
* Creates a SchemaFor[U] by applying a function Schema => Schema
* to the schema generated by this instance.
*/
final def map[U](fn: Schema => Schema): SchemaFor[U] = {
return new SchemaFor[U] {
override def schema = fn(self.schema)
}
}
}
object SchemaFor
extends PrimitiveSchemas
with BigDecimalSchemas
with ByteIterableSchemas
with CollectionSchemas
with EitherSchemas
with EnumSchemas
with OptionSchemas
with StringSchemas
with TemporalSchemas
with TupleSchemas
with MagnoliaDerivedSchemas {
/**
* Returns a [[SchemaFor]] that returns the given schema.
*/
def apply[T](s: Schema): SchemaFor[T] = new SchemaFor[T] {
override def schema: Schema = s
}
def from[T](f: => Schema): SchemaFor[T] = new SchemaFor[T] {
override def schema: Schema = f
}
def apply[T](using schemaFor: SchemaFor[T]): SchemaFor[T] = schemaFor
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy