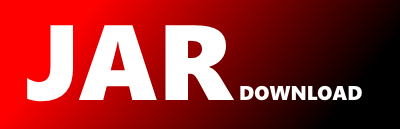
com.smartsheet.api.models.ChartWidgetContent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartsheet-sdk-java Show documentation
Show all versions of smartsheet-sdk-java Show documentation
Library for connecting to Smartsheet Services
/*
* Copyright (C) 2023 Smartsheet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.smartsheet.api.models;
import com.smartsheet.api.models.enums.WidgetType;
import java.util.List;
public class ChartWidgetContent implements WidgetContent {
/**
* Report Id denoting container source, if applicable
*/
private Long reportId;
/**
* Sheet Id denoting container source, if applicable
*/
private Long sheetId;
/**
* Array of Axes
*/
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy