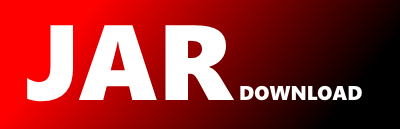
com.slickqa.junit.testrunner.testplan.TestplanFile Maven / Gradle / Ivy
package com.slickqa.junit.testrunner.testplan;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.dataformat.yaml.YAMLFactory;
import com.slickqa.junit.testrunner.Configuration;
import com.slickqa.junit.testrunner.run.TestInformationCollectingExtension;
import com.slickqa.junit.testrunner.output.TestcaseInfo;
import org.junit.platform.engine.DiscoverySelector;
import org.junit.platform.launcher.Launcher;
import org.junit.platform.launcher.LauncherDiscoveryRequest;
import org.junit.platform.launcher.core.LauncherDiscoveryRequestBuilder;
import org.junit.platform.launcher.core.LauncherFactory;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class TestplanFile {
private String name;
private String description;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy