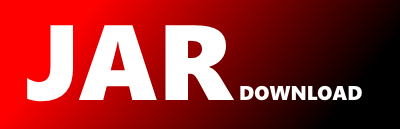
com.smartsheet.api.UserResources Maven / Gradle / Ivy
Show all versions of smartsheet-sdk-java Show documentation
/*
* Copyright (C) 2023 Smartsheet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.smartsheet.api;
import com.smartsheet.api.models.AlternateEmail;
import com.smartsheet.api.models.DeleteUserParameters;
import com.smartsheet.api.models.PagedResult;
import com.smartsheet.api.models.PaginationParameters;
import com.smartsheet.api.models.Sheet;
import com.smartsheet.api.models.User;
import com.smartsheet.api.models.UserProfile;
import com.smartsheet.api.models.enums.ListUserInclusion;
import com.smartsheet.api.models.enums.UserInclusion;
import java.io.FileNotFoundException;
import java.util.Date;
import java.util.EnumSet;
import java.util.List;
import java.util.Set;
/**
* This interface provides methods to access User resources.
*
* Thread Safety: Implementation of this interface must be thread safe.
*/
public interface UserResources {
/**
* List all users.
*
* It mirrors to the following Smartsheet REST API method: GET /users
*
* @return the list of all users
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
PagedResult listUsers() throws SmartsheetException;
/**
* List all users.
*
* It mirrors to the following Smartsheet REST API method: GET /users
*
* @param email the list of email addresses
* @param pagination object containing pagination query parameters
* @return the list of all users
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
PagedResult listUsers(Set email, PaginationParameters pagination) throws SmartsheetException;
/**
* List all users.
*
* It mirrors to the following Smartsheet REST API method: GET /users
*
* @param email the list of email addresses
* @param includes elements to include in response
* @param pagination object containing pagination query parameters
* @return the list of all users
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
PagedResult listUsers(Set email, EnumSet includes,
PaginationParameters pagination) throws SmartsheetException;
/**
* Add a user to the organization, without sending email.
*
* It mirrors to the following Smartsheet REST API method: POST /users
*
* @param user the user object
* @return the user
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
User addUser(User user) throws SmartsheetException;
/**
* Add a user to the organization, without sending email.
*
* It mirrors to the following Smartsheet REST API method: POST /users
*
* @param user the user
* @param sendEmail the send email flag
* @return the user
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
User addUser(User user, boolean sendEmail) throws SmartsheetException;
/**
* Get the current user.
*
* It mirrors to the following Smartsheet REST API method: GET /users/{userId}
*
* @param userId the user id
* @return the current user
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
UserProfile getUser(long userId) throws SmartsheetException;
/**
* Get the current user.
*
* It mirrors to the following Smartsheet REST API method: GET /user/me
*
* @return the current user
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
UserProfile getCurrentUser() throws SmartsheetException;
/**
* Get the current user.
*
* It mirrors to the following Smartsheet REST API method: GET /user/me
*
* @param includes used to specify the optional objects to include.
* @return the current user
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
UserProfile getCurrentUser(EnumSet includes) throws SmartsheetException;
/**
* Update a user.
*
* It mirrors to the following Smartsheet REST API method: PUT /user/{id}
*
* @param user the user to update
* @return the updated user
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
User updateUser(User user) throws SmartsheetException;
/**
* Delete a user in the organization.
*
* It mirrors to the following Smartsheet REST API method: DELETE /user/{id}
*
* @param id the id of the user
* @param parameters the object containing parameters for deleting users
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
void deleteUser(long id, DeleteUserParameters parameters) throws SmartsheetException;
/**
* List all organisation sheets.
*
* It mirrors to the following Smartsheet REST API method: GET /users/sheets
*
* @param pagination the object containing the pagination query parameters
* @param modifiedSince restrict to sheets modified on or after this date
* @return the list of all organisation sheets
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
PagedResult listOrgSheets(PaginationParameters pagination, Date modifiedSince) throws SmartsheetException;
/**
* List all organisation sheets.
*
* It mirrors to the following Smartsheet REST API method: GET /users/sheets
*
* @param pagination the object containing the pagination query parameters
* @return the list of all organisation sheets
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
PagedResult listOrgSheets(PaginationParameters pagination) throws SmartsheetException;
/**
* List all user alternate email(s).
*
* It mirrors to the following Smartsheet REST API method: GET /users/{userId}/alternateemails
*
* @param userId the userID
* @param pagination the pagination parameters
* @return the list of all user alternate email(s)
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
PagedResult listAlternateEmails(long userId, PaginationParameters pagination) throws SmartsheetException;
/**
* Get alternate email.
*
* It mirrors to the following Smartsheet REST API method: GET /users/{userId}/alternateemails/{alternateEmailId}
*
* @param userId the id of the user
* @param altEmailId the alternate email id for the alternate email to retrieve.
* @return the resource. Note that if there is no such resource, this method will throw
* ResourceNotFoundException rather than returning null.
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
AlternateEmail getAlternateEmail(long userId, long altEmailId) throws SmartsheetException;
/**
* Add an alternate email.
*
* It mirrors to the following Smartsheet REST API method: POST /users/{userId}/alternateemails
*
* @param userId the id of the user
* @param altEmails List of alternate email address to add.
* @return List of added alternate email(s).
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
List addAlternateEmail(long userId, List altEmails) throws SmartsheetException;
/**
* Delete an alternate email.
*
* It mirrors to the following Smartsheet REST API method: DELETE /users/{userId}/alternateemails/{alternateEmailId}
*
* @param userId the id of the user
* @param altEmailId the alternate email id for the alternate email to retrieve.
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException if there is any other error during the operation
*/
void deleteAlternateEmail(long userId, long altEmailId) throws SmartsheetException;
/**
* Promote and alternate email to primary.
*
* @param userId id of the user
* @param altEmailId alternate email id
* @return alternateEmail of the primary
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException f there is any other error during the operation
*/
AlternateEmail promoteAlternateEmail(long userId, long altEmailId) throws SmartsheetException;
/**
* Uploads a profile image for the specified user.
*
* @param userId id of the user
* @param file path to the image file
* @param fileType content type of the image file
* @return user
* @throws IllegalArgumentException if any argument is null or empty string
* @throws InvalidRequestException if there is any problem with the REST API request
* @throws AuthorizationException if there is any problem with the REST API authorization (access token)
* @throws ResourceNotFoundException if the resource cannot be found
* @throws ServiceUnavailableException if the REST API service is not available (possibly due to rate limiting)
* @throws SmartsheetException f there is any other error during the operation
*/
User addProfileImage(long userId, String file, String fileType) throws SmartsheetException, FileNotFoundException;
}