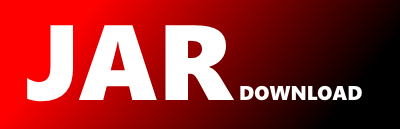
com.smartsheet.api.SmartsheetFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartsheet-sdk-java Show documentation
Show all versions of smartsheet-sdk-java Show documentation
Library for connecting to Smartsheet Services
/*
* Copyright (C) 2023 Smartsheet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.smartsheet.api;
import com.smartsheet.api.internal.SmartsheetImpl;
public class SmartsheetFactory {
/**
* Represents the default base URI of the Smartsheet REST API.
*
* It is a constant with value "https://api.smartsheet.com/2.0".
*/
public static final String DEFAULT_BASE_URI = "https://api.smartsheet.com/2.0/";
/**
* Represents the default base URI of the Smartsheetgov REST API.
*
* It is a constant with value "https://api.smartsheetgov.com/2.0".
*/
public static final String GOV_BASE_URI = "https://api.smartsheetgov.com/2.0/";
/**
* Creates a Smartsheet client with default parameters. SMARTSHEET_ACCESS_TOKEN
* must be set in the environment.
*
* @return the Smartsheet client
*/
public static Smartsheet createDefaultClient() {
String accessToken = System.getenv("SMARTSHEET_ACCESS_TOKEN");
SmartsheetImpl smartsheet = new SmartsheetImpl(DEFAULT_BASE_URI, accessToken);
return smartsheet;
}
/**
* Creates a Smartsheet client with default parameters.
*
* @return the Smartsheet client
*/
public static Smartsheet createDefaultClient(String accessToken) {
SmartsheetImpl smartsheet = new SmartsheetImpl(DEFAULT_BASE_URI, accessToken);
return smartsheet;
}
/**
* Creates a Smartsheet client with default parameters using the Smartsheetgov URI.
* SMARTSHEET_ACCESS_TOKEN must be set in the environment.
*
* @return the Smartsheet client
*/
public static Smartsheet createDefaultGovAccountClient() {
String accessToken = System.getenv("SMARTSHEET_ACCESS_TOKEN");
SmartsheetImpl smartsheet = new SmartsheetImpl(GOV_BASE_URI, accessToken);
return smartsheet;
}
/**
* Creates a Smartsheet client with default parameters using the Smartsheetgov URI.
*
* @return the Smartsheet client
*/
public static Smartsheet createDefaultGovAccountClient(String accessToken) {
SmartsheetImpl smartsheet = new SmartsheetImpl(GOV_BASE_URI, accessToken);
return smartsheet;
}
/**
* Returns a builder to allow the caller to create a custom Smartsheet client.
*
* @return the SmartsheetBuilder
*/
public static SmartsheetBuilder custom() {
return new SmartsheetBuilder();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy