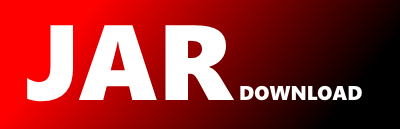
com.softlayer.api.service.network.message.Queue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of softlayer-api-client Show documentation
Show all versions of softlayer-api-client Show documentation
API client for accessing the SoftLayer API
package com.softlayer.api.service.network.message;
import com.softlayer.api.ApiClient;
import com.softlayer.api.ResponseHandler;
import com.softlayer.api.annotation.ApiMethod;
import com.softlayer.api.annotation.ApiProperty;
import com.softlayer.api.annotation.ApiType;
import com.softlayer.api.service.Account;
import com.softlayer.api.service.Entity;
import com.softlayer.api.service.billing.Item;
import com.softlayer.api.service.network.message.queue.Node;
import com.softlayer.api.service.network.message.queue.Status;
import java.util.ArrayList;
import java.util.GregorianCalendar;
import java.util.List;
import java.util.concurrent.Future;
/**
* The SoftLayer_Network_Message_Queue data type contains general information relating to Message Queue account
*
* @see SoftLayer_Network_Message_Queue
*/
@ApiType("SoftLayer_Network_Message_Queue")
public class Queue extends Entity {
/**
* The account that a message queue belongs to.
*/
@ApiProperty
protected Account account;
public Account getAccount() {
return account;
}
public void setAccount(Account account) {
this.account = account;
}
/**
* The current billing item for this message queue account.
*/
@ApiProperty
protected Item billingItem;
public Item getBillingItem() {
return billingItem;
}
public void setBillingItem(Item billingItem) {
this.billingItem = billingItem;
}
/**
* All available message queue nodes
*/
@ApiProperty
protected List nodes;
public List getNodes() {
if (nodes == null) {
nodes = new ArrayList();
}
return nodes;
}
/**
* A message queue account status.
*/
@ApiProperty
protected Status status;
public Status getStatus() {
return status;
}
public void setStatus(Status status) {
this.status = status;
}
/**
* A message queue's associated [[SoftLayer_Account|account]] id.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long accountId;
public Long getAccountId() {
return accountId;
}
public void setAccountId(Long accountId) {
accountIdSpecified = true;
this.accountId = accountId;
}
protected boolean accountIdSpecified;
public boolean isAccountIdSpecified() {
return accountIdSpecified;
}
public void unsetAccountId() {
accountId = null;
accountIdSpecified = false;
}
/**
* The date that a message queue account was created.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected GregorianCalendar createDate;
public GregorianCalendar getCreateDate() {
return createDate;
}
public void setCreateDate(GregorianCalendar createDate) {
createDateSpecified = true;
this.createDate = createDate;
}
protected boolean createDateSpecified;
public boolean isCreateDateSpecified() {
return createDateSpecified;
}
public void unsetCreateDate() {
createDate = null;
createDateSpecified = false;
}
/**
* A message queue's internal identification number
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long id;
public Long getId() {
return id;
}
public void setId(Long id) {
idSpecified = true;
this.id = id;
}
protected boolean idSpecified;
public boolean isIdSpecified() {
return idSpecified;
}
public void unsetId() {
id = null;
idSpecified = false;
}
/**
* A message queue status' internal identifier.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long messageQueueStatusId;
public Long getMessageQueueStatusId() {
return messageQueueStatusId;
}
public void setMessageQueueStatusId(Long messageQueueStatusId) {
messageQueueStatusIdSpecified = true;
this.messageQueueStatusId = messageQueueStatusId;
}
protected boolean messageQueueStatusIdSpecified;
public boolean isMessageQueueStatusIdSpecified() {
return messageQueueStatusIdSpecified;
}
public void unsetMessageQueueStatusId() {
messageQueueStatusId = null;
messageQueueStatusIdSpecified = false;
}
/**
* A unique message queue account name
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String name;
public String getName() {
return name;
}
public void setName(String name) {
nameSpecified = true;
this.name = name;
}
protected boolean nameSpecified;
public boolean isNameSpecified() {
return nameSpecified;
}
public void unsetName() {
name = null;
nameSpecified = false;
}
/**
* Brief notes on this message queue account
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String notes;
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
notesSpecified = true;
this.notes = notes;
}
protected boolean notesSpecified;
public boolean isNotesSpecified() {
return notesSpecified;
}
public void unsetNotes() {
notes = null;
notesSpecified = false;
}
/**
* A count of all available message queue nodes
*/
@ApiProperty
protected Long nodeCount;
public Long getNodeCount() {
return nodeCount;
}
public void setNodeCount(Long nodeCount) {
this.nodeCount = nodeCount;
}
public Service asService(ApiClient client) {
return service(client, id);
}
public static Service service(ApiClient client) {
return client.createService(Service.class, null);
}
public static Service service(ApiClient client, Long id) {
return client.createService(Service.class, id == null ? null : id.toString());
}
/**
* Message Queue provides a scalable, performant, and redundant messaging and notification system that provides asynchronous communication for distributed applications. Developers can use the our Message Queue as the messaging and notification component in their architecture, providing unlimited interactions with high performance and reliable delivery. Our Message Queue offers no limit to the number of messages sent or received, number of queues created, number of notifications created, number of subscriptions, and number of notifications sent - the ultimate in scale.
*
* SoftLayer_Network_Message_Queue can be considered as a message queue account which includes multiple message queue nodes in different data centers.
*
* @see SoftLayer_Network_Message_Queue
*/
@com.softlayer.api.annotation.ApiService("SoftLayer_Network_Message_Queue")
public static interface Service extends com.softlayer.api.Service {
public ServiceAsync asAsync();
public Mask withNewMask();
public Mask withMask();
public void setMask(Mask mask);
/**
* @see SoftLayer_Network_Message_Queue::getObject
*/
@ApiMethod(instanceRequired = true)
public Queue getObject();
/**
* The account that a message queue belongs to.
*
* @see SoftLayer_Network_Message_Queue::getAccount
*/
@ApiMethod(instanceRequired = true)
public Account getAccount();
/**
* The current billing item for this message queue account.
*
* @see SoftLayer_Network_Message_Queue::getBillingItem
*/
@ApiMethod(instanceRequired = true)
public Item getBillingItem();
/**
* All available message queue nodes
*
* @see SoftLayer_Network_Message_Queue::getNodes
*/
@ApiMethod(instanceRequired = true)
public List getNodes();
/**
* A message queue account status.
*
* @see SoftLayer_Network_Message_Queue::getStatus
*/
@ApiMethod(instanceRequired = true)
public Status getStatus();
}
public static interface ServiceAsync extends com.softlayer.api.ServiceAsync {
public Mask withNewMask();
public Mask withMask();
public void setMask(Mask mask);
/**
* Async version of {@link Service#getObject}
*/
public Future getObject();
public Future> getObject(ResponseHandler callback);
/**
* Async version of {@link Service#getAccount}
*/
public Future getAccount();
/**
* Async callback version of {@link Service#getAccount}
*/
public Future> getAccount(ResponseHandler callback);
/**
* Async version of {@link Service#getBillingItem}
*/
public Future- getBillingItem();
/**
* Async callback version of {@link Service#getBillingItem}
*/
public Future> getBillingItem(ResponseHandler
- callback);
/**
* Async version of {@link Service#getNodes}
*/
public Future
> getNodes();
/**
* Async callback version of {@link Service#getNodes}
*/
public Future> getNodes(ResponseHandler> callback);
/**
* Async version of {@link Service#getStatus}
*/
public Future getStatus();
/**
* Async callback version of {@link Service#getStatus}
*/
public Future> getStatus(ResponseHandler callback);
}
public static class Mask extends com.softlayer.api.service.Entity.Mask {
public com.softlayer.api.service.Account.Mask account() {
return withSubMask("account", com.softlayer.api.service.Account.Mask.class);
}
public com.softlayer.api.service.billing.Item.Mask billingItem() {
return withSubMask("billingItem", com.softlayer.api.service.billing.Item.Mask.class);
}
public com.softlayer.api.service.network.message.queue.Node.Mask nodes() {
return withSubMask("nodes", com.softlayer.api.service.network.message.queue.Node.Mask.class);
}
public com.softlayer.api.service.network.message.queue.Status.Mask status() {
return withSubMask("status", com.softlayer.api.service.network.message.queue.Status.Mask.class);
}
public Mask accountId() {
withLocalProperty("accountId");
return this;
}
public Mask createDate() {
withLocalProperty("createDate");
return this;
}
public Mask id() {
withLocalProperty("id");
return this;
}
public Mask messageQueueStatusId() {
withLocalProperty("messageQueueStatusId");
return this;
}
public Mask name() {
withLocalProperty("name");
return this;
}
public Mask notes() {
withLocalProperty("notes");
return this;
}
public Mask nodeCount() {
withLocalProperty("nodeCount");
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy