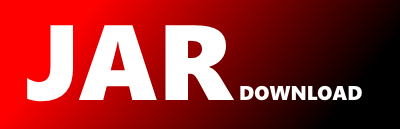
com.softlayer.api.service.network.message.queue.Node Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of softlayer-api-client Show documentation
Show all versions of softlayer-api-client Show documentation
API client for accessing the SoftLayer API
package com.softlayer.api.service.network.message.queue;
import com.softlayer.api.ApiClient;
import com.softlayer.api.ResponseHandler;
import com.softlayer.api.annotation.ApiMethod;
import com.softlayer.api.annotation.ApiProperty;
import com.softlayer.api.annotation.ApiType;
import com.softlayer.api.service.Entity;
import com.softlayer.api.service.container.Graph;
import com.softlayer.api.service.metric.tracking.Object;
import com.softlayer.api.service.metric.tracking.object.Data;
import com.softlayer.api.service.network.message.Queue;
import com.softlayer.api.service.network.service.Resource;
import java.util.GregorianCalendar;
import java.util.List;
import java.util.concurrent.Future;
/**
* The SoftLayer_Network_Message_Queue_Node data type contains general information relating to Message Queue node
*
* @see SoftLayer_Network_Message_Queue_Node
*/
@ApiType("SoftLayer_Network_Message_Queue_Node")
public class Node extends Entity {
/**
* The message queue account this node belongs to.
*/
@ApiProperty
protected Queue messageQueue;
public Queue getMessageQueue() {
return messageQueue;
}
public void setMessageQueue(Queue messageQueue) {
this.messageQueue = messageQueue;
}
/**
* A message queue node's metric tracking object. This object records all request and notification count data for this message queue node.
*/
@ApiProperty
protected Object metricTrackingObject;
public Object getMetricTrackingObject() {
return metricTrackingObject;
}
public void setMetricTrackingObject(Object metricTrackingObject) {
this.metricTrackingObject = metricTrackingObject;
}
@ApiProperty
protected Resource serviceResource;
public Resource getServiceResource() {
return serviceResource;
}
public void setServiceResource(Resource serviceResource) {
this.serviceResource = serviceResource;
}
/**
* A unique account name in this message queue node
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String accountName;
public String getAccountName() {
return accountName;
}
public void setAccountName(String accountName) {
accountNameSpecified = true;
this.accountName = accountName;
}
protected boolean accountNameSpecified;
public boolean isAccountNameSpecified() {
return accountNameSpecified;
}
public void unsetAccountName() {
accountName = null;
accountNameSpecified = false;
}
/**
* A message queue node's internal identification number
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long id;
public Long getId() {
return id;
}
public void setId(Long id) {
idSpecified = true;
this.id = id;
}
protected boolean idSpecified;
public boolean isIdSpecified() {
return idSpecified;
}
public void unsetId() {
id = null;
idSpecified = false;
}
/**
* A message queue node's associated message queue id.
*/
@ApiProperty(canBeNullOrNotSet = true)
protected Long messageQueueId;
public Long getMessageQueueId() {
return messageQueueId;
}
public void setMessageQueueId(Long messageQueueId) {
messageQueueIdSpecified = true;
this.messageQueueId = messageQueueId;
}
protected boolean messageQueueIdSpecified;
public boolean isMessageQueueIdSpecified() {
return messageQueueIdSpecified;
}
public void unsetMessageQueueId() {
messageQueueId = null;
messageQueueIdSpecified = false;
}
/**
* A user-friendly name of this message queue node
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String name;
public String getName() {
return name;
}
public void setName(String name) {
nameSpecified = true;
this.name = name;
}
protected boolean nameSpecified;
public boolean isNameSpecified() {
return nameSpecified;
}
public void unsetName() {
name = null;
nameSpecified = false;
}
/**
* Brief notes on this message queue node
*/
@ApiProperty(canBeNullOrNotSet = true)
protected String notes;
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
notesSpecified = true;
this.notes = notes;
}
protected boolean notesSpecified;
public boolean isNotesSpecified() {
return notesSpecified;
}
public void unsetNotes() {
notes = null;
notesSpecified = false;
}
public Service asService(ApiClient client) {
return service(client, id);
}
public static Service service(ApiClient client) {
return client.createService(Service.class, null);
}
public static Service service(ApiClient client, Long id) {
return client.createService(Service.class, id == null ? null : id.toString());
}
/**
* Message queue account has multiple nodes in different data center. This object represents a message queue node.
*
* @see SoftLayer_Network_Message_Queue_Node
*/
@com.softlayer.api.annotation.ApiService("SoftLayer_Network_Message_Queue_Node")
public static interface Service extends com.softlayer.api.Service {
public ServiceAsync asAsync();
public Mask withNewMask();
public Mask withMask();
public void setMask(Mask mask);
/**
* @see SoftLayer_Network_Message_Queue_Node::addUser
*/
@ApiMethod(instanceRequired = true)
public Boolean addUser(String username);
/**
* @see SoftLayer_Network_Message_Queue_Node::deleteUser
*/
@ApiMethod(instanceRequired = true)
public Boolean deleteUser(String username);
/**
* @see SoftLayer_Network_Message_Queue_Node::getAllUsers
*/
@ApiMethod(instanceRequired = true)
public List getAllUsers();
/**
* @see SoftLayer_Network_Message_Queue_Node::getObject
*/
@ApiMethod(instanceRequired = true)
public Node getObject();
/**
* Retrieve usage graph by date.
*
* @see SoftLayer_Network_Message_Queue_Node::getUsage
*/
@ApiMethod(instanceRequired = true)
public List getUsage(GregorianCalendar startDate, GregorianCalendar endDate);
/**
* Retrieve usage graph by date.
*
* @see SoftLayer_Network_Message_Queue_Node::getUsageGraph
*/
@ApiMethod(instanceRequired = true)
public Graph getUsageGraph(Graph graphData);
/**
* The message queue account this node belongs to.
*
* @see SoftLayer_Network_Message_Queue_Node::getMessageQueue
*/
@ApiMethod(instanceRequired = true)
public Queue getMessageQueue();
/**
* A message queue node's metric tracking object. This object records all request and notification count data for this message queue node.
*
* @see SoftLayer_Network_Message_Queue_Node::getMetricTrackingObject
*/
@ApiMethod(instanceRequired = true)
public Object getMetricTrackingObject();
/**
* @see SoftLayer_Network_Message_Queue_Node::getServiceResource
*/
@ApiMethod(instanceRequired = true)
public Resource getServiceResource();
}
public static interface ServiceAsync extends com.softlayer.api.ServiceAsync {
public Mask withNewMask();
public Mask withMask();
public void setMask(Mask mask);
/**
* Async version of {@link Service#addUser}
*/
public Future addUser(String username);
public Future> addUser(String username, ResponseHandler callback);
/**
* Async version of {@link Service#deleteUser}
*/
public Future deleteUser(String username);
public Future> deleteUser(String username, ResponseHandler callback);
/**
* Async version of {@link Service#getAllUsers}
*/
public Future> getAllUsers();
public Future> getAllUsers(ResponseHandler> callback);
/**
* Async version of {@link Service#getObject}
*/
public Future getObject();
public Future> getObject(ResponseHandler callback);
/**
* Async version of {@link Service#getUsage}
*/
public Future> getUsage(GregorianCalendar startDate, GregorianCalendar endDate);
public Future> getUsage(GregorianCalendar startDate, GregorianCalendar endDate, ResponseHandler> callback);
/**
* Async version of {@link Service#getUsageGraph}
*/
public Future getUsageGraph(Graph graphData);
public Future> getUsageGraph(Graph graphData, ResponseHandler callback);
/**
* Async version of {@link Service#getMessageQueue}
*/
public Future getMessageQueue();
/**
* Async callback version of {@link Service#getMessageQueue}
*/
public Future> getMessageQueue(ResponseHandler callback);
/**
* Async version of {@link Service#getMetricTrackingObject}
*/
public Future
© 2015 - 2025 Weber Informatics LLC | Privacy Policy