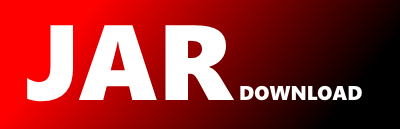
com.smartsheet.api.models.FormatTables Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartsheet-sdk-java Show documentation
Show all versions of smartsheet-sdk-java Show documentation
Library for connecting to Smartsheet Services
/*
* Copyright (C) 2024 Smartsheet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.smartsheet.api.models;
import java.util.List;
public class FormatTables {
/**
* Represents the format descriptor.
*/
private String defaults;
/**
* Represents Possible bold values: none,on.
*/
private List bold;
/**
* Represents Color hex values.
*/
private List color;
/**
* Represents Currency codes and symbols.
*/
private List currency;
/**
* Array of strings containing available date formats
*/
private List dateFormat;
/**
* Represents All allowed decimal count values.
*/
private List decimalCount;
/**
* Represents Font families with additional font information.
*/
private List fontFamily;
/**
* Represents Font sizes in points.
*/
private List fontSize;
/**
* Represents Possible horizontalAlign values: none,on.
*/
private List horizontalAlign;
/**
* Represents Possible italic values: none,on.
*/
private List italic;
/**
* Represents Possible numberFormat values: none
* NUMBER
* CURRENCY
* PERCENT.
*/
private List numberFormat;
/**
* Represents Possible strikethrough values: none,on.
*/
private List strikethrough;
/**
* Represents Possible textWrap values: none,on.
*/
private List textWrap;
/**
* Represents Possible thousandsSeparator values: none,on.
*/
private List thousandsSeparator;
/**
* Represents Possible underline values: none,on.
*/
private List underline;
/**
* Represents Possible verticalAlign values: top, middle, bottom.
*/
private List verticalAlign;
/**
* Gets the format descriptor.
*
* @return the defaults
*/
public String getDefaults() {
return defaults;
}
/**
* Sets the format descriptor.
*
* @param defaults the new defaults
*/
public FormatTables setDefaults(String defaults) {
this.defaults = defaults;
return this;
}
/**
* Gets the Possible bold values.
*
* @return the Possible bold values
*/
public List getBold() {
return bold;
}
/**
* Sets the Possible bold values.
*
* @param bold the new Possible bold values
*/
public FormatTables setBold(List bold) {
this.bold = bold;
return this;
}
/**
* Gets the Color hex values.
*
* @return the Color hex values
*/
public List getColor() {
return color;
}
/**
* Sets the Color hex values.
*
* @param color the new Color hex values
*/
public FormatTables setColor(List color) {
this.color = color;
return this;
}
/**
* Gets the Currency codes and symbols.
*
* @return the Currency codes and symbols
*/
public List getCurrency() {
return currency;
}
/**
* Sets the Currency codes and symbols.
*
* @param currency the new Currency codes and symbols
*/
public FormatTables setCurrency(List currency) {
this.currency = currency;
return this;
}
/**
* Gets the array of strings containing available date formats
*
* @return the date formats
*/
public List getDateFormat() {
return dateFormat;
}
/**
* Sets the array of strings containing available date formats
*
* @param dateFormat the date formats
*/
public FormatTables setDateFormat(List dateFormat) {
this.dateFormat = dateFormat;
return this;
}
/**
* Gets the allowed decimal count values.
*
* @return the allowed decimal count values
*/
public List getDecimalCount() {
return decimalCount;
}
/**
* Sets the allowed decimal count values.
*
* @param decimalCount the new allowed decimal count values
*/
public FormatTables setDecimalCount(List decimalCount) {
this.decimalCount = decimalCount;
return this;
}
/**
* Gets the Font families.
*
* @return the Font families
*/
public List getFontFamily() {
return fontFamily;
}
/**
* Sets the Font families.
*
* @param fontFamily the new Font families
*/
public FormatTables setFontFamily(List fontFamily) {
this.fontFamily = fontFamily;
return this;
}
/**
* Gets the Font sizes in points.
*
* @return the Font sizes in points
*/
public List getFontSize() {
return fontSize;
}
/**
* Sets the Font sizes in points.
*
* @param fontSize the new Font sizes in points
*/
public FormatTables setFontSize(List fontSize) {
this.fontSize = fontSize;
return this;
}
/**
* Gets the Possible horizontalAlign values.
*
* @return the Possible horizontalAlign values
*/
public List getHorizontalAlign() {
return horizontalAlign;
}
/**
* Sets the Possible horizontalAlign values.
*
* @param horizontalAlign the new Possible horizontalAlign values
*/
public FormatTables setHorizontalAlign(List horizontalAlign) {
this.horizontalAlign = horizontalAlign;
return this;
}
/**
* Gets the Possible italic values.
*
* @return the Possible italic values
*/
public List getItalic() {
return italic;
}
/**
* Sets the Possible italic values.
*
* @param italic the new Possible italic values
*/
public FormatTables setItalic(List italic) {
this.italic = italic;
return this;
}
/**
* Gets the numberFormat values.
*
* @return the numberFormat values
*/
public List getNumberFormat() {
return numberFormat;
}
/**
* Sets the numberFormat values.
*
* @param numberFormat the new numberFormat values
*/
public FormatTables setNumberFormat(List numberFormat) {
this.numberFormat = numberFormat;
return this;
}
/**
* Gets the Possible strikethrough values.
*
* @return the Possible strikethrough values
*/
public List getStrikethrough() {
return strikethrough;
}
/**
* Sets the Possible strikethrough values.
*
* @param strikethrough the new Possible strikethrough values
*/
public FormatTables setStrikethrough(List strikethrough) {
this.strikethrough = strikethrough;
return this;
}
/**
* Gets the textWrap values.
*
* @return the textWrap values
*/
public List getTextWrap() {
return textWrap;
}
/**
* Sets the textWrap values.
*
* @param textWrap the new textWrap values
*/
public FormatTables setTextWrap(List textWrap) {
this.textWrap = textWrap;
return this;
}
/**
* Gets the thousandsSeparator values.
*
* @return the thousandsSeparator values
*/
public List getThousandsSeparator() {
return thousandsSeparator;
}
/**
* Sets the thousandsSeparator values.
*
* @param thousandsSeparator the new thousandsSeparator values
*/
public FormatTables setThousandsSeparator(List thousandsSeparator) {
this.thousandsSeparator = thousandsSeparator;
return this;
}
/**
* Gets the Possible underline values.
*
* @return the Possible underline values
*/
public List getUnderline() {
return underline;
}
/**
* Sets the Possible underline values.
*
* @param underline the new Possible underline values
*/
public FormatTables setUnderline(List underline) {
this.underline = underline;
return this;
}
/**
* Gets the Possible verticalAlign values.
*
* @return the Possible verticalAlign values
*/
public List getVerticalAlign() {
return verticalAlign;
}
/**
* Sets the Possible verticalAlign values.
*
* @param verticalAlign the new Possible verticalAlign values
*/
public FormatTables setVerticalAlign(List verticalAlign) {
this.verticalAlign = verticalAlign;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy