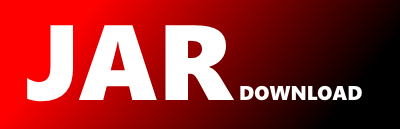
com.smartdevicelink.proxy.rpc.AddCommand Maven / Gradle / Ivy
/*
* Copyright (c) 2017 - 2019, SmartDeviceLink Consortium, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following
* disclaimer in the documentation and/or other materials provided with the
* distribution.
*
* Neither the name of the SmartDeviceLink Consortium, Inc. nor the names of its
* contributors may be used to endorse or promote products derived from this
* software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package com.smartdevicelink.proxy.rpc;
import androidx.annotation.NonNull;
import com.smartdevicelink.protocol.enums.FunctionID;
import com.smartdevicelink.proxy.RPCRequest;
import java.util.Hashtable;
import java.util.List;
/**
* This class will add a command to the application's Command Menu
*
*
* Note: A command will be added to the end of the list of elements in
* the Command Menu under the following conditions:
*
*
* - When a Command is added with no MenuParams value provided
* - When a MenuParams value is provided with a MenuParam.position value
* greater than or equal to the number of menu items currently defined in the
* menu specified by the MenuParam.parentID value
*
*
*
* The set of choices which the application builds using AddCommand can be a
* mixture of:
*
*
* - Choices having only VR synonym definitions, but no MenuParams definitions
*
* - Choices having only MenuParams definitions, but no VR synonym definitions
*
* - Choices having both MenuParams and VR synonym definitions
*
*
* HMILevel needs to be FULL, LIMITED or BACKGROUND
*
* Parameter List
*
*
* Param Name
* Type
* Description
* Req.
* Notes
* Version Available
*
*
* cmdID
* Integer
* unique ID of the command to add
* Y
* minvalue:0; maxvalue:2000000000
* SmartDeviceLink 1.0
*
* menuParams
* ButtonName
* Name of the button to unsubscribe.
* Y
*
* SmartDeviceLink 1.0
*
*
* vrCommands
* String
* An array of strings to be used as VR synonyms for this command.
If this array is provided, it may not be empty.
* N
* minsize:1; maxsize:100
* SmartDeviceLink 1.0
*
* cmdIcon
* Image
* Image struct determining whether static or dynamic icon.
If omitted on supported displays, no (or the default if applicable) icon shall be displayed.
* N
*
* SmartDeviceLink 1.0
*
*
* secondaryImage
* Image
* Optional secondary image struct for menu cell
* N
*
* SmartDeviceLink 7.1.0
*
*
* Response
Indicates that the corresponding request has failed or succeeded, if the response returns with a SUCCESS result code, this means a command was added to the Command Menu successfully.
*
* Non-default Result Codes:
INVALID_ID
DUPLICATE_NAME
*
* @see DeleteCommand
* @see AddSubMenu
* @see DeleteSubMenu
* @since SmartDeviceLink 1.0
*/
public class AddCommand extends RPCRequest {
public static final String KEY_CMD_ICON = "cmdIcon";
public static final String KEY_MENU_PARAMS = "menuParams";
public static final String KEY_CMD_ID = "cmdID";
public static final String KEY_VR_COMMANDS = "vrCommands";
public static final String KEY_SECONDARY_IMAGE = "secondaryImage";
/**
* Constructs a new AddCommand object
*/
public AddCommand() {
super(FunctionID.ADD_COMMAND.toString());
}
/**
*
* Constructs a new AddCommand object indicated by the Hashtable
* parameter
*
*
* @param hash The Hashtable to use
*/
public AddCommand(Hashtable hash) {
super(hash);
}
/**
* Constructs a new AddCommand object
*
* @param cmdID an integer object representing a Command ID Notes: Min Value: 0; Max Value: 2000000000
*/
public AddCommand(@NonNull Integer cmdID) {
this();
setCmdID(cmdID);
}
/**
*
* Returns an Integer object representing the Command ID that you want to add
*
*
* @return Integer -an integer representation a Unique Command ID
*/
public Integer getCmdID() {
return getInteger(KEY_CMD_ID);
}
/**
* Sets a Unique Command ID that identifies the command. Is returned in an
* {@linkplain OnCommand} notification to identify the command
* selected by the user
*
* @param cmdID an integer object representing a Command ID
*
* Notes: Min Value: 0; Max Value: 2000000000
*/
public AddCommand setCmdID(@NonNull Integer cmdID) {
setParameters(KEY_CMD_ID, cmdID);
return this;
}
/**
*
* Returns a MenuParams object which will defined the command and how
* it is added to the Command Menu
*
*
* @return MenuParams -a MenuParams object
*/
public MenuParams getMenuParams() {
return (MenuParams) getObject(MenuParams.class, KEY_MENU_PARAMS);
}
/**
*
* Sets Menu parameters
* If provided, this will define the command and how it is added to the
* Command Menu
* If null, commands will not be accessible through the HMI application menu
*
*
* @param menuParams a menuParams object
*/
public AddCommand setMenuParams(MenuParams menuParams) {
setParameters(KEY_MENU_PARAMS, menuParams);
return this;
}
/**
*
* Gets Voice Recognition Commands
*
*
* @return List -(List) indicating one or more VR phrases
*/
@SuppressWarnings("unchecked")
public List getVrCommands() {
return (List) getObject(String.class, KEY_VR_COMMANDS);
}
/**
*
* Sets Voice Recognition Commands
* If provided, defines one or more VR phrases the recognition of any of
* which triggers the {@linkplain OnCommand} notification with this
* cmdID
* If null, commands will not be accessible by voice commands (when the user
* hits push-to-talk)
*
*
* @param vrCommands List indicating one or more VR phrases
*
* Notes: Optional only if menuParams is provided. If
* provided, array must contain at least one non-empty (not null,
* not zero-length, not whitespace only) element
*/
public AddCommand setVrCommands(List vrCommands) {
setParameters(KEY_VR_COMMANDS, vrCommands);
return this;
}
/**
* Gets the image to be shown along with a command
*
* @return Image -an Image object
* @since SmartDeviceLink 2.0
*/
public Image getCmdIcon() {
return (Image) getObject(Image.class, KEY_CMD_ICON);
}
/**
* Sets the Image
* If provided, defines the image to be be shown along with a command
*
* @param cmdIcon an Image obj representing the Image obj shown along with a
* command
*
* Notes: If omitted on supported displays, no (or the
* default if applicable) icon will be displayed
* @since SmartDeviceLink 2.0
*/
public AddCommand setCmdIcon(Image cmdIcon) {
setParameters(KEY_CMD_ICON, cmdIcon);
return this;
}
/**
* Sets the secondaryImage.
*
* @param secondaryImage Optional secondary image struct for menu cell
* @since SmartDeviceLink 7.1.0
*/
public AddCommand setSecondaryImage(Image secondaryImage) {
setParameters(KEY_SECONDARY_IMAGE, secondaryImage);
return this;
}
/**
* Gets the secondaryImage.
*
* @return Image Optional secondary image struct for menu cell
* @since SmartDeviceLink 7.1.0
*/
public Image getSecondaryImage() {
return (Image) getObject(Image.class, KEY_SECONDARY_IMAGE);
}
}