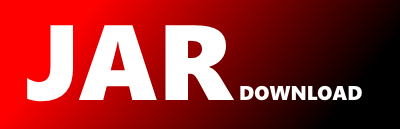
com.smartbear.visualtest.DriverManager Maven / Gradle / Ivy
The newest version!
package com.smartbear.visualtest;
import com.smartbear.visualtest.api.ApiBuilder;
import com.smartbear.visualtest.models.*;
import com.smartbear.visualtest.models.api.DeviceInfoApiResult;
import com.smartbear.visualtest.util.Utils;
import kotlin.Pair;
import lombok.AccessLevel;
import lombok.Getter;
import lombok.Setter;
import org.apache.commons.lang3.time.StopWatch;
import org.openqa.selenium.Capabilities;
import java.io.IOException;
import java.util.List;
import java.util.Map;
import java.util.concurrent.TimeUnit;
@Getter
@Setter
public abstract class DriverManager {
public Object driver;
public Boolean debug = false;
public String debugDir;
public UserAgentInfo userAgentInfo;
public Capabilities driverCapabilities;
public DeviceType deviceType;
public ApiBuilder apiBuilder;
public DeviceInfoApiResult deviceInfo;
public PageDimensions pageDimensions;
@Setter(AccessLevel.NONE)
protected Double MAX_TIME_MIN;
protected Double DEFAULT_MAX_TIME_MIN = 3.5;
protected Double DEFAULT_MAX_IMAGE_SIZE_MB = 20.0;
protected StopWatch fullpageStopWatch;
protected Long approxDiskSize;
public String ignoredElements;
public Boolean headless;
public String domCaptureInfo;
public Object wcagTags;
/**
* The function checks if the driver argument is null and throws an exception if it is.
*
* @param driver The "driver" parameter is an object that represents a web browser driver. It is
* used in the "checkBrowser" method to check if the driver object is null or not. If the driver
* object is null, an error message is logged and an exception is thrown.
*/
public void checkBrowser(Object driver) {
try {
if (driver == null) {
Utils.logger.log(Utils.ERROR, "Driver is null");
throw new Exception("Driver argument is required");
}
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
/**
* This function sets time limit for making screenshot.
*
* @param maxTimeMin Object that should have Double type and is set as maxTimeMin of `Browser`
* @throws Exception `Exception` is thrown if maxTimeMin is not between 0 and 10 minutes or not a `Double` type.
*/
protected void setMAX_TIME_MIN(Object maxTimeMin) throws Exception {
if (!(maxTimeMin instanceof Double)) {
throw new Exception("MAX_TIME_MIN must be an double");
}
if ((Double) maxTimeMin < 0 || (Double) maxTimeMin > 10) {
throw new Exception("MAX_TIME_MIN must be between 0 and 10 minutes");
}
this.MAX_TIME_MIN = (Double) maxTimeMin;
}
/**
* This function checks if any of the limits is reached
*
* @return Pair of Boolean if limit reached and String of limit reached
*/
protected Pair checkLimits() {
if (this.fullpageStopWatch.getTime(TimeUnit.SECONDS) / 60.0 > MAX_TIME_MIN) {
return new Pair<>(true, "MAX_TIME_MIN");
}
if (this.approxDiskSize > DEFAULT_MAX_IMAGE_SIZE_MB * (1024 * 1024)) {
return new Pair<>(true, "MAX_IMAGE_SIZE");
}
return new Pair<>(false, "");
}
abstract protected ScreenshotResult takeViewportScreenshot(Map options);
abstract protected ScreenshotResult takeFullPageScreenshot(Map options);
abstract protected ScreenshotResult takeElementScreenshot(Map options);
public List getAccessibilityViolationRules() throws Exception {
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy