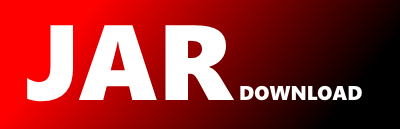
src.main.java.com.smartbear.readyapi.client.model.HarEntry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ready-api-testserver-client Show documentation
Show all versions of ready-api-testserver-client Show documentation
Java client library for creating and executing test recipes against Ready!API TestServer
package com.smartbear.readyapi.client.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.smartbear.readyapi.client.model.HarCache;
import com.smartbear.readyapi.client.model.HarRequest;
import com.smartbear.readyapi.client.model.HarResponse;
import com.smartbear.readyapi.client.model.HarTimings;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
@javax.annotation.Generated(value = "class io.swagger.codegen.languages.JavaClientCodegen", date = "2016-05-24T08:38:08.699-04:00")
public class HarEntry {
private String pageref = null;
private String startedDateTime = null;
private Long time = null;
private HarRequest request = null;
private HarResponse response = null;
private HarCache cache = null;
private HarTimings timings = null;
private String serverIPAddress = null;
private String connection = null;
private String comment = null;
/**
**/
public HarEntry pageref(String pageref) {
this.pageref = pageref;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("pageref")
public String getPageref() {
return pageref;
}
public void setPageref(String pageref) {
this.pageref = pageref;
}
/**
**/
public HarEntry startedDateTime(String startedDateTime) {
this.startedDateTime = startedDateTime;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("startedDateTime")
public String getStartedDateTime() {
return startedDateTime;
}
public void setStartedDateTime(String startedDateTime) {
this.startedDateTime = startedDateTime;
}
/**
**/
public HarEntry time(Long time) {
this.time = time;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("time")
public Long getTime() {
return time;
}
public void setTime(Long time) {
this.time = time;
}
/**
**/
public HarEntry request(HarRequest request) {
this.request = request;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("request")
public HarRequest getRequest() {
return request;
}
public void setRequest(HarRequest request) {
this.request = request;
}
/**
**/
public HarEntry response(HarResponse response) {
this.response = response;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("response")
public HarResponse getResponse() {
return response;
}
public void setResponse(HarResponse response) {
this.response = response;
}
/**
**/
public HarEntry cache(HarCache cache) {
this.cache = cache;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("cache")
public HarCache getCache() {
return cache;
}
public void setCache(HarCache cache) {
this.cache = cache;
}
/**
**/
public HarEntry timings(HarTimings timings) {
this.timings = timings;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("timings")
public HarTimings getTimings() {
return timings;
}
public void setTimings(HarTimings timings) {
this.timings = timings;
}
/**
**/
public HarEntry serverIPAddress(String serverIPAddress) {
this.serverIPAddress = serverIPAddress;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("serverIPAddress")
public String getServerIPAddress() {
return serverIPAddress;
}
public void setServerIPAddress(String serverIPAddress) {
this.serverIPAddress = serverIPAddress;
}
/**
**/
public HarEntry connection(String connection) {
this.connection = connection;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("connection")
public String getConnection() {
return connection;
}
public void setConnection(String connection) {
this.connection = connection;
}
/**
**/
public HarEntry comment(String comment) {
this.comment = comment;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("comment")
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
HarEntry harEntry = (HarEntry) o;
return Objects.equals(this.pageref, harEntry.pageref) &&
Objects.equals(this.startedDateTime, harEntry.startedDateTime) &&
Objects.equals(this.time, harEntry.time) &&
Objects.equals(this.request, harEntry.request) &&
Objects.equals(this.response, harEntry.response) &&
Objects.equals(this.cache, harEntry.cache) &&
Objects.equals(this.timings, harEntry.timings) &&
Objects.equals(this.serverIPAddress, harEntry.serverIPAddress) &&
Objects.equals(this.connection, harEntry.connection) &&
Objects.equals(this.comment, harEntry.comment);
}
@Override
public int hashCode() {
return Objects.hash(pageref, startedDateTime, time, request, response, cache, timings, serverIPAddress, connection, comment);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class HarEntry {\n");
sb.append(" pageref: ").append(toIndentedString(pageref)).append("\n");
sb.append(" startedDateTime: ").append(toIndentedString(startedDateTime)).append("\n");
sb.append(" time: ").append(toIndentedString(time)).append("\n");
sb.append(" request: ").append(toIndentedString(request)).append("\n");
sb.append(" response: ").append(toIndentedString(response)).append("\n");
sb.append(" cache: ").append(toIndentedString(cache)).append("\n");
sb.append(" timings: ").append(toIndentedString(timings)).append("\n");
sb.append(" serverIPAddress: ").append(toIndentedString(serverIPAddress)).append("\n");
sb.append(" connection: ").append(toIndentedString(connection)).append("\n");
sb.append(" comment: ").append(toIndentedString(comment)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy