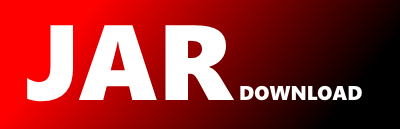
com.smoketurner.notification.application.protos.NotificationProtos Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: notification.proto
package com.smoketurner.notification.application.protos;
public final class NotificationProtos {
private NotificationProtos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface PropertyOrBuilder extends
// @@protoc_insertion_point(interface_extends:notification.Property)
com.google.protobuf.MessageOrBuilder {
/**
* required string key = 1;
*/
boolean hasKey();
/**
* required string key = 1;
*/
java.lang.String getKey();
/**
* required string key = 1;
*/
com.google.protobuf.ByteString
getKeyBytes();
/**
* required string value = 2;
*/
boolean hasValue();
/**
* required string value = 2;
*/
java.lang.String getValue();
/**
* required string value = 2;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
* Protobuf type {@code notification.Property}
*/
public static final class Property extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:notification.Property)
PropertyOrBuilder {
// Use Property.newBuilder() to construct.
private Property(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Property(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Property defaultInstance;
public static Property getDefaultInstance() {
return defaultInstance;
}
public Property getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Property(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
key_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
value_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_Property_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_Property_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.smoketurner.notification.application.protos.NotificationProtos.Property.class, com.smoketurner.notification.application.protos.NotificationProtos.Property.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Property parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Property(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
private java.lang.Object key_;
/**
* required string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
}
}
/**
* required string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
private java.lang.Object value_;
/**
* required string value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
key_ = "";
value_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getKeyBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getValueBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getKeyBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getValueBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.smoketurner.notification.application.protos.NotificationProtos.Property parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.Property parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.Property parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.Property parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.Property parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.Property parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.Property parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.Property parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.Property parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.Property parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.smoketurner.notification.application.protos.NotificationProtos.Property prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code notification.Property}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:notification.Property)
com.smoketurner.notification.application.protos.NotificationProtos.PropertyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_Property_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_Property_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.smoketurner.notification.application.protos.NotificationProtos.Property.class, com.smoketurner.notification.application.protos.NotificationProtos.Property.Builder.class);
}
// Construct using com.smoketurner.notification.application.protos.NotificationProtos.Property.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
key_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
value_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_Property_descriptor;
}
public com.smoketurner.notification.application.protos.NotificationProtos.Property getDefaultInstanceForType() {
return com.smoketurner.notification.application.protos.NotificationProtos.Property.getDefaultInstance();
}
public com.smoketurner.notification.application.protos.NotificationProtos.Property build() {
com.smoketurner.notification.application.protos.NotificationProtos.Property result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.smoketurner.notification.application.protos.NotificationProtos.Property buildPartial() {
com.smoketurner.notification.application.protos.NotificationProtos.Property result = new com.smoketurner.notification.application.protos.NotificationProtos.Property(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.key_ = key_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.smoketurner.notification.application.protos.NotificationProtos.Property) {
return mergeFrom((com.smoketurner.notification.application.protos.NotificationProtos.Property)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.smoketurner.notification.application.protos.NotificationProtos.Property other) {
if (other == com.smoketurner.notification.application.protos.NotificationProtos.Property.getDefaultInstance()) return this;
if (other.hasKey()) {
bitField0_ |= 0x00000001;
key_ = other.key_;
onChanged();
}
if (other.hasValue()) {
bitField0_ |= 0x00000002;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasKey()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.smoketurner.notification.application.protos.NotificationProtos.Property parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.smoketurner.notification.application.protos.NotificationProtos.Property) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object key_ = "";
/**
* required string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string key = 1;
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
/**
* required string key = 1;
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* required string key = 1;
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
private java.lang.Object value_ = "";
/**
* required string value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 2;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 2;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 2;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:notification.Property)
}
static {
defaultInstance = new Property(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:notification.Property)
}
public interface NotificationPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:notification.NotificationPB)
com.google.protobuf.MessageOrBuilder {
/**
* required int64 id = 1;
*/
boolean hasId();
/**
* required int64 id = 1;
*/
long getId();
/**
* required string category = 2;
*/
boolean hasCategory();
/**
* required string category = 2;
*/
java.lang.String getCategory();
/**
* required string category = 2;
*/
com.google.protobuf.ByteString
getCategoryBytes();
/**
* required string message = 3;
*/
boolean hasMessage();
/**
* required string message = 3;
*/
java.lang.String getMessage();
/**
* required string message = 3;
*/
com.google.protobuf.ByteString
getMessageBytes();
/**
* required int64 created_at = 4;
*/
boolean hasCreatedAt();
/**
* required int64 created_at = 4;
*/
long getCreatedAt();
/**
* repeated .notification.Property property = 5;
*/
java.util.List
getPropertyList();
/**
* repeated .notification.Property property = 5;
*/
com.smoketurner.notification.application.protos.NotificationProtos.Property getProperty(int index);
/**
* repeated .notification.Property property = 5;
*/
int getPropertyCount();
/**
* repeated .notification.Property property = 5;
*/
java.util.List extends com.smoketurner.notification.application.protos.NotificationProtos.PropertyOrBuilder>
getPropertyOrBuilderList();
/**
* repeated .notification.Property property = 5;
*/
com.smoketurner.notification.application.protos.NotificationProtos.PropertyOrBuilder getPropertyOrBuilder(
int index);
}
/**
* Protobuf type {@code notification.NotificationPB}
*/
public static final class NotificationPB extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:notification.NotificationPB)
NotificationPBOrBuilder {
// Use NotificationPB.newBuilder() to construct.
private NotificationPB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private NotificationPB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final NotificationPB defaultInstance;
public static NotificationPB getDefaultInstance() {
return defaultInstance;
}
public NotificationPB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NotificationPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
id_ = input.readInt64();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
category_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
message_ = bs;
break;
}
case 32: {
bitField0_ |= 0x00000008;
createdAt_ = input.readInt64();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
property_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
property_.add(input.readMessage(com.smoketurner.notification.application.protos.NotificationProtos.Property.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
property_ = java.util.Collections.unmodifiableList(property_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_NotificationPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_NotificationPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.class, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public NotificationPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NotificationPB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private long id_;
/**
* required int64 id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 id = 1;
*/
public long getId() {
return id_;
}
public static final int CATEGORY_FIELD_NUMBER = 2;
private java.lang.Object category_;
/**
* required string category = 2;
*/
public boolean hasCategory() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string category = 2;
*/
public java.lang.String getCategory() {
java.lang.Object ref = category_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
category_ = s;
}
return s;
}
}
/**
* required string category = 2;
*/
public com.google.protobuf.ByteString
getCategoryBytes() {
java.lang.Object ref = category_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
category_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MESSAGE_FIELD_NUMBER = 3;
private java.lang.Object message_;
/**
* required string message = 3;
*/
public boolean hasMessage() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required string message = 3;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
message_ = s;
}
return s;
}
}
/**
* required string message = 3;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CREATED_AT_FIELD_NUMBER = 4;
private long createdAt_;
/**
* required int64 created_at = 4;
*/
public boolean hasCreatedAt() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required int64 created_at = 4;
*/
public long getCreatedAt() {
return createdAt_;
}
public static final int PROPERTY_FIELD_NUMBER = 5;
private java.util.List property_;
/**
* repeated .notification.Property property = 5;
*/
public java.util.List getPropertyList() {
return property_;
}
/**
* repeated .notification.Property property = 5;
*/
public java.util.List extends com.smoketurner.notification.application.protos.NotificationProtos.PropertyOrBuilder>
getPropertyOrBuilderList() {
return property_;
}
/**
* repeated .notification.Property property = 5;
*/
public int getPropertyCount() {
return property_.size();
}
/**
* repeated .notification.Property property = 5;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.Property getProperty(int index) {
return property_.get(index);
}
/**
* repeated .notification.Property property = 5;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.PropertyOrBuilder getPropertyOrBuilder(
int index) {
return property_.get(index);
}
private void initFields() {
id_ = 0L;
category_ = "";
message_ = "";
createdAt_ = 0L;
property_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasCategory()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasMessage()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasCreatedAt()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getPropertyCount(); i++) {
if (!getProperty(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getCategoryBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getMessageBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt64(4, createdAt_);
}
for (int i = 0; i < property_.size(); i++) {
output.writeMessage(5, property_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getCategoryBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getMessageBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, createdAt_);
}
for (int i = 0; i < property_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, property_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code notification.NotificationPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:notification.NotificationPB)
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_NotificationPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_NotificationPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.class, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.Builder.class);
}
// Construct using com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getPropertyFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
id_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
category_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
message_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
createdAt_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
if (propertyBuilder_ == null) {
property_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
propertyBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_NotificationPB_descriptor;
}
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB getDefaultInstanceForType() {
return com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.getDefaultInstance();
}
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB build() {
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB buildPartial() {
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB result = new com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.category_ = category_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.message_ = message_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.createdAt_ = createdAt_;
if (propertyBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
property_ = java.util.Collections.unmodifiableList(property_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.property_ = property_;
} else {
result.property_ = propertyBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB) {
return mergeFrom((com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB other) {
if (other == com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.getDefaultInstance()) return this;
if (other.hasId()) {
setId(other.getId());
}
if (other.hasCategory()) {
bitField0_ |= 0x00000002;
category_ = other.category_;
onChanged();
}
if (other.hasMessage()) {
bitField0_ |= 0x00000004;
message_ = other.message_;
onChanged();
}
if (other.hasCreatedAt()) {
setCreatedAt(other.getCreatedAt());
}
if (propertyBuilder_ == null) {
if (!other.property_.isEmpty()) {
if (property_.isEmpty()) {
property_ = other.property_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensurePropertyIsMutable();
property_.addAll(other.property_);
}
onChanged();
}
} else {
if (!other.property_.isEmpty()) {
if (propertyBuilder_.isEmpty()) {
propertyBuilder_.dispose();
propertyBuilder_ = null;
property_ = other.property_;
bitField0_ = (bitField0_ & ~0x00000010);
propertyBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getPropertyFieldBuilder() : null;
} else {
propertyBuilder_.addAllMessages(other.property_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasId()) {
return false;
}
if (!hasCategory()) {
return false;
}
if (!hasMessage()) {
return false;
}
if (!hasCreatedAt()) {
return false;
}
for (int i = 0; i < getPropertyCount(); i++) {
if (!getProperty(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long id_ ;
/**
* required int64 id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 id = 1;
*/
public long getId() {
return id_;
}
/**
* required int64 id = 1;
*/
public Builder setId(long value) {
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* required int64 id = 1;
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object category_ = "";
/**
* required string category = 2;
*/
public boolean hasCategory() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string category = 2;
*/
public java.lang.String getCategory() {
java.lang.Object ref = category_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
category_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string category = 2;
*/
public com.google.protobuf.ByteString
getCategoryBytes() {
java.lang.Object ref = category_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
category_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string category = 2;
*/
public Builder setCategory(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
category_ = value;
onChanged();
return this;
}
/**
* required string category = 2;
*/
public Builder clearCategory() {
bitField0_ = (bitField0_ & ~0x00000002);
category_ = getDefaultInstance().getCategory();
onChanged();
return this;
}
/**
* required string category = 2;
*/
public Builder setCategoryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
category_ = value;
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
* required string message = 3;
*/
public boolean hasMessage() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required string message = 3;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
message_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string message = 3;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string message = 3;
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
message_ = value;
onChanged();
return this;
}
/**
* required string message = 3;
*/
public Builder clearMessage() {
bitField0_ = (bitField0_ & ~0x00000004);
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
* required string message = 3;
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
message_ = value;
onChanged();
return this;
}
private long createdAt_ ;
/**
* required int64 created_at = 4;
*/
public boolean hasCreatedAt() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required int64 created_at = 4;
*/
public long getCreatedAt() {
return createdAt_;
}
/**
* required int64 created_at = 4;
*/
public Builder setCreatedAt(long value) {
bitField0_ |= 0x00000008;
createdAt_ = value;
onChanged();
return this;
}
/**
* required int64 created_at = 4;
*/
public Builder clearCreatedAt() {
bitField0_ = (bitField0_ & ~0x00000008);
createdAt_ = 0L;
onChanged();
return this;
}
private java.util.List property_ =
java.util.Collections.emptyList();
private void ensurePropertyIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
property_ = new java.util.ArrayList(property_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.smoketurner.notification.application.protos.NotificationProtos.Property, com.smoketurner.notification.application.protos.NotificationProtos.Property.Builder, com.smoketurner.notification.application.protos.NotificationProtos.PropertyOrBuilder> propertyBuilder_;
/**
* repeated .notification.Property property = 5;
*/
public java.util.List getPropertyList() {
if (propertyBuilder_ == null) {
return java.util.Collections.unmodifiableList(property_);
} else {
return propertyBuilder_.getMessageList();
}
}
/**
* repeated .notification.Property property = 5;
*/
public int getPropertyCount() {
if (propertyBuilder_ == null) {
return property_.size();
} else {
return propertyBuilder_.getCount();
}
}
/**
* repeated .notification.Property property = 5;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.Property getProperty(int index) {
if (propertyBuilder_ == null) {
return property_.get(index);
} else {
return propertyBuilder_.getMessage(index);
}
}
/**
* repeated .notification.Property property = 5;
*/
public Builder setProperty(
int index, com.smoketurner.notification.application.protos.NotificationProtos.Property value) {
if (propertyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePropertyIsMutable();
property_.set(index, value);
onChanged();
} else {
propertyBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .notification.Property property = 5;
*/
public Builder setProperty(
int index, com.smoketurner.notification.application.protos.NotificationProtos.Property.Builder builderForValue) {
if (propertyBuilder_ == null) {
ensurePropertyIsMutable();
property_.set(index, builderForValue.build());
onChanged();
} else {
propertyBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .notification.Property property = 5;
*/
public Builder addProperty(com.smoketurner.notification.application.protos.NotificationProtos.Property value) {
if (propertyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePropertyIsMutable();
property_.add(value);
onChanged();
} else {
propertyBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .notification.Property property = 5;
*/
public Builder addProperty(
int index, com.smoketurner.notification.application.protos.NotificationProtos.Property value) {
if (propertyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePropertyIsMutable();
property_.add(index, value);
onChanged();
} else {
propertyBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .notification.Property property = 5;
*/
public Builder addProperty(
com.smoketurner.notification.application.protos.NotificationProtos.Property.Builder builderForValue) {
if (propertyBuilder_ == null) {
ensurePropertyIsMutable();
property_.add(builderForValue.build());
onChanged();
} else {
propertyBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .notification.Property property = 5;
*/
public Builder addProperty(
int index, com.smoketurner.notification.application.protos.NotificationProtos.Property.Builder builderForValue) {
if (propertyBuilder_ == null) {
ensurePropertyIsMutable();
property_.add(index, builderForValue.build());
onChanged();
} else {
propertyBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .notification.Property property = 5;
*/
public Builder addAllProperty(
java.lang.Iterable extends com.smoketurner.notification.application.protos.NotificationProtos.Property> values) {
if (propertyBuilder_ == null) {
ensurePropertyIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, property_);
onChanged();
} else {
propertyBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .notification.Property property = 5;
*/
public Builder clearProperty() {
if (propertyBuilder_ == null) {
property_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
propertyBuilder_.clear();
}
return this;
}
/**
* repeated .notification.Property property = 5;
*/
public Builder removeProperty(int index) {
if (propertyBuilder_ == null) {
ensurePropertyIsMutable();
property_.remove(index);
onChanged();
} else {
propertyBuilder_.remove(index);
}
return this;
}
/**
* repeated .notification.Property property = 5;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.Property.Builder getPropertyBuilder(
int index) {
return getPropertyFieldBuilder().getBuilder(index);
}
/**
* repeated .notification.Property property = 5;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.PropertyOrBuilder getPropertyOrBuilder(
int index) {
if (propertyBuilder_ == null) {
return property_.get(index); } else {
return propertyBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .notification.Property property = 5;
*/
public java.util.List extends com.smoketurner.notification.application.protos.NotificationProtos.PropertyOrBuilder>
getPropertyOrBuilderList() {
if (propertyBuilder_ != null) {
return propertyBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(property_);
}
}
/**
* repeated .notification.Property property = 5;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.Property.Builder addPropertyBuilder() {
return getPropertyFieldBuilder().addBuilder(
com.smoketurner.notification.application.protos.NotificationProtos.Property.getDefaultInstance());
}
/**
* repeated .notification.Property property = 5;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.Property.Builder addPropertyBuilder(
int index) {
return getPropertyFieldBuilder().addBuilder(
index, com.smoketurner.notification.application.protos.NotificationProtos.Property.getDefaultInstance());
}
/**
* repeated .notification.Property property = 5;
*/
public java.util.List
getPropertyBuilderList() {
return getPropertyFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.smoketurner.notification.application.protos.NotificationProtos.Property, com.smoketurner.notification.application.protos.NotificationProtos.Property.Builder, com.smoketurner.notification.application.protos.NotificationProtos.PropertyOrBuilder>
getPropertyFieldBuilder() {
if (propertyBuilder_ == null) {
propertyBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.smoketurner.notification.application.protos.NotificationProtos.Property, com.smoketurner.notification.application.protos.NotificationProtos.Property.Builder, com.smoketurner.notification.application.protos.NotificationProtos.PropertyOrBuilder>(
property_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
property_ = null;
}
return propertyBuilder_;
}
// @@protoc_insertion_point(builder_scope:notification.NotificationPB)
}
static {
defaultInstance = new NotificationPB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:notification.NotificationPB)
}
public interface NotificationListPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:notification.NotificationListPB)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .notification.NotificationPB notification = 1;
*/
java.util.List
getNotificationList();
/**
* repeated .notification.NotificationPB notification = 1;
*/
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB getNotification(int index);
/**
* repeated .notification.NotificationPB notification = 1;
*/
int getNotificationCount();
/**
* repeated .notification.NotificationPB notification = 1;
*/
java.util.List extends com.smoketurner.notification.application.protos.NotificationProtos.NotificationPBOrBuilder>
getNotificationOrBuilderList();
/**
* repeated .notification.NotificationPB notification = 1;
*/
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPBOrBuilder getNotificationOrBuilder(
int index);
/**
* repeated int64 deleted_id = 2;
*/
java.util.List getDeletedIdList();
/**
* repeated int64 deleted_id = 2;
*/
int getDeletedIdCount();
/**
* repeated int64 deleted_id = 2;
*/
long getDeletedId(int index);
}
/**
* Protobuf type {@code notification.NotificationListPB}
*/
public static final class NotificationListPB extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:notification.NotificationListPB)
NotificationListPBOrBuilder {
// Use NotificationListPB.newBuilder() to construct.
private NotificationListPB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private NotificationListPB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final NotificationListPB defaultInstance;
public static NotificationListPB getDefaultInstance() {
return defaultInstance;
}
public NotificationListPB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NotificationListPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
notification_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
notification_.add(input.readMessage(com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.PARSER, extensionRegistry));
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
deletedId_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
deletedId_.add(input.readInt64());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002) && input.getBytesUntilLimit() > 0) {
deletedId_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
deletedId_.add(input.readInt64());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
notification_ = java.util.Collections.unmodifiableList(notification_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
deletedId_ = java.util.Collections.unmodifiableList(deletedId_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_NotificationListPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_NotificationListPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB.class, com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public NotificationListPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NotificationListPB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public static final int NOTIFICATION_FIELD_NUMBER = 1;
private java.util.List notification_;
/**
* repeated .notification.NotificationPB notification = 1;
*/
public java.util.List getNotificationList() {
return notification_;
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public java.util.List extends com.smoketurner.notification.application.protos.NotificationProtos.NotificationPBOrBuilder>
getNotificationOrBuilderList() {
return notification_;
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public int getNotificationCount() {
return notification_.size();
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB getNotification(int index) {
return notification_.get(index);
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationPBOrBuilder getNotificationOrBuilder(
int index) {
return notification_.get(index);
}
public static final int DELETED_ID_FIELD_NUMBER = 2;
private java.util.List deletedId_;
/**
* repeated int64 deleted_id = 2;
*/
public java.util.List
getDeletedIdList() {
return deletedId_;
}
/**
* repeated int64 deleted_id = 2;
*/
public int getDeletedIdCount() {
return deletedId_.size();
}
/**
* repeated int64 deleted_id = 2;
*/
public long getDeletedId(int index) {
return deletedId_.get(index);
}
private void initFields() {
notification_ = java.util.Collections.emptyList();
deletedId_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getNotificationCount(); i++) {
if (!getNotification(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < notification_.size(); i++) {
output.writeMessage(1, notification_.get(i));
}
for (int i = 0; i < deletedId_.size(); i++) {
output.writeInt64(2, deletedId_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < notification_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, notification_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < deletedId_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(deletedId_.get(i));
}
size += dataSize;
size += 1 * getDeletedIdList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code notification.NotificationListPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:notification.NotificationListPB)
com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_NotificationListPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_NotificationListPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB.class, com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB.Builder.class);
}
// Construct using com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getNotificationFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (notificationBuilder_ == null) {
notification_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
notificationBuilder_.clear();
}
deletedId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.smoketurner.notification.application.protos.NotificationProtos.internal_static_notification_NotificationListPB_descriptor;
}
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB getDefaultInstanceForType() {
return com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB.getDefaultInstance();
}
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB build() {
com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB buildPartial() {
com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB result = new com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB(this);
int from_bitField0_ = bitField0_;
if (notificationBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
notification_ = java.util.Collections.unmodifiableList(notification_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.notification_ = notification_;
} else {
result.notification_ = notificationBuilder_.build();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
deletedId_ = java.util.Collections.unmodifiableList(deletedId_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.deletedId_ = deletedId_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB) {
return mergeFrom((com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB other) {
if (other == com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB.getDefaultInstance()) return this;
if (notificationBuilder_ == null) {
if (!other.notification_.isEmpty()) {
if (notification_.isEmpty()) {
notification_ = other.notification_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureNotificationIsMutable();
notification_.addAll(other.notification_);
}
onChanged();
}
} else {
if (!other.notification_.isEmpty()) {
if (notificationBuilder_.isEmpty()) {
notificationBuilder_.dispose();
notificationBuilder_ = null;
notification_ = other.notification_;
bitField0_ = (bitField0_ & ~0x00000001);
notificationBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getNotificationFieldBuilder() : null;
} else {
notificationBuilder_.addAllMessages(other.notification_);
}
}
}
if (!other.deletedId_.isEmpty()) {
if (deletedId_.isEmpty()) {
deletedId_ = other.deletedId_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureDeletedIdIsMutable();
deletedId_.addAll(other.deletedId_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getNotificationCount(); i++) {
if (!getNotification(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.smoketurner.notification.application.protos.NotificationProtos.NotificationListPB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List notification_ =
java.util.Collections.emptyList();
private void ensureNotificationIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
notification_ = new java.util.ArrayList(notification_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.Builder, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPBOrBuilder> notificationBuilder_;
/**
* repeated .notification.NotificationPB notification = 1;
*/
public java.util.List getNotificationList() {
if (notificationBuilder_ == null) {
return java.util.Collections.unmodifiableList(notification_);
} else {
return notificationBuilder_.getMessageList();
}
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public int getNotificationCount() {
if (notificationBuilder_ == null) {
return notification_.size();
} else {
return notificationBuilder_.getCount();
}
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB getNotification(int index) {
if (notificationBuilder_ == null) {
return notification_.get(index);
} else {
return notificationBuilder_.getMessage(index);
}
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public Builder setNotification(
int index, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB value) {
if (notificationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNotificationIsMutable();
notification_.set(index, value);
onChanged();
} else {
notificationBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public Builder setNotification(
int index, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.Builder builderForValue) {
if (notificationBuilder_ == null) {
ensureNotificationIsMutable();
notification_.set(index, builderForValue.build());
onChanged();
} else {
notificationBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public Builder addNotification(com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB value) {
if (notificationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNotificationIsMutable();
notification_.add(value);
onChanged();
} else {
notificationBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public Builder addNotification(
int index, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB value) {
if (notificationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNotificationIsMutable();
notification_.add(index, value);
onChanged();
} else {
notificationBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public Builder addNotification(
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.Builder builderForValue) {
if (notificationBuilder_ == null) {
ensureNotificationIsMutable();
notification_.add(builderForValue.build());
onChanged();
} else {
notificationBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public Builder addNotification(
int index, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.Builder builderForValue) {
if (notificationBuilder_ == null) {
ensureNotificationIsMutable();
notification_.add(index, builderForValue.build());
onChanged();
} else {
notificationBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public Builder addAllNotification(
java.lang.Iterable extends com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB> values) {
if (notificationBuilder_ == null) {
ensureNotificationIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, notification_);
onChanged();
} else {
notificationBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public Builder clearNotification() {
if (notificationBuilder_ == null) {
notification_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
notificationBuilder_.clear();
}
return this;
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public Builder removeNotification(int index) {
if (notificationBuilder_ == null) {
ensureNotificationIsMutable();
notification_.remove(index);
onChanged();
} else {
notificationBuilder_.remove(index);
}
return this;
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.Builder getNotificationBuilder(
int index) {
return getNotificationFieldBuilder().getBuilder(index);
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationPBOrBuilder getNotificationOrBuilder(
int index) {
if (notificationBuilder_ == null) {
return notification_.get(index); } else {
return notificationBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public java.util.List extends com.smoketurner.notification.application.protos.NotificationProtos.NotificationPBOrBuilder>
getNotificationOrBuilderList() {
if (notificationBuilder_ != null) {
return notificationBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(notification_);
}
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.Builder addNotificationBuilder() {
return getNotificationFieldBuilder().addBuilder(
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.getDefaultInstance());
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.Builder addNotificationBuilder(
int index) {
return getNotificationFieldBuilder().addBuilder(
index, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.getDefaultInstance());
}
/**
* repeated .notification.NotificationPB notification = 1;
*/
public java.util.List
getNotificationBuilderList() {
return getNotificationFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.Builder, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPBOrBuilder>
getNotificationFieldBuilder() {
if (notificationBuilder_ == null) {
notificationBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPB.Builder, com.smoketurner.notification.application.protos.NotificationProtos.NotificationPBOrBuilder>(
notification_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
notification_ = null;
}
return notificationBuilder_;
}
private java.util.List deletedId_ = java.util.Collections.emptyList();
private void ensureDeletedIdIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
deletedId_ = new java.util.ArrayList(deletedId_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated int64 deleted_id = 2;
*/
public java.util.List
getDeletedIdList() {
return java.util.Collections.unmodifiableList(deletedId_);
}
/**
* repeated int64 deleted_id = 2;
*/
public int getDeletedIdCount() {
return deletedId_.size();
}
/**
* repeated int64 deleted_id = 2;
*/
public long getDeletedId(int index) {
return deletedId_.get(index);
}
/**
* repeated int64 deleted_id = 2;
*/
public Builder setDeletedId(
int index, long value) {
ensureDeletedIdIsMutable();
deletedId_.set(index, value);
onChanged();
return this;
}
/**
* repeated int64 deleted_id = 2;
*/
public Builder addDeletedId(long value) {
ensureDeletedIdIsMutable();
deletedId_.add(value);
onChanged();
return this;
}
/**
* repeated int64 deleted_id = 2;
*/
public Builder addAllDeletedId(
java.lang.Iterable extends java.lang.Long> values) {
ensureDeletedIdIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, deletedId_);
onChanged();
return this;
}
/**
* repeated int64 deleted_id = 2;
*/
public Builder clearDeletedId() {
deletedId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:notification.NotificationListPB)
}
static {
defaultInstance = new NotificationListPB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:notification.NotificationListPB)
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_notification_Property_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_notification_Property_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_notification_NotificationPB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_notification_NotificationPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_notification_NotificationListPB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_notification_NotificationListPB_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\022notification.proto\022\014notification\"&\n\010Pr" +
"operty\022\013\n\003key\030\001 \002(\t\022\r\n\005value\030\002 \002(\t\"}\n\016No" +
"tificationPB\022\n\n\002id\030\001 \002(\003\022\020\n\010category\030\002 \002" +
"(\t\022\017\n\007message\030\003 \002(\t\022\022\n\ncreated_at\030\004 \002(\003\022" +
"(\n\010property\030\005 \003(\0132\026.notification.Propert" +
"y\"\\\n\022NotificationListPB\0222\n\014notification\030" +
"\001 \003(\0132\034.notification.NotificationPB\022\022\n\nd" +
"eleted_id\030\002 \003(\003BG\n/com.smoketurner.notif" +
"ication.application.protosB\022Notification" +
"ProtosH\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_notification_Property_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_notification_Property_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_notification_Property_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_notification_NotificationPB_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_notification_NotificationPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_notification_NotificationPB_descriptor,
new java.lang.String[] { "Id", "Category", "Message", "CreatedAt", "Property", });
internal_static_notification_NotificationListPB_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_notification_NotificationListPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_notification_NotificationListPB_descriptor,
new java.lang.String[] { "Notification", "DeletedId", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy