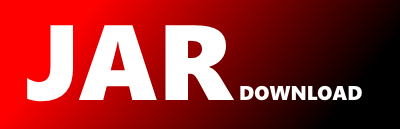
com.socketLabs.injectionApi.SendResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of injectionApi Show documentation
Show all versions of injectionApi Show documentation
SocketLabs Email Delivery Java library
package com.socketLabs.injectionApi;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
/**
* The response of an SocketLabsClient send request.
*/
public class SendResponse {
/**
* The result of the SocketLabsClient send request.
*/
@JsonProperty("ErrorCode")
private SendResult result;
/**
* The unique key generated by the Injection API if an unexpected error occurs during the SocketLabsClient send request.
* This unique key can be used by SocketLabs support to troubleshoot the issue.
*/
@JsonProperty("TransactionReceipt")
private String transactionReceipt;
/**
* An array of AddressResult objects that contain the status of each address that failed. If no messages failed this array is empty.
*/
@JsonProperty("MessageResults")
private List addressResults;
/**
* Initializes a new instance of the SendResponse class
*/
public SendResponse() {
}
/**
* Initializes a new instance of the SendResponse class
* @param result The result of the SocketLabsClient send request.
*/
public SendResponse(SendResult result) {
this.result = result;
this.transactionReceipt = null;
this.addressResults = null;
}
/**
* Initializes a new instance of the SendResponse class
* @param result The result of the SocketLabsClient send request.
* @param addressResults An array of AddressResult of the SocketLabsClient send request.
*/
public SendResponse(SendResult result, List addressResults) {
this.result = result;
this.transactionReceipt = null;
this.addressResults = addressResults;
}
/**
* Initializes a new instance of the SendResponse class
* @param result The result of the SocketLabsClient send request.
* @param transactionReceipt The unique key generated by the Injection API if an unexpected error occurs during the SocketLabsClient send request.
* @param addressResults An array of AddressResult objects that contain the status of each address that failed. If no messages failed this array is empty.
*/
public SendResponse(SendResult result, String transactionReceipt, List addressResults) {
this.result = result;
this.transactionReceipt = transactionReceipt;
this.addressResults = addressResults;
}
/**
* Get the result of the SocketLabsClient send request.
* @return SendResult
*/
public SendResult getResult() {
return result;
}
/**
* Set the result of the SocketLabsClient send request.
* @param value SendResult
*/
public void setResult(SendResult value) {
this.result = value;
}
/**
* Get the unique key generated by the Injection API if an unexpected error occurs during the SocketLabsClient send request.
* @return String
*/
public String getTransactionReceipt() {
return transactionReceipt;
}
/**
* Set the unique key generated by the Injection API if an unexpected error occurs during the SocketLabsClient send request.
* @param value String
*/
public void setTransactionReceipt(String value) {
this.transactionReceipt = value;
}
/**
* Get the array of AddressResult objects that contain the status of each address that failed. If no messages failed this array is empty.
* @return List of AddressResult objects
*/
public List getAddressResults() {
return addressResults;
}
/**
* Set the array of AddressResult objects that contain the status of each address that failed. If no messages failed this array is empty.
* @param value {@code List}
*/
public void setAddressResults(List value) {
this.addressResults = value;
}
/**
* A message detailing why the SocketLabsClient send request failed.
* @return String
*/
public String getResponseMessage() {
switch (this.result) {
case UnknownError:
return "An error has occured that was unforeseen";
case Timeout:
return "A timeout occurred sending the message";
case Success:
return "Successful send of message";
case Warning:
return "Warnings were found while sending the message";
case InternalError:
return "Internal server error";
case MessageTooLarge:
return "Message size has exceeded the size limit";
case TooManyRecipients:
return "Message exceeded maximum recipient count in the message";
case InvalidData:
return "Invalid data was found on the message";
case OverQuota:
return "The account is over the send quota, rate limit exceeded";
case TooManyErrors:
return "Too many errors occurred sending the message";
case InvalidAuthentication:
return "The ServerId/ApiKey combination is invalid";
case AccountDisabled:
return "The account has been disabled";
case TooManyMessages:
return "Too many messages were found in the request";
case NoValidRecipients:
return "No valid recipients were found in the message";
case InvalidAddress:
return "An invalid recipient were found on the message";
case InvalidAttachment:
return "An invalid attachment were found on the message";
case NoMessages:
return "No message body was found in the message";
case EmptyMessage:
return "No message body was found in the message";
case EmptySubject:
return "No subject was found in the message";
case InvalidFrom:
return "An invalid from address was found on the message";
case EmptyToAddress:
return "No To addresses were found in the message";
case NoValidBodyParts:
return "No valid message body was found in the message";
case InvalidTemplateId:
return "An invalid TemplateId was found in the message";
case TemplateHasNoContent:
return "The specified TemplateId has no content for the message";
case MessageBodyConflict:
return "A conflict occurred on the message body of the message";
case InvalidMergeData:
return "Invalid MergeData was found on the message";
case AuthenticationValidationFailed:
return "SDK Validation Error : Authentication Validation Failed, Missing or invalid ServerId or ApiKey";
case RecipientValidationMaxExceeded:
return "SDK Validation Error : Message exceeded maximum recipient count in the message";
case RecipientValidationNoneInMessage:
return "SDK Validation Error : No Recipients were found in the message";
case EmailAddressValidationMissingFrom:
return "SDK Validation Error : From email address is missing in the message";
case RecipientValidationMissingTo:
return "SDK Validation Error : To addresses are missing in the message";
case EmailAddressValidationInvalidFrom:
return "SDK Validation Error : From email address in the message is invalid";
case MessageValidationEmptySubject:
return "SDK Validation Error : No Subject was found in the message";
case MessageValidationEmptyMessage:
return "SDK Validation Error : No message body was found in the message";
case MessageValidationInvalidCustomHeaders:
return "SDK Validation Error : Invalid Custom Headers were found in the message";
case RecipientValidationInvalidReplyTo:
return "SDK Validation Error : Invalid ReplyTo Address was found in the message";
case RecipientValidationInvalidRecipients:
return "SDK Validation Error : Invalid recipients were found in the message";
default:
return "";
}
}
/**
* Represents the SendResponse class as a string. Useful for debugging.
* @return String
*/
@Override
public String toString() {
return String.format("%s: %s", this.result.toString(), this.getResponseMessage());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy