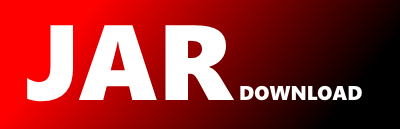
com.smartsheet.api.Smartsheet Maven / Gradle / Ivy
Show all versions of smartsheet-sdk-java Show documentation
/*
* Copyright (C) 2023 Smartsheet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.smartsheet.api;
/**
* This interface is the entry point of the Smartsheet SDK, it provides convenient methods to get XXXResources instances
* for accessing different types of resources.
*
* Thread Safety: Implementation of this interface must be thread safe.
*/
public interface Smartsheet {
/**
* Enable request/response tracing in client
* @param levels - what to trace (if anything; null if not tracing at all)
*/
void setTraces(Trace... levels);
/**
* enable/disable pretty JSON trace logging
* @param pretty if the JSON is nicely-formatted or compact
*/
void setTracePrettyPrint(boolean pretty);
/**
* Set the access token to use.
*
* @param accessToken the new access token
* @throws IllegalArgumentException if any argument is null/empty string
*/
void setAccessToken(String accessToken);
/**
* Set the email of the user to assume.
*
* @param assumedUser the new assumed user
* @throws IllegalArgumentException if any argument is null/empty string
*/
void setAssumedUser(String assumedUser);
/**
* Set the change agent identifier.
*
* @param changeAgent the new change agent
* @throws IllegalArgumentException if any argument is null/empty string
*/
void setChangeAgent(String changeAgent);
/**
* Set the user agent header string.
*
* @param userAgent the new user agent string
* @throws IllegalArgumentException if any argument is null/empty string
*/
void setUserAgent(String userAgent);
/**
* Sets the max retry time if the HttpClient is an instance of DefaultHttpClient
*
* @param maxRetryTimeMillis max retry time
*/
void setMaxRetryTimeMillis(long maxRetryTimeMillis);
/**
* Returns the HomeResources instance that provides access to Home resources.
*
* @return the home resources instance
*/
HomeResources homeResources();
/**
* Returns the WorkspaceResources instance that provides access to Workspace resources.
*
* @return the workspace resources instance
*/
WorkspaceResources workspaceResources();
/**
* Returns the FolderResources instance that provides access to Folder resources.
*
* @return the folder resources instance
*/
FolderResources folderResources();
/**
* Returns the TemplateResources instance that provides access to Template resources.
*
* @return the template resources instance
*/
TemplateResources templateResources();
/**
* Returns the SheetResources instance that provides access to Sheet resources.
*
* @return the sheet resources instance
*/
SheetResources sheetResources();
/**
* Returns the SightResources instance that provides access to Sight resources.
*
* @return the sight resources instance
*/
SightResources sightResources();
/**
* Returns the FavoriteResources instance that provides access to Favorite resources.
*
* @return the favorite resources instance
*/
FavoriteResources favoriteResources();
/**
* Returns the UserResources instance that provides access to User resources.
*
* @return the user resources instance
*/
UserResources userResources();
/**
* Returns the {@link GroupResources} instance that provides access to Group resources.
*
* @return the group resources instance
*/
GroupResources groupResources();
/**
* Returns the {@link ServerInfoResources} instance that provides access to Server Info resources.
*
* @return the serverinfo resources instance
*/
ServerInfoResources serverInfoResources();
/**
* Returns the SearchResources instance that provides access to searching resources.
*
* @return the search resources instance
*/
SearchResources searchResources();
/**
* Returns the ReportResources instance that provides access to report resources.
*
* @return the report resources instance
*/
ReportResources reportResources();
/**
* Returns the TokenResources instance that provides access to token resources.
*
* @return the token resources instance
*/
TokenResources tokenResources();
/**
* Returns the ContactResources instance that provides access to contact resources.
*
* @return the contact resources instance
*/
ContactResources contactResources();
/**
* Returns the ImageUrlResources instance that provides access to image Url resources
*
* @return the image Url resources instance
*/
ImageUrlResources imageUrlResources();
/**
* Returns the WebhookResources instance that provides access to webhook resources
*
* @return the webhook resources instance
*/
WebhookResources webhookResources();
/**
* Returns the PassthroughResources instance that provides access to passthrough resources
*
* @return the passthrough resources instance
*/
PassthroughResources passthroughResources();
/**
* Returns the EventResources instance that provides access to event resources
*
* @return the event resources instance
*/
EventResources eventResources();
}