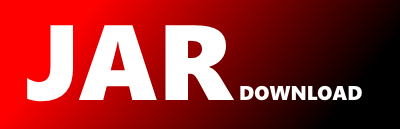
com.smartdevicelink.proxy.rpc.Grid Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdl_java_se Show documentation
Show all versions of sdl_java_se Show documentation
The app library component of SDL is meant to run on the end userâs smart-device from within SDL enabled apps, as an embedded app, or connected to the cloud. App libraries allow the apps to connect to SDL enabled head-units and hardware through bluetooth, USB, and TCP for Android, and cloud and embedded apps can connect through web sockets, Java Beans, and other custom transports. Once the library establishes a connection between the smart device and head-unit through the preferred method of transport, the two components are able to communicate using the SDL defined protocol. The app integrating this library project is then able to expose its functionality to the head-unit through text, media, and other interactive elements.
package com.smartdevicelink.proxy.rpc;
import androidx.annotation.NonNull;
import com.smartdevicelink.proxy.RPCStruct;
import java.util.Hashtable;
/**
* Struct that describes a location (origin coordinates and span) of a vehicle component (Module)
*/
public class Grid extends RPCStruct {
public static final String KEY_COL = "col";
public static final String KEY_ROW = "row";
public static final String KEY_LEVEL = "level";
public static final String KEY_COL_SPAN = "colspan";
public static final String KEY_ROW_SPAN = "rowspan";
public static final String KEY_LEVEL_SPAN = "levelspan";
public Grid() {
}
public Grid(Hashtable hash) {
super(hash);
}
/**
* Struct that describes a location (origin coordinates and span) of a vehicle component (Module)
*
* @param row Sets the row's value of this Grid
* @param column Sets the column of this Grid
*/
public Grid(@NonNull Integer row, @NonNull Integer column) {
this();
setRow(row);
setCol(column);
}
/**
* Sets the column of this Grid
*
* @param col the column to be set
*/
public Grid setCol(@NonNull Integer col) {
setValue(KEY_COL, col);
return this;
}
/**
* Get the column value of this Grid
*
* @return the column value
*/
public Integer getCol() {
return getInteger(KEY_COL);
}
/**
* Sets the row's value of this Grid
*
* @param row the row to be set
*/
public Grid setRow(@NonNull Integer row) {
setValue(KEY_ROW, row);
return this;
}
/**
* Gets the row value of this Grid
*
* @return the row value
*/
public Integer getRow() {
return getInteger(KEY_ROW);
}
/**
* Sets the level value of this Grid
*
* @param level the level to be set
*/
public Grid setLevel(Integer level) {
setValue(KEY_LEVEL, level);
return this;
}
/**
* Gets the level value of this Grid
*
* @return the level
*/
public Integer getLevel() {
return getInteger(KEY_LEVEL);
}
/**
* Sets the column span of this Grid
*
* @param span the span to be set
*/
public Grid setColSpan(Integer span) {
setValue(KEY_COL_SPAN, span);
return this;
}
/**
* Gets the column span of this Grid
*
* @return the column span
*/
public Integer getColSpan() {
return getInteger(KEY_COL_SPAN);
}
/**
* Sets the row span of this Grid
*
* @param span the span to be set
*/
public Grid setRowSpan(Integer span) {
setValue(KEY_ROW_SPAN, span);
return this;
}
/**
* Gets the row span of this Grid
*
* @return the row span
*/
public Integer getRowSpan() {
return getInteger(KEY_ROW_SPAN);
}
/**
* Sets the level span of this Grid
*
* @param span the span to be set
*/
public Grid setLevelSpan(Integer span) {
setValue(KEY_LEVEL_SPAN, span);
return this;
}
/**
* Gets the level span of this Grid
*
* @return the level span
*/
public Integer getLevelSpan() {
return getInteger(KEY_LEVEL_SPAN);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy