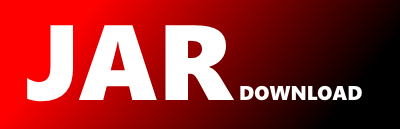
com.smartdevicelink.proxy.rpc.TouchEvent Maven / Gradle / Ivy
Show all versions of sdl_java_se Show documentation
/*
* Copyright (c) 2017 - 2019, SmartDeviceLink Consortium, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following
* disclaimer in the documentation and/or other materials provided with the
* distribution.
*
* Neither the name of the SmartDeviceLink Consortium, Inc. nor the names of its
* contributors may be used to endorse or promote products derived from this
* software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package com.smartdevicelink.proxy.rpc;
import androidx.annotation.NonNull;
import com.smartdevicelink.proxy.RPCStruct;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.List;
/**
* For touchscreen interactions, the mode of how the choices are presented.
*
* Parameter List
*
*
*
* Name
* Type
* Description
* Reg.
* Notes
* Version
*
*
* id
* Integer
* A touch's unique identifier. The application can track the current touch events by id. If a touch event has type begin, the id should be added to the set of touches. If a touch event has type end, the id should be removed from the set of touches.
* N
* Min Value: 0; Max Value: 9
* SmartDeviceLink 3.0
*
*
* ts
* Float
* The time that the touch was recorded. This number can the time since the beginning of the session or something else as long as the units are in milliseconds.
* The timestamp is used to determined the rate of change of position of a touch.
The application also uses the time to verify whether two touches,with different ids, are part of a single action by the user.
* If there is only a single timestamp in this array,
it is the same for every coordinate in the coordinates array.
* Y
* minvalue="0" maxvalue="5000000000" minsize="1" maxsize="1000"
* SmartDeviceLink 3.0
*
*
* c
* Integer
* The coordinates of the screen area where the touch event occurred.
* Y
*
* SmartDeviceLink 3.0
*
*
*
* @since SmartDeviceLink 3.0
*
* @see SoftButtonCapabilities
* @see ButtonCapabilities
* @see OnButtonPress
*/
public class TouchEvent extends RPCStruct {
public static final String KEY_ID = "id";
public static final String KEY_TS = "ts";
public static final String KEY_C = "c";
public TouchEvent() {
}
/**
*
Constructs a new TouchEvent object indicated by the Hashtable parameter
*
* @param hash The Hashtable to use
*/
public TouchEvent(Hashtable hash) {
super(hash);
}
/**
* Constructs a new TouchEvent object
*
* @param id A touch's unique identifier.
* @param ts The time that the touch was recorded.
* @param c The coordinates of the screen area where the touch event occurred.
*/
public TouchEvent(@NonNull Integer id, @NonNull List ts, @NonNull List c) {
this();
setId(id);
setTimestamps(ts);
setTouchCoordinates(c);
}
public TouchEvent setId(@NonNull Integer id) {
setValue(KEY_ID, id);
return this;
}
public Integer getId() {
return getInteger(KEY_ID);
}
/**
* Use getTimestamps
*
* @return
* @deprecated in 4.0.2. Use {@link #getTimestamps()}()} instead.
*/
@Deprecated
public List getTs() {
return getTimestamps();
}
@SuppressWarnings("unchecked")
public List getTimestamps() {
if (getValue(KEY_TS) instanceof List>) {
List> list = (List>) getValue(KEY_TS);
if (list != null && list.size() > 0) {
Object obj = list.get(0);
if (obj instanceof Integer) { //Backwards case
int size = list.size();
List listOfInt = (List) list;
List listOfLongs = new ArrayList<>(size);
for (int i = 0; i < size; i++) {
listOfLongs.add(listOfInt.get(i).longValue());
}
return listOfLongs;
} else if (obj instanceof Long) {
return (List) list;
}
}
}
return null;
}
public TouchEvent setTimestamps(@NonNull List ts) {
setValue(KEY_TS, ts);
return this;
}
/**
* Use setTimestamps.
*
* @param ts
* @deprecated in 4.0.2. Use {@link #setTimestamps(List)} instead.
*/
@Deprecated
public TouchEvent setTs(List ts) {
setTimestamps(ts);
return this;
}
/**
* Use getTouchCoordinates
*
* @return
* @deprecated in 4.0.2. Use {@link #getTouchCoordinates()} instead.
*/
@Deprecated
public List getC() {
return getTouchCoordinates();
}
@SuppressWarnings("unchecked")
public List getTouchCoordinates() {
return (List) getObject(TouchCoord.class, KEY_C);
}
/**
* Use setTouchCoordinates
*
* @return
* @deprecated in 4.0.2. Use {@link #setTouchCoordinates(List)} instead.
*/
@Deprecated
public TouchEvent setC(List c) {
setTouchCoordinates(c);
return this;
}
public TouchEvent setTouchCoordinates(@NonNull List c) {
setValue(KEY_C, c);
return this;
}
}