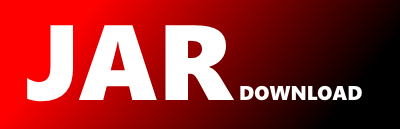
src.main.java.com.smartbear.readyapi.client.model.FileDataSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ready-api-testserver-client Show documentation
Show all versions of ready-api-testserver-client Show documentation
Java client library for creating and executing test recipes against Ready!API TestServer
package com.smartbear.readyapi.client.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
/**
* Data structure for specifying <a href=\"http://readyapi.smartbear.com/structure/sources/file/introduction\">File Data Source</a> test step.
**/
@ApiModel(description = "Data structure for specifying File Data Source test step.")
@javax.annotation.Generated(value = "class io.swagger.codegen.languages.JavaClientCodegen", date = "2016-05-24T08:38:08.699-04:00")
public class FileDataSource {
private String file = null;
private String charset = null;
private String separator = null;
private Boolean trim = false;
private Boolean quotedValues = false;
/**
* The name of the file used for the data source.
**/
public FileDataSource file(String file) {
this.file = file;
return this;
}
@ApiModelProperty(example = "null", value = "The name of the file used for the data source.")
@JsonProperty("file")
public String getFile() {
return file;
}
public void setFile(String file) {
this.file = file;
}
/**
* Character set used in the data file.
**/
public FileDataSource charset(String charset) {
this.charset = charset;
return this;
}
@ApiModelProperty(example = "null", value = "Character set used in the data file.")
@JsonProperty("charset")
public String getCharset() {
return charset;
}
public void setCharset(String charset) {
this.charset = charset;
}
/**
* Character used in the file to separate the values.
**/
public FileDataSource separator(String separator) {
this.separator = separator;
return this;
}
@ApiModelProperty(example = "null", value = "Character used in the file to separate the values.")
@JsonProperty("separator")
public String getSeparator() {
return separator;
}
public void setSeparator(String separator) {
this.separator = separator;
}
/**
* Removes excess blank spaces.
**/
public FileDataSource trim(Boolean trim) {
this.trim = trim;
return this;
}
@ApiModelProperty(example = "null", value = "Removes excess blank spaces.")
@JsonProperty("trim")
public Boolean getTrim() {
return trim;
}
public void setTrim(Boolean trim) {
this.trim = trim;
}
/**
* Set this to true if values are in quotes.
**/
public FileDataSource quotedValues(Boolean quotedValues) {
this.quotedValues = quotedValues;
return this;
}
@ApiModelProperty(example = "null", value = "Set this to true if values are in quotes.")
@JsonProperty("quotedValues")
public Boolean getQuotedValues() {
return quotedValues;
}
public void setQuotedValues(Boolean quotedValues) {
this.quotedValues = quotedValues;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
FileDataSource fileDataSource = (FileDataSource) o;
return Objects.equals(this.file, fileDataSource.file) &&
Objects.equals(this.charset, fileDataSource.charset) &&
Objects.equals(this.separator, fileDataSource.separator) &&
Objects.equals(this.trim, fileDataSource.trim) &&
Objects.equals(this.quotedValues, fileDataSource.quotedValues);
}
@Override
public int hashCode() {
return Objects.hash(file, charset, separator, trim, quotedValues);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class FileDataSource {\n");
sb.append(" file: ").append(toIndentedString(file)).append("\n");
sb.append(" charset: ").append(toIndentedString(charset)).append("\n");
sb.append(" separator: ").append(toIndentedString(separator)).append("\n");
sb.append(" trim: ").append(toIndentedString(trim)).append("\n");
sb.append(" quotedValues: ").append(toIndentedString(quotedValues)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy