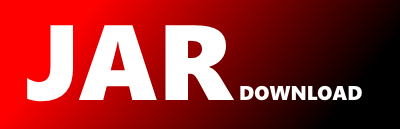
src.main.java.com.smartbear.readyapi.client.model.PropertyTransferTarget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ready-api-testserver-client Show documentation
Show all versions of ready-api-testserver-client Show documentation
Java client library for creating and executing test recipes against Ready!API TestServer
package com.smartbear.readyapi.client.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
/**
* Data structure for specifying the target of the property transfer.
**/
@ApiModel(description = "Data structure for specifying the target of the property transfer.")
@javax.annotation.Generated(value = "class io.swagger.codegen.languages.JavaClientCodegen", date = "2016-07-21T13:20:07.368+02:00")
public class PropertyTransferTarget {
private String targetName = null;
private String property = null;
private String pathLanguage = null;
private String path = null;
/**
* The full name of the target test step.
**/
public PropertyTransferTarget targetName(String targetName) {
this.targetName = targetName;
return this;
}
@ApiModelProperty(example = "null", value = "The full name of the target test step.")
@JsonProperty("targetName")
public String getTargetName() {
return targetName;
}
public void setTargetName(String targetName) {
this.targetName = targetName;
}
/**
* Property name to transfer the values to.
**/
public PropertyTransferTarget property(String property) {
this.property = property;
return this;
}
@ApiModelProperty(example = "null", value = "Property name to transfer the values to.")
@JsonProperty("property")
public String getProperty() {
return property;
}
public void setProperty(String property) {
this.property = property;
}
/**
* The language used to specify the path expression. Possible values: - XPath
- XQuery
- JSONPath
**/
public PropertyTransferTarget pathLanguage(String pathLanguage) {
this.pathLanguage = pathLanguage;
return this;
}
@ApiModelProperty(example = "null", value = "The language used to specify the path expression. Possible values: - XPath
- XQuery
- JSONPath
")
@JsonProperty("pathLanguage")
public String getPathLanguage() {
return pathLanguage;
}
public void setPathLanguage(String pathLanguage) {
this.pathLanguage = pathLanguage;
}
/**
* The expression in the specified language that specifies the exact element to which the data is be written.
**/
public PropertyTransferTarget path(String path) {
this.path = path;
return this;
}
@ApiModelProperty(example = "null", value = "The expression in the specified language that specifies the exact element to which the data is be written.")
@JsonProperty("path")
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PropertyTransferTarget propertyTransferTarget = (PropertyTransferTarget) o;
return Objects.equals(this.targetName, propertyTransferTarget.targetName) &&
Objects.equals(this.property, propertyTransferTarget.property) &&
Objects.equals(this.pathLanguage, propertyTransferTarget.pathLanguage) &&
Objects.equals(this.path, propertyTransferTarget.path);
}
@Override
public int hashCode() {
return Objects.hash(targetName, property, pathLanguage, path);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PropertyTransferTarget {\n");
sb.append(" targetName: ").append(toIndentedString(targetName)).append("\n");
sb.append(" property: ").append(toIndentedString(property)).append("\n");
sb.append(" pathLanguage: ").append(toIndentedString(pathLanguage)).append("\n");
sb.append(" path: ").append(toIndentedString(path)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy