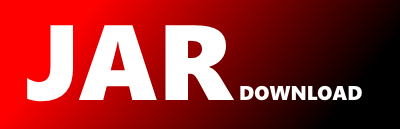
com.smartsheet.api.models.Schedule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartsheet-sdk-java Show documentation
Show all versions of smartsheet-sdk-java Show documentation
Library for connecting to Smartsheet Services
/*
* Copyright (C) 2023 Smartsheet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.smartsheet.api.models;
import com.smartsheet.api.models.enums.DayDescriptor;
import com.smartsheet.api.models.enums.DayOrdinal;
import com.smartsheet.api.models.enums.ScheduleType;
import java.util.Date;
import java.util.List;
public class Schedule {
/**
* Schedule type
*/
private ScheduleType type;
/**
* The date, time and time zone at which the first delivery will start.
*/
private Date startAt;
/**
* The date, time and time zone at which the delivery schedule will end.
*/
private Date endAt;
/**
* The day within the month
*/
private Integer dayOfMonth;
/**
* The day ordinal
*/
private DayOrdinal dayOrdinal;
/**
* An array of day descriptors
*/
private List dayDescriptors;
/**
* Frequency on which the request will be delivered
*/
private Integer repeatEvery;
/**
* The date and time for when the last request was sent.
*/
private Date lastSentAt;
/**
* The date and time for when the next request is scheduled to send.
*/
private Date nextSendAt;
/**
* Get the schedule type
*
* @return type
*/
public ScheduleType getType() {
return type;
}
/**
* Set the schedule type
*/
public Schedule setType(ScheduleType type) {
this.type = type;
return this;
}
/**
* Get the date, time and time zone at which the first delivery will start
*
* @return startAt
*/
public Date getStartAt() {
return startAt;
}
/**
* Set the date, time and time zone at which the first delivery will start
*/
public Schedule setStartAt(Date startAt) {
this.startAt = startAt;
return this;
}
/**
* Get the date, time and time zone at which the delivery schedule will end
*
* @return endAt
*/
public Date getEndAt() {
return endAt;
}
/**
* Set the date, time and time zone at which the delivery schedule will end
*/
public Schedule setEndAt(Date endAt) {
this.endAt = endAt;
return this;
}
/**
* Get the day within the month
*
* @return dayOfMonth
*/
public Integer getDayOfMonth() {
return dayOfMonth;
}
/**
* Set the day within the month
*/
public Schedule setDayOfMonth(Integer dayOfMonth) {
this.dayOfMonth = dayOfMonth;
return this;
}
/**
* Get the day ordinal
*
* @return dayOrdinal
*/
public DayOrdinal getDayOrdinal() {
return dayOrdinal;
}
/**
* Set the day ordinal
*/
public Schedule setDayOrdinal(DayOrdinal dayOrdinal) {
this.dayOrdinal = dayOrdinal;
return this;
}
/**
* Get an array of day descriptors
*
* @return dayDescriptors
*/
public List getDayDescriptors() {
return dayDescriptors;
}
/**
* Set the array of day descriptors
*/
public Schedule setDayDescriptors(List dayDescriptors) {
this.dayDescriptors = dayDescriptors;
return this;
}
/**
* Get the frequency on which the request will be delivered.
*
* @return repeatEvery
*/
public Integer getRepeatEvery() {
return repeatEvery;
}
/**
* Set the frequency on which the request will be delivered.
*/
public Schedule setRepeatEvery(Integer repeatEvery) {
this.repeatEvery = repeatEvery;
return this;
}
/**
* Get the date and time for when the last request was sent.
*
* @return lastSentAt
*/
public Date getLastSentAt() {
return lastSentAt;
}
/**
* Set the date and time for when the last request was sent.
*/
public Schedule setLastSentAt(Date lastSentAt) {
this.lastSentAt = lastSentAt;
return this;
}
/**
* Get the date and time for when the next request is scheduled to send.
*
* @return nextSendAt
*/
public Date getNextSendAt() {
return nextSendAt;
}
/**
* Set the date and time for when the next request is schedule to send.
*/
public Schedule sentNextSendAt(Date nextSendAt) {
this.nextSendAt = nextSendAt;
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy