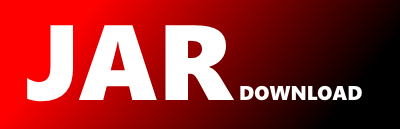
com.slimgears.apt.data.AnnotationValueInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apt-utils Show documentation
Show all versions of apt-utils Show documentation
General purpose utils / module: apt-utils
package com.slimgears.apt.data;
import com.google.auto.common.AnnotationMirrors;
import com.google.auto.common.MoreElements;
import com.google.auto.common.MoreTypes;
import com.google.auto.value.AutoOneOf;
import com.google.auto.value.AutoValue;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.slimgears.apt.util.ImportTracker;
import com.slimgears.util.stream.Optionals;
import javax.lang.model.element.AnnotationMirror;
import javax.lang.model.element.AnnotationValue;
import javax.lang.model.type.TypeMirror;
import java.util.Optional;
import java.util.stream.Collectors;
import java.util.stream.Stream;
@AutoValue
public abstract class AnnotationValueInfo implements HasName, HasType {
public enum Kind {
Primitive,
Array,
Type,
Annotation
}
@AutoOneOf(Kind.class)
public static abstract class Value {
public abstract Kind kind();
public abstract Object primitive();
public abstract AnnotationInfo annotation();
public abstract TypeInfo type();
public abstract ImmutableList array();
public String asString() {
if (kind() == Kind.Annotation) {
return annotation().asString();
} else if (kind() == Kind.Type) {
return ImportTracker.useType(TypeInfo.of(type().erasureName())) + ".class";
} else if (kind() == Kind.Array) {
ImmutableList items = array();
return items.size() != 1
? items.stream().map(Value::asString).collect(Collectors.joining(", ", "{", "}"))
: items.get(0).asString();
} else {
Object primitive = primitive();
if (primitive instanceof String) {
return '"' + primitive.toString() + '"';
} else {
return primitive.toString();
}
}
}
public static Value ofType(TypeInfo typeInfo) {
return AutoOneOf_AnnotationValueInfo_Value.type(typeInfo);
}
public static Value ofPrimitive(Object primitive) {
return AutoOneOf_AnnotationValueInfo_Value.primitive(primitive);
}
public static Value ofArray(Value... array) {
return AutoOneOf_AnnotationValueInfo_Value.array(ImmutableList.copyOf(array));
}
public static Value ofAnnotation(AnnotationInfo annotation) {
return AutoOneOf_AnnotationValueInfo_Value.annotation(annotation);
}
}
public abstract Value value();
public boolean isPrimitive() {
return value().kind() == Kind.Primitive;
}
public boolean isAnnotation() {
return value().kind() == Kind.Annotation;
}
public boolean isType() {
return value().kind() == Kind.Type;
}
public boolean isArray() {
return value().kind() == Kind.Array;
}
public static Builder builder() {
return new AutoValue_AnnotationValueInfo.Builder();
}
public static AnnotationValueInfo of(String name, Value value) {
return builder().name(name).value(value).build();
}
public static AnnotationValueInfo of(String name, AnnotationValue value) {
Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy