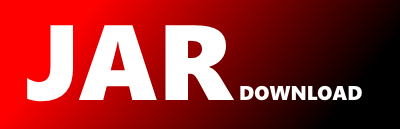
com.smartdevicelink.proxy.rpc.SeatLocationCapability Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdl_java_se Show documentation
Show all versions of sdl_java_se Show documentation
The app library component of SDL is meant to run on the end userâs smart-device from within SDL enabled apps, as an embedded app, or connected to the cloud. App libraries allow the apps to connect to SDL enabled head-units and hardware through bluetooth, USB, and TCP for Android, and cloud and embedded apps can connect through web sockets, Java Beans, and other custom transports. Once the library establishes a connection between the smart device and head-unit through the preferred method of transport, the two components are able to communicate using the SDL defined protocol. The app integrating this library project is then able to expose its functionality to the head-unit through text, media, and other interactive elements.
package com.smartdevicelink.proxy.rpc;
import com.smartdevicelink.proxy.RPCStruct;
import java.util.Hashtable;
import java.util.List;
public class SeatLocationCapability extends RPCStruct {
public static final String KEY_ROWS = "rows";
public static final String KEY_COLS = "columns";
public static final String KEY_LEVELS = "levels";
public static final String KEY_SEATS = "seats";
public SeatLocationCapability() {
}
public SeatLocationCapability(Hashtable hash) {
super(hash);
}
/**
* Sets the seat rows for this capability
*
* @param rows rows to be set
*/
public SeatLocationCapability setRows(Integer rows) {
setValue(KEY_ROWS, rows);
return this;
}
/**
* Gets the seat rows of this capability
*
* @return the seat rows
*/
public Integer getRows() {
return getInteger(KEY_ROWS);
}
/**
* Sets the seat columns for this capability
*
* @param cols the seat columns to be set
*/
public SeatLocationCapability setCols(Integer cols) {
setValue(KEY_COLS, cols);
return this;
}
/**
* Gets the seat columns of this capability
*
* @return the seat columns
*/
public Integer getCols() {
return getInteger(KEY_COLS);
}
/**
* Sets the levels for this capability
*
* @param levels the levels to be set
*/
public SeatLocationCapability setLevels(Integer levels) {
setValue(KEY_LEVELS, levels);
return this;
}
/**
* Gets the seat levels of this capability
*
* @return the seat levels
*/
public Integer getLevels() {
return getInteger(KEY_LEVELS);
}
/**
* Sets the seat locations for this capability
*
* @param locations the locations to be set
*/
public SeatLocationCapability setSeats(List locations) {
setValue(KEY_SEATS, locations);
return this;
}
/**
* Gets the seat locations of this capability
*
* @return the seat locations
* @deprecated use {@link #getSeats()} instead.
*/
@SuppressWarnings("unchecked")
@Deprecated
public List getSeatLocations() {
return getSeats();
}
/**
* Gets the seat locations of this capability
*
* @return the seat locations
*/
public List getSeats() {
return (List) getObject(SeatLocation.class, KEY_SEATS);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy