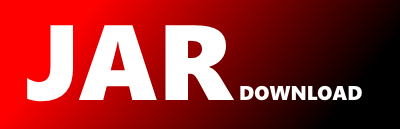
com.smartsheet.api.SheetRowResources Maven / Gradle / Ivy
Show all versions of smartsheet-sdk-java Show documentation
/*
* Copyright (C) 2023 Smartsheet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.smartsheet.api;
import com.smartsheet.api.models.CopyOrMoveRowDirective;
import com.smartsheet.api.models.CopyOrMoveRowResult;
import com.smartsheet.api.models.MultiRowEmail;
import com.smartsheet.api.models.PartialRowUpdateResult;
import com.smartsheet.api.models.Row;
import com.smartsheet.api.models.RowEmail;
import com.smartsheet.api.models.enums.ObjectExclusion;
import com.smartsheet.api.models.enums.RowCopyInclusion;
import com.smartsheet.api.models.enums.RowInclusion;
import com.smartsheet.api.models.enums.RowMoveInclusion;
import java.util.EnumSet;
import java.util.List;
import java.util.Set;
/**
* This interface provides methods to access row resources that are associated to a sheet object.
*
* Thread Safety: Implementation of this interface must be thread safe.
*/
public interface SheetRowResources {
/**
* Insert rows to a sheet.
*
* It mirrors to the following Smartsheet REST API method: POST /sheets/{id}/rows
*
* @param sheetId the sheet id
* @param rows the list of rows to create
* @return the list of created rows
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
List addRows(long sheetId, List rows) throws SmartsheetException;
/**
* Insert rows to a sheet.
*
* It mirrors to the following Smartsheet REST API method: POST /sheets/{id}/rows
*
* @param sheetId the sheet id
* @param rows the list of rows to create
* @param includes optional objects to include
* @param excludes optional objects to exclude
* @return the list of created rows
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
List addRows(
long sheetId,
List rows,
EnumSet includes,
EnumSet excludes
) throws SmartsheetException;
/**
* Insert rows to a sheet, allowing partial success. If a row cannot be inserted, it will fail, while the others may succeed..
*
* It mirrors to the following Smartsheet REST API method: POST /sheets/{id}/rows
*
* @param sheetId the sheet id
* @param rows the list of rows to create
* @return the list of created rows
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
PartialRowUpdateResult addRowsAllowPartialSuccess(long sheetId, List rows) throws SmartsheetException;
/**
* Insert rows to a sheet, allowing partial success. If a row cannot be inserted, it will fail, while the others may succeed..
*
* It mirrors to the following Smartsheet REST API method: POST /sheets/{id}/rows
*
* @param sheetId the sheet id
* @param rows the list of rows to create
* @param includes optional objects to include
* @param excludes optional objects to exclude
* @return the list of created rows
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
PartialRowUpdateResult addRowsAllowPartialSuccess(
long sheetId,
List rows,
EnumSet includes,
EnumSet excludes
) throws SmartsheetException;
/**
* Get a row.
*
* It mirrors to the following Smartsheet REST API method: GET /sheets/{sheetId}/rows/{rowId}
*
* @param sheetId the id of the sheet
* @param rowId the id of the row
* @param includes optional objects to include
* @param excludes optional objects to exclude
* @return the created row (note that if there is no such resource, this method will throw ResourceNotFoundException rather
* than returning null).
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
Row getRow(long sheetId, long rowId, EnumSet includes, EnumSet excludes) throws SmartsheetException;
/**
* Delete a row.
*
* It mirrors to the following Smartsheet REST API method: DELETE /sheets/{sheetId}/rows/{rowId}
*
* Exceptions:
* InvalidRequestException : if there is any problem with the REST API request
* AuthorizationException : if there is any problem with the REST API authorization(access token)
* ResourceNotFoundException : if the resource can not be found
* ServiceUnavailableException : if the REST API service is not available (possibly due to rate limiting)
* SmartsheetRestException : if there is any other REST API related error occurred during the operation
* SmartsheetException : if there is any other error occurred during the operation
*
* @param sheetId the sheet id
* @param rowId the row id
* @throws SmartsheetException the smartsheet exception
* @deprecated as of API 2.0.2 release, replaced by {@link #deleteRows(long, Set, boolean)}
*/
@Deprecated(since = "2.0.2", forRemoval = true)
void deleteRow(long sheetId, long rowId) throws SmartsheetException;
/**
* Send a row via email to the designated recipients.
*
* It mirrors to the following Smartsheet REST API method: POST /sheets/{sheetId}/rows/{rowId}/emails
*
* Exceptions:
* IllegalArgumentException : if any argument is null
* InvalidRequestException : if there is any problem with the REST API request
* AuthorizationException : if there is any problem with the REST API authorization(access token)
* ServiceUnavailableException : if the REST API service is not available (possibly due to rate limiting)
* SmartsheetRestException : if there is any other REST API related error occurred during the operation
* SmartsheetException : if there is any other error occurred during the operation
*
* @param sheetId the id of the sheet
* @param rowId the id of the row
* @param email the row email
* @throws SmartsheetException the smartsheet exception
* @deprecated as of API V2.0.2, replaced by {@link #sendRows(long, MultiRowEmail)}
*/
@Deprecated(since = "2.0.2", forRemoval = true)
void sendRow(long sheetId, long rowId, RowEmail email) throws SmartsheetException;
/**
* Send a row via email to the designated recipients.
*
* It mirrors to the following Smartsheet REST API method: POST /sheets/{sheetId}/rows/emails
*
* Exceptions:
* IllegalArgumentException : if any argument is null
* InvalidRequestException : if there is any problem with the REST API request
* AuthorizationException : if there is any problem with the REST API authorization(access token)
* ServiceUnavailableException : if the REST API service is not available (possibly due to rate limiting)
* SmartsheetRestException : if there is any other REST API related error occurred during the operation
* SmartsheetException : if there is any other error occurred during the operation
*
* @param sheetId the id of the sheet
* @param email the multi row email
* @throws SmartsheetException the smartsheet exception
*/
void sendRows(long sheetId, MultiRowEmail email) throws SmartsheetException;
/**
* Deletes one or more row(s) from the Sheet specified in the URL.
*
* It mirrors to the following Smartsheet REST API method: DELETE /sheets/{sheetId}/rows/{rowId}
*
* Exceptions:
* InvalidRequestException : if there is any problem with the REST API request
* AuthorizationException : if there is any problem with the REST API authorization(access token)
* ResourceNotFoundException : if the resource can not be found
* ServiceUnavailableException : if the REST API service is not available (possibly due to rate limiting)
* SmartsheetRestException : if there is any other REST API related error occurred during the operation
* SmartsheetException : if there is any other error occurred during the operation
*
* @param sheetId the sheet id
* @param rowIds the row ids
* @param ignoreRowsNotFound boolean for ignoring row ids not found
* @return a list of deleted rows
* @throws SmartsheetException the smartsheet exception
*/
List deleteRows(long sheetId, Set rowIds, boolean ignoreRowsNotFound) throws SmartsheetException;
/**
* Update rows.
*
* It mirrors to the following Smartsheet REST API method: PUT /sheets/{sheetId}/rows
*
* Exceptions:
* IllegalArgumentException : if any argument is null
* InvalidRequestException : if there is any problem with the REST API request
* AuthorizationException : if there is any problem with the REST API authorization(access token)
* ServiceUnavailableException : if the REST API service is not available (possibly due to rate limiting)
* SmartsheetRestException : if there is any other REST API related error occurred during the operation
* SmartsheetException : if there is any other error occurred during the operation
*
* @param sheetId the id of the sheet
* @param rows the list of rows
* @return a list of rows
* @throws SmartsheetException the smartsheet exception
*/
List updateRows(long sheetId, List rows) throws SmartsheetException;
/**
* Update rows.
*
* It mirrors to the following Smartsheet REST API method: PUT /sheets/{sheetId}/rows
*
* Exceptions:
* IllegalArgumentException : if any argument is null
* InvalidRequestException : if there is any problem with the REST API request
* AuthorizationException : if there is any problem with the REST API authorization(access token)
* ServiceUnavailableException : if the REST API service is not available (possibly due to rate limiting)
* SmartsheetRestException : if there is any other REST API related error occurred during the operation
* SmartsheetException : if there is any other error occurred during the operation
*
* @param sheetId the id of the sheet
* @param rows the list of rows
* @param includes optional objects to include
* @param excludes optional objects to exclude
* @return a list of rows
* @throws SmartsheetException the smartsheet exception
*/
List updateRows(
long sheetId,
List rows,
EnumSet includes,
EnumSet excludes
) throws SmartsheetException;
/**
* Update rows, but allow partial success. The PartialRowUpdateResult will contain the successful
* rows and those that failed, with specific messages for each.
*
* It mirrors to the following Smartsheet REST API method: PUT /sheets/{sheetId}/rows
*
* Exceptions:
* IllegalArgumentException : if any argument is null
* InvalidRequestException : if there is any problem with the REST API request
* AuthorizationException : if there is any problem with the REST API authorization(access token)
* ServiceUnavailableException : if the REST API service is not available (possibly due to rate limiting)
* SmartsheetRestException : if there is any other REST API related error occurred during the operation
* SmartsheetException : if there is any other error occurred during the operation
*
* @param sheetId the id of the sheet
* @param rows the list of rows
* @return a list of rows
* @throws SmartsheetException the smartsheet exception
*/
PartialRowUpdateResult updateRowsAllowPartialSuccess(long sheetId, List rows) throws SmartsheetException;
/**
* Update rows, but allow partial success. The PartialRowUpdateResult will contain the successful
* rows and those that failed, with specific messages for each.
*
* It mirrors to the following Smartsheet REST API method: PUT /sheets/{sheetId}/rows
*
* Exceptions:
* IllegalArgumentException : if any argument is null
* InvalidRequestException : if there is any problem with the REST API request
* AuthorizationException : if there is any problem with the REST API authorization(access token)
* ServiceUnavailableException : if the REST API service is not available (possibly due to rate limiting)
* SmartsheetRestException : if there is any other REST API related error occurred during the operation
* SmartsheetException : if there is any other error occurred during the operation
*
* @param sheetId the id of the sheet
* @param rows the list of rows
* @param includes optional objects to include
* @param excludes optional objects to exclude
* @return a list of rows
* @throws SmartsheetException the smartsheet exception
*/
PartialRowUpdateResult updateRowsAllowPartialSuccess(
long sheetId,
List rows,
EnumSet includes,
EnumSet excludes
) throws SmartsheetException;
/**
* Moves Row(s) from the Sheet specified in the URL to (the bottom of) another sheet.
*
* It mirrors to the following Smartsheet REST API method: POST /sheets/{sheetId}/rows/move
*
* Exceptions:
* IllegalArgumentException : if any argument is null, or path is empty string
* InvalidRequestException : if there is any problem with the REST API request
* AuthorizationException : if there is any problem with the REST API authorization(access token)
* ServiceUnavailableException : if the REST API service is not available (possibly due to rate limiting)
* SmartsheetRestException : if there is any other REST API related error occurred during the operation
* SmartsheetException : if there is any other error occurred during the operation
*
* @param sheetId the sheet ID to move
* @param includes the parameters to include
* @param ignoreRowsNotFound optional,specifying row Ids that do not exist within the source sheet
* @param moveParameters CopyOrMoveRowDirective object
* @return the result object
* @throws SmartsheetException the smartsheet exception
*/
CopyOrMoveRowResult moveRows(
Long sheetId,
EnumSet includes,
Boolean ignoreRowsNotFound,
CopyOrMoveRowDirective moveParameters
) throws SmartsheetException;
/**
* Copies Row(s) from the Sheet specified in the URL to (the bottom of) another sheet.
*
* It mirrors to the following Smartsheet REST API method: POST /sheets/{sheetId}/rows/move
*
* Exceptions:
* IllegalArgumentException : if any argument is null, or path is empty string
* InvalidRequestException : if there is any problem with the REST API request
* AuthorizationException : if there is any problem with the REST API authorization(access token)
* ServiceUnavailableException : if the REST API service is not available (possibly due to rate limiting)
* SmartsheetRestException : if there is any other REST API related error occurred during the operation
* SmartsheetException : if there is any other error occurred during the operation
*
* @param sheetId the sheet ID to move
* @param includes the parameters to include
* @param ignoreRowsNotFound optional,specifying row Ids that do not exist within the source sheet
* @param copyParameters CopyOrMoveRowDirective object
* @return the result object
* @throws SmartsheetException the smartsheet exception
*/
CopyOrMoveRowResult copyRows(
Long sheetId,
EnumSet includes,
Boolean ignoreRowsNotFound,
CopyOrMoveRowDirective copyParameters
) throws SmartsheetException;
/**
* Creates an object of RowAttachmentResources.
*
* @return the created RowAttachmentResources object
*/
RowAttachmentResources attachmentResources();
/**
* Creates an object of RowDiscussionResources.
*
* @return the created RowDiscussionResources object
*/
RowDiscussionResources discussionResources();
/**
* Creates an object of RowColumnResources.
*
* @return the created RowColumnResources object
*/
RowColumnResources cellResources();
}