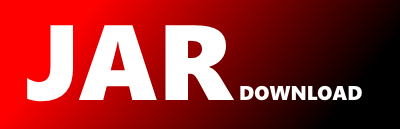
com.smartling.maven.plugins.smartlingapi.mojo.GetFilesMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartling-api-maven-plugin Show documentation
Show all versions of smartling-api-maven-plugin Show documentation
Maven plugin for accessing Smartling API.
The newest version!
package com.smartling.maven.plugins.smartlingapi.mojo;
import static org.apache.commons.io.FilenameUtils.normalize;
import java.io.File;
import java.io.IOException;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.apache.commons.io.FileUtils;
import org.apache.maven.model.Resource;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import com.google.common.base.Nullable;
import com.google.common.base.Predicate;
import com.google.common.collect.Iterables;
import com.smartling.api.sdk.dto.file.FileStatus;
import com.smartling.api.sdk.dto.file.StringResponse;
import com.smartling.api.sdk.dto.project.ProjectLocale;
import com.smartling.api.sdk.exceptions.ApiException;
import com.smartling.api.sdk.file.FileListSearchParams;
import com.smartling.api.sdk.file.RetrievalType;
import com.smartling.maven.plugins.smartlingapi.SmartlingApiMavenPluginUtils;
/**
* Downloads translated files from Smartling TMS.
*
* @version $Id$
* @since 1.0.0
*/
@Mojo(name = "getFiles", defaultPhase = LifecyclePhase.GENERATE_RESOURCES, requiresOnline = true, threadSafe = true)
class GetFilesMojo extends AbstractSmartlingFileApiMojo
{
private static final String DOWNLOAD_FILES_BY_FILEURIS_INFO_MESSAGE =
SmartlingApiMavenPluginUtils.SMARTLING_API_PLUGIN_NAME + " using fileUris, %d files to download";
private static final String DOWNLOAD_FILES_BY_FILESFILTER_INFO_MESSAGE =
SmartlingApiMavenPluginUtils.SMARTLING_API_PLUGIN_NAME + " using filesFilter, %d files to download";
private static final String DOWNLOAD_FILE_INFO_MESSAGE =
SmartlingApiMavenPluginUtils.SMARTLING_API_PLUGIN_NAME + " downloading file; fileUri=%s, locale=%s, retrievalType=%s, destFileName=%s";
private static final String DOWNLOAD_FILE_FAILED_ERROR_MESSAGE =
SmartlingApiMavenPluginUtils.SMARTLING_API_PLUGIN_NAME + " failed downloading file; fileUri=%s, locale=%s";
private static final String SAVE_FILE_FAILED_ERROR_MESSAGE =
SmartlingApiMavenPluginUtils.SMARTLING_API_PLUGIN_NAME + " failed writing file; destFileName=%s";
/**
* Target directory for the downloaded files.
*/
@Parameter(defaultValue = "${project.build.directory}/generated-resources/smartling", required = true)
private String baseDirectory;
/**
* Target files filter. This parameter is ignored if fileUris
is specified.
*/
@Parameter
private FileListSearchParams filesFilter;
/**
* Target files. This parameter invalidates filesFilter
if defined.
*/
@Parameter
private Set fileUris;
/**
* Target locales. The default is "all project's locales".
*/
@Parameter
private Set locales;
/**
* Retrieval type.
*/
@Parameter(defaultValue = "PUBLISHED", required = true)
private RetrievalType retrievalType;
/**
* Sequence of uri-to-filename transformers.
* The default is a single `appendLocaleSuffix` transformer which appends locale id just before file extension (as expected by {@link java.util.ResourceBundle}).
*/
@Parameter
private List nameTransformers;
@Override
public String getGoalName()
{
return "getFiles";
}
@Override
public void doExecute() throws MojoExecutionException, MojoFailureException
{
// if target locales were not defined: get target locales list
if (locales == null)
{
List projectLocalesList = null;
try
{
projectLocalesList = downloadProjectLocalesList();
}
catch (final ApiException e)
{
handleError(String.format(DOWNLOAD_PROJECT_LOCALES_LIST_FAILED_ERROR_MESSAGE), e);
}
locales = new HashSet();
for (final ProjectLocale projectLocale : projectLocalesList)
locales.add(projectLocale.getLocale());
}
// if name transformers were not defined: initialize defaults
if (nameTransformers == null)
{
nameTransformers = Collections.singletonList(new AppendLocaleSuffix());
}
// register `baseDirectory` as a resources-directory (if not already registered)
final String normalizedBaseDirectory = normalize(baseDirectory);
final boolean baseDirectoryMavenResourceAlreadyExists = Iterables.any(getMavenProject().getBuild().getResources(), new Predicate()
{
@Override
public boolean apply(final Resource mavenResource)
{
return normalize(mavenResource.getDirectory()).equals(normalizedBaseDirectory);
}
});
if (!baseDirectoryMavenResourceAlreadyExists)
{
final Resource baseDirectoryMavenResource = new Resource();
baseDirectoryMavenResource.setDirectory(normalizedBaseDirectory);
getMavenProject().addResource(baseDirectoryMavenResource);
}
// get files
if (null != fileUris)
getFiles(fileUris, normalizedBaseDirectory);
else
getFiles(filesFilter, normalizedBaseDirectory);
}
private void getFiles(final Set fileUris, final String baseDirectory) throws MojoFailureException
{
getLog().info(String.format(DOWNLOAD_FILES_BY_FILEURIS_INFO_MESSAGE, fileUris.size()));
// TODO: parallelize
// TODO: continue with next file on exception if not failsOnError
for (final String fileUri : fileUris)
getFileForAllLocales(fileUri, baseDirectory);
}
private void getFiles(final @Nullable FileListSearchParams filesFilter, final String baseDirectory) throws MojoFailureException
{
try
{
final List filesList = downloadFilesList(filesFilter);
getLog().info(String.format(DOWNLOAD_FILES_BY_FILESFILTER_INFO_MESSAGE, filesList.size()));
// TODO: parallelize
// TODO: continue with next file on exception if not failsOnError
for (final FileStatus fileStatus : filesList)
getFileForAllLocales(fileStatus.getFileUri(), baseDirectory);
}
catch (final ApiException e)
{
handleError(String.format(DOWNLOAD_FILES_LIST_FAILED_ERROR_MESSAGE), e);
}
}
private void getFileForAllLocales(final String fileUri, final String baseDirectory) throws MojoFailureException
{
// TODO: continue with next locale on exception if not failsOnError
for (final String locale : locales)
getFile(fileUri, locale, baseDirectory);
}
private void getFile(final String fileUri, final String locale, final String baseDirectory) throws MojoFailureException
{
final String destFileNameRelativeToBase = transformName(fileUri, locale, nameTransformers);
final File destFileAbsolute = new File(baseDirectory, destFileNameRelativeToBase);
getLog().info(String.format(DOWNLOAD_FILE_INFO_MESSAGE, fileUri, locale, retrievalType.toString(), destFileNameRelativeToBase));
try
{
final StringResponse downloadFileResponse = getFileApiClientAdapter().getFile(fileUri, locale, retrievalType);
saveFile(downloadFileResponse, destFileAbsolute);
}
catch (final ApiException e)
{
handleError(String.format(DOWNLOAD_FILE_FAILED_ERROR_MESSAGE, fileUri, locale), e);
}
catch (final IOException e)
{
handleError(String.format(SAVE_FILE_FAILED_ERROR_MESSAGE, destFileNameRelativeToBase), e);
}
}
private void saveFile(final StringResponse downloadFileResponse, final File destFile) throws IOException
{
FileUtils.writeStringToFile(destFile, downloadFileResponse.getContents(), downloadFileResponse.getEncoding());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy