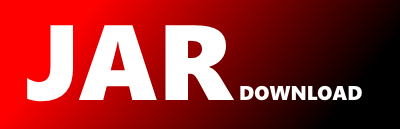
com.smartling.maven.plugins.smartlingapi.mojo.UploadFilesMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartling-api-maven-plugin Show documentation
Show all versions of smartling-api-maven-plugin Show documentation
Maven plugin for accessing Smartling API.
The newest version!
package com.smartling.maven.plugins.smartlingapi.mojo;
import static com.smartling.maven.plugins.smartlingapi.SmartlingApiMavenPluginUtils.detectFileType;
import static com.smartling.maven.plugins.smartlingapi.SmartlingApiMavenPluginUtils.unixStyleFileName;
import java.io.File;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import org.apache.commons.lang3.CharEncoding;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import com.smartling.api.sdk.dto.ApiResponse;
import com.smartling.api.sdk.dto.file.UploadFileData;
import com.smartling.api.sdk.exceptions.ApiException;
import com.smartling.api.sdk.file.FileType;
import com.smartling.api.sdk.file.parameters.FileUploadParameterBuilder;
import com.smartling.maven.plugins.smartlingapi.FileTypeDetectionException;
import com.smartling.maven.plugins.smartlingapi.SmartlingApiMavenPluginUtils;
/**
* Uploads (or re-uploads) original files to Smartling TMS.
*
* @version $Id$
* @since 1.0.0
*/
@Mojo(name = "uploadFiles", defaultPhase = LifecyclePhase.DEPLOY, requiresOnline = true, threadSafe = true)
class UploadFilesMojo extends AbstractSmartlingFileApiMojo
{
private static final String UPLOAD_FILES_INFO_MESSAGE =
SmartlingApiMavenPluginUtils.SMARTLING_API_PLUGIN_NAME + " %d files to upload";
private static final String UPLOAD_FILE_INFO_MESSAGE =
SmartlingApiMavenPluginUtils.SMARTLING_API_PLUGIN_NAME + " uploading file; srcFileName=%s, fileUri=%s, fileType=%s";
private static final String FILE_TYPE_DETECTION_FAILED_ERROR_MESSAGE =
SmartlingApiMavenPluginUtils.SMARTLING_API_PLUGIN_NAME + " failed detecting file type; srcFileName=%s";
private static final String UPLOAD_FILE_FAILED_ERROR_MESSAGE =
SmartlingApiMavenPluginUtils.SMARTLING_API_PLUGIN_NAME + " failed uploading file; srcFileName=%s, fileUri=%s";
private static final String UPLOAD_FILE_RESPONSE_INFO_MESSAGE =
SmartlingApiMavenPluginUtils.SMARTLING_API_PLUGIN_NAME + " file upload completed; srcFileName=%s, fileUri=%s. Statistics: stringCount=%d, wordCount=%d, isOverwritten=%b";
/**
* Base directory for files to be uploaded.
*/
@Parameter(defaultValue = "${project.build.resources[0].directory}", required = true)
private String baseDirectory;
/**
* A list of inclusion filters for file uploading. The processing order is "includes before excludes".
*/
@Parameter
private Set includes;
/**
* A list of exclusion filters for file uploading. The processing order is "includes before excludes".
*/
@Parameter
private Set excludes;
/**
* File type (applies to all uploaded files).
* If this parameter is omitted, then type will be inferred for every individual file by its extension.
*/
@Parameter
private FileType fileType;
/**
* File encoding (applies to all uploaded files).
*/
@Parameter(defaultValue = CharEncoding.UTF_8, required = true)
private String fileEncoding;
/**
* Automatically approve content strings after file upload.
*/
@Parameter(defaultValue = "false")
private boolean approveContent;
/**
* Callback URL to be called by Smartling after file upload.
*/
@Parameter
private String callbackUrl;
/**
* Sequence of filename-to-uri transformers. No transformers are applied by default.
*/
@Parameter
private List nameTransformers;
@Override
public String getGoalName()
{
return "uploadFiles";
}
@Override
public void doExecute() throws MojoExecutionException, MojoFailureException
{
final Collection filesRelativeToBase = scanDirectoryForInclusionsExclusions(new File(baseDirectory), includes, excludes);
final String locale = "en-US"; // TODO: make configurable; use project's default locale by default
uploadFiles(filesRelativeToBase, locale);
}
private void uploadFiles(final Collection filesRelativeToBase, final String locale) throws MojoFailureException
{
getLog().info(String.format(UPLOAD_FILES_INFO_MESSAGE, filesRelativeToBase.size()));
// TODO: parallelize
// TODO: continue with next file on exception if not failsOnError
for (final File fileRelativeToBase : filesRelativeToBase)
uploadFile(fileRelativeToBase, locale);
}
private void uploadFile(final File fileRelativeToBase, final String locale) throws MojoFailureException
{
final String fileNameRelativeToBase = unixStyleFileName(fileRelativeToBase);
final String destFileUri = transformName(fileNameRelativeToBase, locale, nameTransformers);
final File fileAbsolute = new File(baseDirectory, fileNameRelativeToBase);
try
{
final FileType fileType = getFileType(fileAbsolute);
getLog().info(String.format(UPLOAD_FILE_INFO_MESSAGE, fileNameRelativeToBase, destFileUri, fileType.toString()));
final FileUploadParameterBuilder fileUploadParameterBuilder = new FileUploadParameterBuilder()
.fileType(fileType)
.fileUri(destFileUri)
.approveContent(approveContent)
.callbackUrl(callbackUrl); // TODO: use all possible config options here
final ApiResponse uploadFileResponse = getFileApiClientAdapter().uploadFile(fileAbsolute, fileEncoding, fileUploadParameterBuilder);
checkApiResponseSuccess(uploadFileResponse);
getLog().info(String.format(UPLOAD_FILE_RESPONSE_INFO_MESSAGE, fileNameRelativeToBase, destFileUri,
uploadFileResponse.getData().getStringCount(),
uploadFileResponse.getData().getWordCount(),
uploadFileResponse.getData().isOverWritten()));
}
catch (final FileTypeDetectionException e)
{
handleError(String.format(FILE_TYPE_DETECTION_FAILED_ERROR_MESSAGE, fileNameRelativeToBase), e);
}
catch (final ApiException e)
{
handleError(String.format(UPLOAD_FILE_FAILED_ERROR_MESSAGE, fileNameRelativeToBase, destFileUri), e);
}
}
private FileType getFileType(final File file) throws FileTypeDetectionException
{
return (null != fileType) ? fileType : detectFileType(file);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy