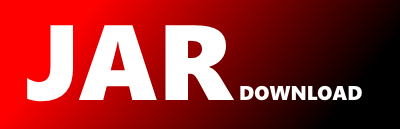
com.smartdevicelink.proxy.rpc.GPSData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdl_java_se Show documentation
Show all versions of sdl_java_se Show documentation
The app library component of SDL is meant to run on the end userâs smart-device from within SDL enabled apps, as an embedded app, or connected to the cloud. App libraries allow the apps to connect to SDL enabled head-units and hardware through bluetooth, USB, and TCP for Android, and cloud and embedded apps can connect through web sockets, Java Beans, and other custom transports. Once the library establishes a connection between the smart device and head-unit through the preferred method of transport, the two components are able to communicate using the SDL defined protocol. The app integrating this library project is then able to expose its functionality to the head-unit through text, media, and other interactive elements.
/*
* Copyright (c) 2017 - 2019, SmartDeviceLink Consortium, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following
* disclaimer in the documentation and/or other materials provided with the
* distribution.
*
* Neither the name of the SmartDeviceLink Consortium, Inc. nor the names of its
* contributors may be used to endorse or promote products derived from this
* software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package com.smartdevicelink.proxy.rpc;
import androidx.annotation.NonNull;
import com.smartdevicelink.proxy.RPCStruct;
import com.smartdevicelink.proxy.rpc.enums.CompassDirection;
import com.smartdevicelink.proxy.rpc.enums.Dimension;
import com.smartdevicelink.util.SdlDataTypeConverter;
import java.util.Hashtable;
/**
* Describes the GPS data. Not all data will be available on all car lines.
* Parameter List
*
*
* Name
* Type
* Description
* SmartDeviceLink Ver. Available
*
*
* longitudeDegrees
* Double
* Minvalue: - 180
* Maxvalue: 180
*
* SmartDeviceLink 2.0
*
*
* latitudeDegrees
* Double
* Minvalue: - 90Maxvalue: 90
*
* SmartDeviceLink 2.0
*
*
* utcYear
* Integer
* Minvalue: 2010Maxvalue: 2100
*
* SmartDeviceLink 2.0
*
*
* utcMonth
* Integer
* Minvalue: 1Maxvalue: 12
*
* SmartDeviceLink 2.0
*
*
* utcDay
* Integer
* Minvalue: 1Maxvalue: 31
*
* SmartDeviceLink 2.0
*
*
* utcHours
* Integer
* Minvalue: 0Maxvalue: 23
*
* SmartDeviceLink 2.0
*
*
* utcMinutes
* Integer
* Minvalue: 0Maxvalue: 59
*
* SmartDeviceLink 2.0
*
*
* utcSeconds
* Integer
* Minvalue: 0Maxvalue: 59
*
* SmartDeviceLink 2.0
*
*
* pdop
* Integer
* Positional Dilution of Precision. If undefined or unavailable, then value shall be set to 0.Minvalue: 0Maxvalue: 1000
*
* SmartDeviceLink 2.0
*
*
* hdop
* Integer
* Horizontal Dilution of Precision. If value is unknown, value shall be set to 0.Minvalue: 0Maxvalue: 1000
*
* SmartDeviceLink 2.0
*
*
* vdop
* Integer
* Vertical Dilution of Precision. If value is unknown, value shall be set to 0.Minvalue: 0Maxvalue: 1000
*
* SmartDeviceLink 2.0
*
*
* actual
* Boolean
* True, if coordinates are based on satellites.
* False, if based on dead reckoning
*
* SmartDeviceLink 2.0
*
*
* satellites
* Integer
* Number of satellites in view
* Minvalue: 0
* Maxvalue: 31
*
* SmartDeviceLink 2.0
*
*
* altitude
* Integer
* Altitude in meters
* Minvalue: -10000
* Maxvalue: 10000
* Note: SYNC uses Mean Sea Level for calculating GPS.
* SmartDeviceLink 2.0
*
*
* heading
* Double
* The heading. North is 0, East is 90, etc.
* Minvalue: 0
* Maxvalue: 359.99
* Resolution is 0.01
*
* SmartDeviceLink 2.0
*
*
* speed
* Integer
* The speed in KPH
* Minvalue: 0
* Maxvalue: 500
*
* SmartDeviceLink 2.0
*
*
*
* @since SmartDeviceLink 2.0
*/
public class GPSData extends RPCStruct {
public static final String KEY_LONGITUDE_DEGREES = "longitudeDegrees";
public static final String KEY_LATITUDE_DEGREES = "latitudeDegrees";
public static final String KEY_UTC_YEAR = "utcYear";
public static final String KEY_UTC_MONTH = "utcMonth";
public static final String KEY_UTC_DAY = "utcDay";
public static final String KEY_UTC_HOURS = "utcHours";
public static final String KEY_UTC_MINUTES = "utcMinutes";
public static final String KEY_UTC_SECONDS = "utcSeconds";
public static final String KEY_COMPASS_DIRECTION = "compassDirection";
public static final String KEY_PDOP = "pdop";
public static final String KEY_VDOP = "vdop";
public static final String KEY_HDOP = "hdop";
public static final String KEY_ACTUAL = "actual";
public static final String KEY_SATELLITES = "satellites";
public static final String KEY_DIMENSION = "dimension";
public static final String KEY_ALTITUDE = "altitude";
public static final String KEY_HEADING = "heading";
public static final String KEY_SPEED = "speed";
public static final String KEY_SHIFTED = "shifted";
/**
* Constructs a newly allocated GPSData object
*/
public GPSData() {
}
/**
* Constructs a newly allocated GPSData object indicated by the Hashtable parameter
*
* @param hash The Hashtable to use
*/
public GPSData(Hashtable hash) {
super(hash);
}
/**
* Constructs a newly allocated GPSData object
*/
public GPSData(@NonNull Double longitudeDegrees, @NonNull Double latitudeDegrees) {
this();
setLongitudeDegrees(longitudeDegrees);
setLatitudeDegrees(latitudeDegrees);
}
/**
* set longitude degrees
*
* @param longitudeDegrees degrees of the longitudinal position
*/
public GPSData setLongitudeDegrees(@NonNull Double longitudeDegrees) {
setValue(KEY_LONGITUDE_DEGREES, longitudeDegrees);
return this;
}
/**
* get longitude degrees
*
* @return longitude degrees
*/
public Double getLongitudeDegrees() {
Object object = getValue(KEY_LONGITUDE_DEGREES);
return SdlDataTypeConverter.objectToDouble(object);
}
/**
* set latitude degrees
*
* @param latitudeDegrees degrees of the latitudinal position
*/
public GPSData setLatitudeDegrees(@NonNull Double latitudeDegrees) {
setValue(KEY_LATITUDE_DEGREES, latitudeDegrees);
return this;
}
/**
* get latitude degrees
*
* @return latitude degrees
*/
public Double getLatitudeDegrees() {
Object object = getValue(KEY_LATITUDE_DEGREES);
return SdlDataTypeConverter.objectToDouble(object);
}
/**
* set utc year
*
* @param utcYear utc year
*/
public GPSData setUtcYear(Integer utcYear) {
setValue(KEY_UTC_YEAR, utcYear);
return this;
}
/**
* get utc year
*
* @return utc year
*/
public Integer getUtcYear() {
return getInteger(KEY_UTC_YEAR);
}
/**
* set utc month
*
* @param utcMonth utc month
*/
public GPSData setUtcMonth(Integer utcMonth) {
setValue(KEY_UTC_MONTH, utcMonth);
return this;
}
/**
* get utc month
*
* @return utc month
*/
public Integer getUtcMonth() {
return getInteger(KEY_UTC_MONTH);
}
/**
* set utc day
*
* @param utcDay utc day
*/
public GPSData setUtcDay(Integer utcDay) {
setValue(KEY_UTC_DAY, utcDay);
return this;
}
/**
* get utc day
*
* @return utc day
*/
public Integer getUtcDay() {
return getInteger(KEY_UTC_DAY);
}
/**
* set utc hours
*
* @param utcHours utc hours
*/
public GPSData setUtcHours(Integer utcHours) {
setValue(KEY_UTC_HOURS, utcHours);
return this;
}
/**
* get utc hours
*
* @return utc hours
*/
public Integer getUtcHours() {
return getInteger(KEY_UTC_HOURS);
}
/**
* set utc minutes
*
* @param utcMinutes utc minutes
*/
public GPSData setUtcMinutes(Integer utcMinutes) {
setValue(KEY_UTC_MINUTES, utcMinutes);
return this;
}
/**
* get utc minutes
*
* @return utc minutes
*/
public Integer getUtcMinutes() {
return getInteger(KEY_UTC_MINUTES);
}
/**
* set utc seconds
*
* @param utcSeconds utc seconds
*/
public GPSData setUtcSeconds(Integer utcSeconds) {
setValue(KEY_UTC_SECONDS, utcSeconds);
return this;
}
/**
* get utc seconds
*
* @return utc seconds
*/
public Integer getUtcSeconds() {
return getInteger(KEY_UTC_SECONDS);
}
public GPSData setCompassDirection(CompassDirection compassDirection) {
setValue(KEY_COMPASS_DIRECTION, compassDirection);
return this;
}
public CompassDirection getCompassDirection() {
return (CompassDirection) getObject(CompassDirection.class, KEY_COMPASS_DIRECTION);
}
/**
* set the positional dilution of precision
*
* @param pdop the positional dilution of precision
*/
public GPSData setPdop(Double pdop) {
setValue(KEY_PDOP, pdop);
return this;
}
/**
* get the positional dilution of precision
*/
public Double getPdop() {
Object object = getValue(KEY_PDOP);
return SdlDataTypeConverter.objectToDouble(object);
}
/**
* set the horizontal dilution of precision
*
* @param hdop the horizontal dilution of precision
*/
public GPSData setHdop(Double hdop) {
setValue(KEY_HDOP, hdop);
return this;
}
/**
* get the horizontal dilution of precision
*
* @return the horizontal dilution of precision
*/
public Double getHdop() {
Object object = getValue(KEY_HDOP);
return SdlDataTypeConverter.objectToDouble(object);
}
/**
* set the vertical dilution of precision
*
* @param vdop the vertical dilution of precision
*/
public GPSData setVdop(Double vdop) {
setValue(KEY_VDOP, vdop);
return this;
}
/**
* get the vertical dilution of precision
*
* @return the vertical dilution of precision
*/
public Double getVdop() {
Object object = getValue(KEY_VDOP);
return SdlDataTypeConverter.objectToDouble(object);
}
/**
* set what coordinates based on
*
* @param actual True, if coordinates are based on satellites.False, if based on dead reckoning
*/
public GPSData setActual(Boolean actual) {
setValue(KEY_ACTUAL, actual);
return this;
}
/**
* get what coordinates based on
*
* @return True, if coordinates are based on satellites.False, if based on dead reckoning
*/
public Boolean getActual() {
return getBoolean(KEY_ACTUAL);
}
/**
* set the number of satellites in view
*
* @param satellites the number of satellites in view
*/
public GPSData setSatellites(Integer satellites) {
setValue(KEY_SATELLITES, satellites);
return this;
}
/**
* get the number of satellites in view
*
* @return the number of satellites in view
*/
public Integer getSatellites() {
return getInteger(KEY_SATELLITES);
}
public GPSData setDimension(Dimension dimension) {
setValue(KEY_DIMENSION, dimension);
return this;
}
public Dimension getDimension() {
return (Dimension) getObject(Dimension.class, KEY_DIMENSION);
}
/**
* set altitude in meters
*
* @param altitude altitude in meters
*/
public GPSData setAltitude(Double altitude) {
setValue(KEY_ALTITUDE, altitude);
return this;
}
/**
* get altitude in meters
*
* @return altitude in meters
*/
public Double getAltitude() {
Object object = getValue(KEY_ALTITUDE);
return SdlDataTypeConverter.objectToDouble(object);
}
/**
* set the heading.North is 0, East is 90, etc.
*
* @param heading the heading.
*/
public GPSData setHeading(Double heading) {
setValue(KEY_HEADING, heading);
return this;
}
/**
* get the heading
*/
public Double getHeading() {
Object object = getValue(KEY_HEADING);
return SdlDataTypeConverter.objectToDouble(object);
}
/**
* set speed in KPH
*
* @param speed the speed
*/
public GPSData setSpeed(Double speed) {
setValue(KEY_SPEED, speed);
return this;
}
/**
* get the speed in KPH
*
* @return the speed in KPH
*/
public Double getSpeed() {
Object object = getValue(KEY_SPEED);
return SdlDataTypeConverter.objectToDouble(object);
}
/**
* Sets the shifted param for GPSData.
*
* @param shifted True, if GPS lat/long, time, and altitude have been purposefully shifted (requires a proprietary algorithm to unshift).
* False, if the GPS data is raw and un-shifted.
* If not provided, then value is assumed False.
*/
public GPSData setShifted(Boolean shifted) {
setValue(KEY_SHIFTED, shifted);
return this;
}
/**
* Gets the shifted param for GPSData.
*
* @return Boolean - True, if GPS lat/long, time, and altitude have been purposefully shifted (requires a proprietary algorithm to unshift).
*/
public Boolean getShifted() {
return getBoolean(KEY_SHIFTED);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy