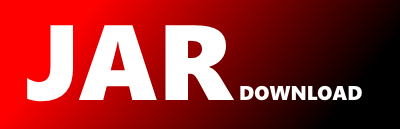
com.smartsheet.api.models.ShortcutDataItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartsheet-sdk-java Show documentation
Show all versions of smartsheet-sdk-java Show documentation
Library for connecting to Smartsheet Services
/*
* Copyright (C) 2023 Smartsheet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.smartsheet.api.models;
import com.smartsheet.api.models.enums.AttachmentType;
import com.smartsheet.api.models.format.Format;
public class ShortcutDataItem {
/**
* Lable for the data point
*/
private String label;
/**
* format Descriptor
*/
private Format labelFormat;
/**
* Attachment type(one of FILE, GOOGLE_DRIVE, LINK, BOX_COM, DROPBOX, EVERNOTE, EGNYTE, ONEDRIVE, SMARTSHEET)
*/
private AttachmentType attachmentType;
/**
* Hyperlink object
*/
private Hyperlink hyperlink;
/**
* The display order for the Shortcut
*/
private Integer order;
/**
* The mime type for the Shortcut
*/
private String mimeType;
/**
* Get the label for the data point.
*
* @return label
*/
public String getLabel() {
return label;
}
/**
* Set the label for the data point.
*/
public ShortcutDataItem setLabel(String label) {
this.label = label;
return this;
}
/**
* Get the label format string
*
* @return labelFormat;
*/
public Format getLabelFormat() {
return labelFormat;
}
/**
* Set the label format string
*/
public ShortcutDataItem setLabelFormat(Format labelFormat) {
this.labelFormat = labelFormat;
return this;
}
/**
* Get the attachment type
* (one of FILE, GOOGLE_DRIVE, LINK, BOX_COM, DROPBOX, EVERNOTE, EGNYTE, ONEDRIVE, SMARTSHEET)
*
* @return attachmentType
*/
public AttachmentType getAttachmentType() {
return attachmentType;
}
/**
* Set the attachment type
* (one of FILE, GOOGLE_DRIVE, LINK, BOX_COM, DROPBOX, EVERNOTE, or EGNYTE).
*/
public ShortcutDataItem setAttachmentType(AttachmentType attachmentType) {
this.attachmentType = attachmentType;
return this;
}
/**
* Get the hyperlink object
*
* @return hyperlink
*/
public Hyperlink getHyperlink() {
return hyperlink;
}
/**
* Set the hyperlink object
*/
public ShortcutDataItem setHyperlink(Hyperlink hyperlink) {
this.hyperlink = hyperlink;
return this;
}
/**
* Get the display order for this shortcut data item
*
* @return order
*/
public Integer getOrder() {
return order;
}
/**
* Set the display order for this shortcut data item
*/
public ShortcutDataItem setOrder(Integer order) {
this.order = order;
return this;
}
/**
* Get the MIME type
*
* @return mimeType
*/
public String getMimeType() {
return mimeType;
}
/**
* Set the MIME Type
*/
public ShortcutDataItem setMimeType(String mimeType) {
this.mimeType = mimeType;
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy